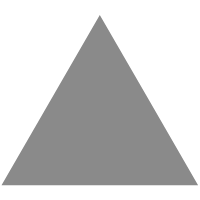
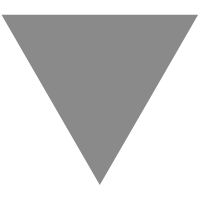
Go实战--golang中操作PDF(rsc.io/pdf、jung-kurt/gofpdf、signintech/gopdf)
source link: https://studygolang.com/articles/11398?fr=sidebar
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Go实战--golang中操作PDF(rsc.io/pdf、jung-kurt/gofpdf、signintech/gopdf)
wangshubo1989 · 2017-10-18 15:01:21 · 10898 次点击 · 预计阅读时间 5 分钟 · 大约8小时之前 开始浏览生命不止,继续 go go go !!!
昨天介绍了golang中如何操作excel:
Go实战–golang中操作excel(tealeg/xlsx、360EntSecGroup-Skylar/excelize)
那么今天就跟大家分享一下,golang中如何操作PDF。
PDF简介
The Portable Document Format (PDF) is a file format used to present documents in a manner independent of application software, hardware, and operating systems.[3] Each PDF file encapsulates a complete description of a fixed-layout flat document, including the text, fonts, graphics, and other information needed to display it.
pdf(Portable Document Format的简称,意为“便携式文档格式”),是由Adobe Systems用于与应用程序、操作系统、硬件无关的方式进行文件交换所发展出的文件格式。PDF文件以PostScript语言图象模型为基础,无论在哪种打印机上都可保证精确的颜色和准确的打印效果,即PDF会忠实地再现原稿的每一个字符、颜色以及图象。
rsc.io/pdf
github地址:
https://github.com/rsc/pdf
Star: 202
文档地址:
https://godoc.org/rsc.io/pdf
go get rsc.io/pdf
读取PDF文件,获取总页数
package main
import (
"fmt"
"rsc.io/pdf"
)
func main() {
file, err := pdf.Open("go.pdf")
if err != nil {
panic(err)
}
fmt.Println(file.NumPage())
}
读取某一页的内容
package main
import (
"fmt"
"rsc.io/pdf"
)
func main() {
file, err := pdf.Open("test.pdf")
if err != nil {
panic(err)
}
fmt.Println(file.Page(2).Content())
}
jung-kurt/gofpdf
注意:该库不支持中文!!!
github地址:
https://github.com/jung-kurt/gofpdf
Star: 733
文档地址:
https://godoc.org/github.com/jung-kurt/gofpdf
go get github.com/jung-kurt/gofpdf
生成PDF文档
package main
import (
"fmt"
"github.com/jung-kurt/gofpdf"
)
func main() {
pdf := gofpdf.New("P", "mm", "A4", "")
pdf.AddPage()
pdf.SetFont("Arial", "B", 26)
pdf.Cell(40, 10, "Hello PDF World")
err := pdf.OutputFileAndClose("write_pdf.pdf")
if err != nil {
fmt.Println(err)
}
}
生成加密的PDF
package main
import (
"fmt"
"github.com/jung-kurt/gofpdf"
)
func main() {
pdf := gofpdf.New("P", "mm", "A4", "")
pdf.SetProtection(gofpdf.CnProtectPrint, "123", "abc")
pdf.AddPage()
pdf.SetFont("Arial", "", 12)
pdf.Write(10, "You Must Enter the Password!!!")
err := pdf.OutputFileAndClose("write_pdf_with_password.pdf")
if err != nil {
fmt.Println(err)
}
}
PDF中插入图片
package main
import (
"fmt"
"github.com/jung-kurt/gofpdf"
)
func main() {
pdf := gofpdf.New("P", "mm", "A4", "")
pdf.AddPage()
pdf.SetFont("Arial", "", 11)
pdf.Image("test.png", 10, 10, 30, 0, false, "", 0, "")
pdf.Text(50, 20, "test.png")
pdf.Image("test.gif", 10, 40, 30, 0, false, "", 0, "")
pdf.Text(50, 50, "test.gif")
pdf.Image("test.jpg", 10, 130, 30, 0, false, "", 0, "")
pdf.Text(50, 140, "test.jpg")
err := pdf.OutputFileAndClose("write_pdf_with_image.pdf")
if err != nil {
fmt.Println(err)
}
}
PDF中增加链接、HTML
package main
import (
"fmt"
"github.com/jung-kurt/gofpdf"
)
func main() {
pdf := gofpdf.New("P", "mm", "A4", "")
pdf.AddPage()
pdf.SetFont("Helvetica", "", 20)
_, lineHt := pdf.GetFontSize()
pdf.Write(lineHt, "To find out what's new in this tutorial, click ")
pdf.SetFont("", "U", 0)
link := pdf.AddLink()
pdf.WriteLinkID(lineHt, "here", link)
pdf.SetFont("", "", 0)
// Second page: image link and basic HTML with link
pdf.AddPage()
pdf.SetLink(link, 0, -1)
pdf.Image("test.png", 10, 12, 30, 0, false, "", 0, "http://blog.csdn.net/wangshubo1989?viewmode=contents")
pdf.SetLeftMargin(45)
pdf.SetFontSize(14)
_, lineHt = pdf.GetFontSize()
htmlStr := `You can now easily print text mixing different styles: <b>bold</b>, ` +
`<i>italic</i>, <u>underlined</u>, or <b><i><u>all at once</u></i></b>!<br><br>` +
`<center>You can also center text.</center>` +
`<right>Or align it to the right.</right>` +
`You can also insert links on text, such as ` +
`<a href="http://www.fpdf.org">http://blog.csdn.net/wangshubo1989?viewmode=contents</a>, or on an image: click on the logo.`
html := pdf.HTMLBasicNew()
html.Write(lineHt, htmlStr)
err := pdf.OutputFileAndClose("write_html.pdf")
if err != nil {
fmt.Println(err)
}
}
signintech/gopdf
既然jung-kurt/gofpdf不支持中文,那么就介绍一个支持中文的signintech/gopdf。
github地址:
https://github.com/signintech/gopdf
Star: 422
go get -u github.com/signintech/gopdf
生成PDF文件
为了炫酷一点,自己从网上下载一个字体。
package main
import (
"log"
"github.com/signintech/gopdf"
)
func main() {
pdf := gopdf.GoPdf{}
pdf.Start(gopdf.Config{PageSize: gopdf.Rect{W: 595.28, H: 841.89}}) //595.28, 841.89 = A4
pdf.AddPage()
err := pdf.AddTTFFont("wts11", "TTENuoJ_0.ttf")
if err != nil {
log.Print(err.Error())
return
}
err = pdf.SetFont("wts11", "", 14)
if err != nil {
log.Print(err.Error())
return
}
pdf.Cell(nil, "我闭目在经殿的香雾中, 蓦然听见你颂经中的真言;")
pdf.WritePdf("hello.pdf")
}
有疑问加站长微信联系(非本文作者)

入群交流(和以上内容无关):加入Go大咖交流群,或添加微信:liuxiaoyan-s 备注:入群;或加QQ群:692541889
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK