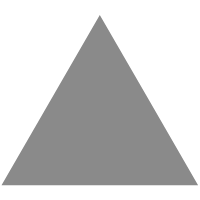
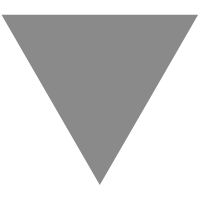
Dynamic Add Edit Delete Table Row in jQuery
source link: https://www.laravelcode.com/post/dynamic-add-edit-delete-table-row-in-jquery
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Dynamic Add Edit Delete Table Row in jQuery
In this article, I will share with you how to add edit delete table row using jquery. many times you need to build a CRUD application and in that application, you want to make add, edit, delete functionality on the same page without refreshing the page then you can be done this with simple jquery code. here i will share with you one simple example you can use this code in your web application.
i use here bootstrap CDN for making this simple add, update, edit , delete table row in same page.
Preview :

Example :
<!DOCTYPE html>
<html>
<head>
<title>Dynamic Add Edit Delete Table Row Example in jQuery - LaravelCode</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script>
</head>
<body>
<div class="container">
<h1>Dynamic Add Edit Delete Table Row Example in jQuery - LaravelCode</h1>
<form>
<div class="form-group">
<label>Name:</label>
<input type="text" name="name" class="form-control" value="Harsukh Makwana" required="">
</div>
<div class="form-group">
<label>Email:</label>
<input type="text" name="email" class="form-control" value="[email protected]" required="">
</div>
<button type="submit" class="btn btn-success save-btn">Save</button>
</form>
<br/>
<table class="table table-bordered data-table">
<thead>
<th>Name</th>
<th>Email</th>
<th width="200px">Action</th>
</thead>
<tbody>
</tbody>
</table>
</div>
<script type="text/javascript">
$("form").submit(function(e){
e.preventDefault();
var name = $("input[name='name']").val();
var email = $("input[name='email']").val();
$(".data-table tbody").append("<tr data-name='"+name+"' data-email='"+email+"'><td>"+name+"</td><td>"+email+"</td><td><button class='btn btn-info btn-xs btn-edit'>Edit</button><button class='btn btn-danger btn-xs btn-delete'>Delete</button></td></tr>");
$("input[name='name']").val('');
$("input[name='email']").val('');
});
$("body").on("click", ".btn-delete", function(){
$(this).parents("tr").remove();
});
$("body").on("click", ".btn-edit", function(){
var name = $(this).parents("tr").attr('data-name');
var email = $(this).parents("tr").attr('data-email');
$(this).parents("tr").find("td:eq(0)").html('<input name="edit_name" value="'+name+'">');
$(this).parents("tr").find("td:eq(1)").html('<input name="edit_email" value="'+email+'">');
$(this).parents("tr").find("td:eq(2)").prepend("<button class='btn btn-info btn-xs btn-update'>Update</button><button class='btn btn-warning btn-xs btn-cancel'>Cancel</button>")
$(this).hide();
});
$("body").on("click", ".btn-cancel", function(){
var name = $(this).parents("tr").attr('data-name');
var email = $(this).parents("tr").attr('data-email');
$(this).parents("tr").find("td:eq(0)").text(name);
$(this).parents("tr").find("td:eq(1)").text(email);
$(this).parents("tr").find(".btn-edit").show();
$(this).parents("tr").find(".btn-update").remove();
$(this).parents("tr").find(".btn-cancel").remove();
});
$("body").on("click", ".btn-update", function(){
var name = $(this).parents("tr").find("input[name='edit_name']").val();
var email = $(this).parents("tr").find("input[name='edit_email']").val();
$(this).parents("tr").find("td:eq(0)").text(name);
$(this).parents("tr").find("td:eq(1)").text(email);
$(this).parents("tr").attr('data-name', name);
$(this).parents("tr").attr('data-email', email);
$(this).parents("tr").find(".btn-edit").show();
$(this).parents("tr").find(".btn-cancel").remove();
$(this).parents("tr").find(".btn-update").remove();
});
</script>
</body>
</html>
i hope it will be help you.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK