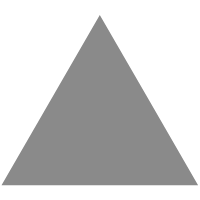
0
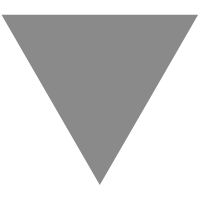
Javascripts中if的优化
source link: https://bajie.dev/posts/20220615-javascripts_if/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Javascripts中if的优化
2022-06-15
2 分钟阅读
Javascripts 中的 if … else 用起来倒是很方便,但是看起来就不舒服了
if (true) {
}
if (true) {
}
if (true) {
if (true) {
if (true) {
}
} else {
}
} else {
}
fallback
上面看起来很闹心吧,下面讲讲优化:
一、三元优化
if (ture) else可以用三元操作符来优化它:
// Bad 😥
if (true) {
console.log("Congratutions!")
} else {
console.warn("Oops, something went wrong!")
}
// Great 🥰
true
? console.log("Congratutions")
: console.warn("Oops, something went wrong!")
javascript
二、与优化
true的情况下才执行可以用与来进行优化:
if (true) {
alert(1)
}
// is equals to:
true && alert(1)
javascript
三、提前返回
如果有多个判断,按照复杂程度,从上到下,提前返回。
function handleRequest(req) {
if (req.code >= 500) {
return "Server error";
}
if (req.code >= 404) {
return "Cilent error";
}
return "Suceess";
}
javascript
四、类表格优化
下面是类似表格似的选择,通常会用 switch 来进行优化
// Bad 😥
const weekday = (num) => {
if (num === 1) {
return "Monday"
} else if (num === 2) {
return "Tuesday"
} else if (num === 3) {
return "Wednesday"
} else if (num === 4) {
return "Thursday"
} else if (num === 5) {
return "Friday"
} else if (num === 6) {
return "Saturday"
} else if (num === 7) {
return "Sunday"
} else {
return "Unknown"
}
}
console.log(weekday(1)) // Monday
fallback
switch 也不是最优解,用对象的键值对来解:
const weekday = (option) => {
let obj = {
1: "Monday",
2: "Tuesday",
3: "Wednesday",
4: "Thursday",
5: "Friday",
6: "Saturday",
0: "Sunday"
}
return obj[option] ?? "Unknown"
}
console.log(weekday(1)) // Monday
javascript
也可以用 ES6 的 map 来实现:
// Great 🥰
const weekday = (num) => {
const map = new Map()
.set(1, "Monday")
.set(2, "Tuesday")
.set(3, "Wednesday")
.set(4, "Thursday")
.set(5, "Friday")
.set(6, "Saturday")
.set(7, "Sunday");
return map.has(num) ? map.get(num) : "Unknown";
};
console.log(weekday(1));
javascript
五、选项多对一的数组优化
看如下代码,多个选项对应一个返回:
const getContinent = (option) => {
if (option === "China" || option === "Japan") {
return "Asia";
}
if (option === "Germany" || option === "France") {
return "Europe";
}
};
console.log(getContinent("China"));
javascript
使用数组来容纳多个选项,然后用 includes 来判断并返回
const getContinent = (option) => {
const Asia = ["China", "Japan"];
const Europe = ["Germany", "Franch"];
if (Asia.includes(option)) return "Asia";
if (Europe.includes(option)) return "Europe";
return "Unknown";
};
console.log(getContinent("China")); // Asia
javascript
进一步优化,用 && 与优化
const getContinent = (option) => {
let [result, setResult] = ["unknown", (str) => (result = str)];
const Asia = ["China", "Japan"];
const Europe = ["Germany", "Franch"];
Asia.includes(option) && setResult("Asia");
Europe.includes(option) && setResult("Europe");
return result;
};
console.log(getContinent("China"));
fallback
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK