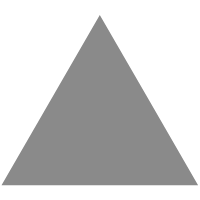
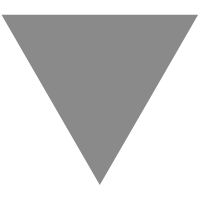
Paypal Card Payment REST API integration in Laravel 6
source link: https://www.laravelcode.com/post/paypal-card-payment-rest-api-integration-in-laravel-6
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Paypal Card Payment REST API integration in Laravel 6
In this article we will share with you how to integrate paypal card payment in your laravel application. in many time you need to integrate online card payment system in your laravel project. so, this article will be help for you. right now amny payment solution is available like stripe, paypal, SecurePay, 2Checkout, Authorize.net etc..
Paypal is very simple and easy payment gateway integration and it have large document, library and packages provide on online so you can get easiest any soslution on regarding on your error or any extra help.
Before you never integrate paypal card API in you laravel application then don't worry we are here show youu all step by step and very easy way.
Step - 1 Create paypal sandbox account
Very first step here you have one paypal sandbox account for before going live integration you can test and check all paypal card integration working fine there. simple go to this link and signup/signin your paypal sandbox account Paypal Developer Dashboard.
After singup/singin in your paypal sandbox account then click on the Accounts
menu which seen in left side. and then create one test bussined account and one buyer account. please seen following image for more information.
[ADDCODE]
After create account then click on View/Edit Account
link which you can seen under Manage Accounts
. after click on this then open one popup and in this popup you can get your Sandbox API Credentials.
We need following Paypal Sandbox Credentials
for make paypal card payment request.
METHOD – The name of the API call you’re making.
USER – The API username
PWD – The API password
SIGNATURE – The API signature
VERSION – The API version
You can also check full paypal document on click by this link Direct Payment Paypal API Docs.
Step - 2 Create route
After get you Sandbox API Credentials
then create following two routes.
Route::get('paypal-payment-form', 'PaypalController@index')->name('paypal-payment-form');
Route::get('paypal-payment-form-submit', 'PaypalController@payment')->name('paypal-payment-form-submit');
Step - 2 Create controller
After, done above two routes then we neet to create PaypalController.php
file help of following artisan command.
php artisan make:controller PaypalController
After run this commant your PaypalController.php
file automatic created on app/Http/Controllers
folder. just open it and write following code into that file.
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class PaypalController extends Controller
{
public function index(Request $request)
{
return view('paypal-payment-form');
}
public function payment(Request $request)
{
$request_params = array (
'METHOD' => 'DoDirectPayment',
'USER' => 'laracode101_api1.gmail.com',
'PWD' => 'BQJBSMHVP8WCEETS',
'SIGNATURE' => 'AyVuUcpetZUWCXV.EHq.qRhxEb6.AChQnxfoM9w6UJXXRBh2cH1GUawh',
'VERSION' => '85.0',
'PAYMENTACTION' => 'Sale',
'IPADDRESS' => '127.0.0.1',
'CREDITCARDTYPE' => 'Visa',
'ACCT' => '4032032452071167',
'EXPDATE' => '072023',
'CVV2' => '123',
'FIRSTNAME' => 'Yang',
'LASTNAME' => 'Ling',
'STREET' => '1 Main St',
'CITY' => 'San Jose',
'STATE' => 'CA',
'COUNTRYCODE' => 'US',
'ZIP' => '95131',
'AMT' => '100.00',
'CURRENCYCODE' => 'USD',
'DESC' => 'Testing Payments Pro'
);
$nvp_string = '';
foreach($request_params as $var=>$val)
{
$nvp_string .= '&'.$var.'='.urlencode($val);
}
$curl = curl_init();
curl_setopt($curl, CURLOPT_VERBOSE, 0);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($curl, CURLOPT_TIMEOUT, 30);
curl_setopt($curl, CURLOPT_URL, 'https://api-3t.sandbox.paypal.com/nvp');
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $nvp_string);
$result = curl_exec($curl);
curl_close($curl);
$data = $this->NVPToArray($result);
if($data['ACK'] == 'Success') {
# Database integration...
echo "Your payment was processed success.";
} if ($data['ACK'] == 'Failure') {
# Database integration...
echo "Your payment was declined/fail.";
} else {
echo "Something went wront please try again letter.";
}
}
public function NVPToArray($NVPString)
{
$proArray = array();
while(strlen($NVPString)) {
// key
$keypos= strpos($NVPString,'=');
$keyval = substr($NVPString,0,$keypos);
//value
$valuepos = strpos($NVPString,'&') ? strpos($NVPString,'&'): strlen($NVPString);
$valval = substr($NVPString,$keypos+1,$valuepos-$keypos-1);
$proArray[$keyval] = urldecode($valval);
$NVPString = substr($NVPString,$valuepos+1,strlen($NVPString));
}
return $proArray;
}
}
Here you can check following try response we will got from the paypal REST API server.
Success response
Array ( [TIMESTAMP] => 2012-04-16T08:15:41Z [CORRELATIONID] => 9a652cbabfdd9 [ACK] => Success [VERSION] => 85.0 [BUILD] => 2764190 [AMT] => 100.00 [CURRENCYCODE] => USD [AVSCODE] => X [CVV2MATCH] => M [TRANSACTIONID] => 6VR832690S591564M )
Fail response
Array ( [TIMESTAMP] => 2012-04-16T08:18:46Z [CORRELATIONID] => 2db182b912a9 [ACK] => Failure [VERSION] => 85.0 [BUILD] => 2764190 [L_ERRORCODE0] => 10527 [L_SHORTMESSAGE0] => Invalid Data [L_LONGMESSAGE0] => This transaction cannot be processed. Please enter a valid credit card number and type. [L_SEVERITYCODE0] => Error [AMT] => 100.00 [CURRENCYCODE] => USD )
Step - 3 Create blade file
After done controller file then we create one laravel blade HTML file which simple card payment design. Now we are create one simple blade file in resources/views/paypal-payment-form.blade.php
file and here we are make very simple html layout for make card payment.
After done this step then your card payment layout look something like, please seen following sceenshot
IMAGE
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-4">
<div class="card">
<div class="card-header">{{ __('Paypal Card Payment') }}</div>
<div class="card-body">
<form method="POST" action="{{ route('paypal-payment-form-submit') }}">
@csrf
<div class="form-group row">
<div class="col-md-12">
<input id="card_no" type="text" class="form-control @error('card_no') is-invalid @enderror" name="card_no" value="{{ old('card_no') }}" required autocomplete="card_no" placeholder="Card No." autofocus>
@error('card_no')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row">
<div class="col-md-6">
<input id="exp_month" type="text" class="form-control @error('exp_month') is-invalid @enderror" name="exp_month" value="{{ old('exp_month') }}" required autocomplete="exp_month" placeholder="Exp. Month (Eg. 02)" autofocus>
@error('exp_month')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
<div class="col-md-6">
<input id="exp_year" type="text" class="form-control @error('exp_year') is-invalid @enderror" name="exp_year" value="{{ old('exp_year') }}" required autocomplete="exp_year" placeholder="Exp. Year (Eg. 2020)" autofocus>
@error('exp_year')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row">
<div class="col-md-12">
<input id="cvv" type="password" class="form-control @error('cvv') is-invalid @enderror" name="cvv" required autocomplete="current-password" placeholder="CVV">
@error('cvv')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row mb-0">
<div class="col-md-12">
<button type="submit" class="btn btn-primary btn-block">
{{ __('PAY NOW') }}
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
@endsection
cUrl Request Data
if you want direct use cUrl request in your terminal or posman then you can use following code example.
curl https://api-3t.sandbox.paypal.com/nvp \
--insecure \
-d VERSION=56.0 \
-d SIGNATURE api_signature \
-d USER api_username \
-d PWD api_password \
-d METHOD=DoDirectPayment \
-d PAYMENTACTION=Sale \
-d IPADDRESS=192.168.0.1 \
-d AMT=8.88 \
-d CREDITCARDTYPE=Visa \
-d ACCT=4683075410516684 \
-d EXPDATE=042011 \
-d CVV2=123 \
-d FIRSTNAME=John \
-d LASTNAME=Smith \
-d STREET=1 Main St. \
-d CITY=San Jose \
-d STATE=CA \
-d ZIP=95131 \
-d COUNTRYCODE=US
cUrl Response Data
TIMESTAMP=...
&ACK=Success
&VERSION=56%2e0
&BUILD=1195961
&AMT=8%2e88
&CURRENCYCODE=USD
&AVSCODE=X
&CVV2MATCH=M
&TRANSACTIONID=...
&CORRELATIONID=...
I hope it can help you.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK