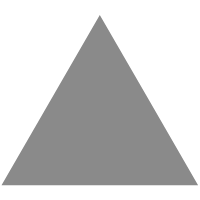
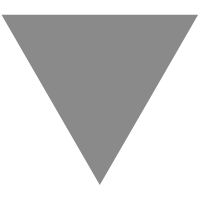
Ternary Operator ? : in C#
source link: https://code-maze.com/csharp-ternary-operator/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Ternary Operator ? : in C#
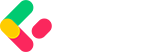
We value your privacy
Want to build great APIs? Or become even better at creating full stack applications? Check our Products Page and find our Books and Video Courses that we create using only the latest .NET technologies. Bonus materials included!
In this article, we are going to learn about the ternary operator (?:) in C#.
Let’s start.
What is a Ternary Operator?
Ternary Operator
is a conditional operator in C#. It helps us to define and execute our statements based on conditions, so basically, it is an alternative form of the if-else statement.
Syntax of C# Ternary Operator
The ternary operator
always work with 3 operands:
condition_expression ? statement_1 : statement_2
condition_expression
has two cases, if condition_expression
is true
then statement_1
is a return value. However, if condition_expression
is false
, the operator returns statement_2
. Condition is always a Boolean
expression.
This operator can replace the if-else statement in some situations.
Let’s see how:
var x = 5; var y = 10; if (x > y) { Console.WriteLine("x is greater than y"); } else { Console.WriteLine("y is greater than x"); } var result = (x > y) ? "x is greater than y" : "y is greater than x"; Console.WriteLine(result);
Here, we see that the ternary operator replaces the multi-line if-else statement with just a single line of code.
Note: It always returns a value as a result of comparisons.
Nested Ternary Operator
We can use a nested ternary operator
by using more than one condition:
var a = 10; var b = 10; if (a > b) { Console.WriteLine("a is greater than b"); } else if (a < b) { Console.WriteLine("b is greater than a"); } else { Console.WriteLine("a is equal to b"); } var comparison = (a > b) ? "a is greater than b" : (a < b) ? "b is greater than a" : "a is equal to b"; Console.WriteLine(comparison);
From our example, we can see that we can check multiple conditions with a single operator.
The conditional operator is right-associative. It means that the operator evaluates a ? b : c ? d : e
expression as a ? b : (c ? d : e)
, not as (a ? b : c) ? d: e
.
Conditional ref Expression
From the C# version 7.2, we are able to use the ref
keyword to assign the local ref variable conditionally with a conditional ref expression.
Let’s check the example without the ref
keyword:
var array1 = new int[] { 1, 2, 3, 4, 5 }; var number1 = 100; var value1 = number1 >= 100 ? array1[2] : array1[4]; value1 = 0; Console.WriteLine(string.Join(" ", array1));
In this case value1
does not point to array1
. So, when the value of value1
changes to "0"
, array1
stays unchanged.
But now, let’s see what happens when we use the ref
keyword in the same example:
var array2 = new int[] { 1, 2, 3, 4, 5 }; var number2 = 100; ref var value2 = ref ((number2 >= 100) ? ref array2[2] : ref array2[4]); value2 = 10000; Console.WriteLine(string.Join(" ", array2));
Here the value2
variable points to the third element (index 2) of array2
. This means when the value of value2
changes to 10000
, it also changes the array’s element on index 2.
Conclusion
In the article, we have learned about the ternary conditional operator. We’ve also seen how it works compared to the if-else statement.
Want to build great APIs? Or become even better at creating full stack applications? Check our Products Page and find our Books and Video Courses that we create using only the latest .NET technologies. Bonus materials included!
Share:
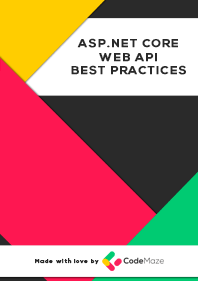
--- FREE eBook ---
Top 16 BEST PRACTICES
to improve API effectiveness 10x.
Find out how!
© Copyright code-maze.com 2016 - 2022
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK