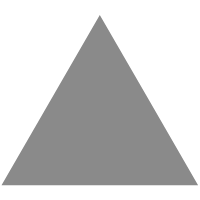
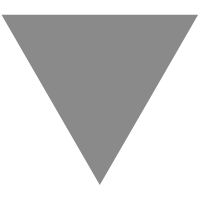
File Upload in PHP: Quick Tutorial
source link: https://codecondo.com/file-upload-in-php-quick-tutorial/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
What Is PHP File Upload?
Many websites provide file upload functionality. This means they allow users to upload files such as images, videos, PDFs, or documents, manage them, and potentially re-deliver these files to website users.
PHP is one of the most widely used programming languages for handling server-side applications for websites and web services. The PHP framework provides built-in functionality for uploading and validating files uploaded from the client side.
For example, you can easily create a PHP script that lets users upload documents to your site and saves them to a folder. The uploader itself will be relatively simple, but other tasks, such as quickly transferring files to users via CDN, managing large numbers of files, and transforming files into different formats (for example, converting .docx to .pdf) require more complex solutions.
Typically, PHP file upload functionality will consist of a PHP script together with an HTML form. Users interact with the HTML form, and the submit button triggers a script that uploads the files to a temporary directory and then moves them to the target location.
PHP POST Method File Upload
You can upload binary or text files using the PHP POST feature. PHP offers file manipulation and authentication capabilities, giving you complete control over who can upload and what users can do to files after upload. PHP supports file uploads from all browsers that comply with RFC-1867.
You can create a file upload page by setting up a special form. The form might describe the required format for the encoding type:
<!– Please specify the enctype as follows –>
<form enctype=”multipart/form-data” action=”__URL__” method=”POST”>
<!– Specify MAX_FILE_SIZE before file input –>
<input type=”hidden” name=”MAX_FILE_SIZE” value=”20000″ />
<!– The input element name determines the $_FILES array name –>
Send the file as follows: <input name=”examplefile” type=”file” />
<input type=”submit” value=”Send File” />
</form>
You can point to a PHP file by placing it in the __URL__ element.
According to the above example, the hidden MAX_FILE_SIZE field, measured in bytes, should precede the file input field. It should have a value equal to the maximum accepted file size that PHP supports. This element is important to include in the form because it prevents failed file transfers. Users thus avoid waiting for transfers of files that are too large.
Note that users can easily fool this setting from the browser side. Do not rely exclusively on this feature to block larger files—rather, you can view this as a guideline that adds convenience for client-side application users. However, the server-side PHP settings are fool-proof and can enforce the maximum file size.
The $_FILES element refers to global files containing all uploaded data. The contents of this form element, given our example form, should include the following:
- The original file name on the client side—$_FILES[‘examplefile’][‘name’].
- The file’s mime type— $_FILES[‘examplefile’][‘type’]. The browser might not always provide this information. PHP does not check the mime type, so you shouldn’t take this value for granted.
- The file’s temporary name—$_FILES[‘examplefile’][‘tmp_name’]. This is the file’s name during server-side storage.
- The uploaded file’s size (in bytes) -.$_FILES[‘examplefile’][‘size’].
- The full path—$_FILES[‘userexamplefile’][‘full_path’]. This is the value that the browser submitted, so it might not include an actual directory structure, and you cannot trust it.
- The file upload error code—$_FILES[‘examplefile’][‘error’].
These details refer to an uploaded file called “examplefile”, but you could use any file name.
The server stores files in its temporary directory by default, unless you specify another storage location via the upload_tmp_dir PHP directive. You can change the default server directory using the TMPDIR environment variable to set the PHP running environment. You cannot set the environment with putenv() in the PHP script.
You can also use the environment variable to verify that your other operations are functioning on uploaded files.These details refer to an uploaded file called “examplefile,” but you could use any file name. The server stores files in its temporary directory by default unless you specify another storage location via the upload_tmp_dir PHP directive. You can change the default server directory using the TMPDIR environment variable to set the PHP running environment. You cannot set the environment with putenv() in the PHP script. You can also use the environment variable to verify that your other operations are functioning on uploaded files.
PHP PUT Method File Upload
Another, often overlooked way to implement file upload in PHP is the PUT method. PHP supports uploading files via the HTTP PUT method, and this can be simpler to implement than via POST. However, it is also less secure and uploads must be carefully controlled and validated. If you are not careful, you could make the application vulnerable to local file injection (LFI) and similar attacks.
The user’s POST request will look something like this:
PUT /folder/file-to-upload.pdf HTTP/1.1
If this request is not specially handled, the server will save the file in the specified file within the application’s web root. Naturally, this is not advised for security and other reasons. To properly handle this type of request, you need to use a Script directive, specifying a PHP file that should run when the server receives a put request.
You can place a line like the following in the <Directory> block or <VirtualHost> block of your Apache configuration file, depending on whether you want it to apply to the entire server or specific paths:
Script PUT /uploadHandler.php
This instructs the web server to send PUT requests to your uploadHander script. Note that the destination resource for The destination resource for PUT requests should be the script itself, not the filename of the uploaded file.
Here is an example showing how to handle files submitted through PUT requests.
The following PHP code intercepts the PUT request and creates a file on the server to write the contents to:
$putdata = fopen(“php://input”, “r”);
$fp = fopen(“putfile.ext”, “w”);
The following code reads the data in increments of 1024 bytes and writes it to the file:
while ($data = fread($putdata, 1024))
fwrite($fp, $data);
Finally, we close the put streams:
fclose($fp);
fclose($putdata);
In addition to this basic functionality, it is mandatory to add authentication and file validation to the script, to prevent unsafe or malicious uploads to the server.
Conclusion
In this article, I explained the basics of PHP file upload and showed two methods for implementing your own PHP file upload script:
-
- Via POST method – adding a form to the page that allows a user to provide their file as an input and have it uploaded to the PHP server.
- Via PUT method – enabling a user to push the file directly to the server vs. PUT request (must be handled with care for security reasons).
I hope this will be useful as you learn to provide additional functionality for your PHP users.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK