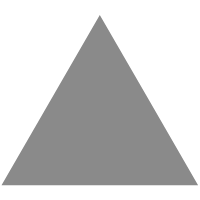
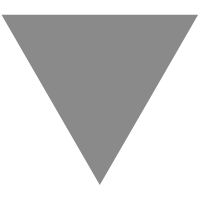
Online Sieve of Eratosthenes Demo via Go and Vue.js
source link: http://siongui.github.io/2018/05/01/sieve-of-eratosthenes-via-gopherjs-vue/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
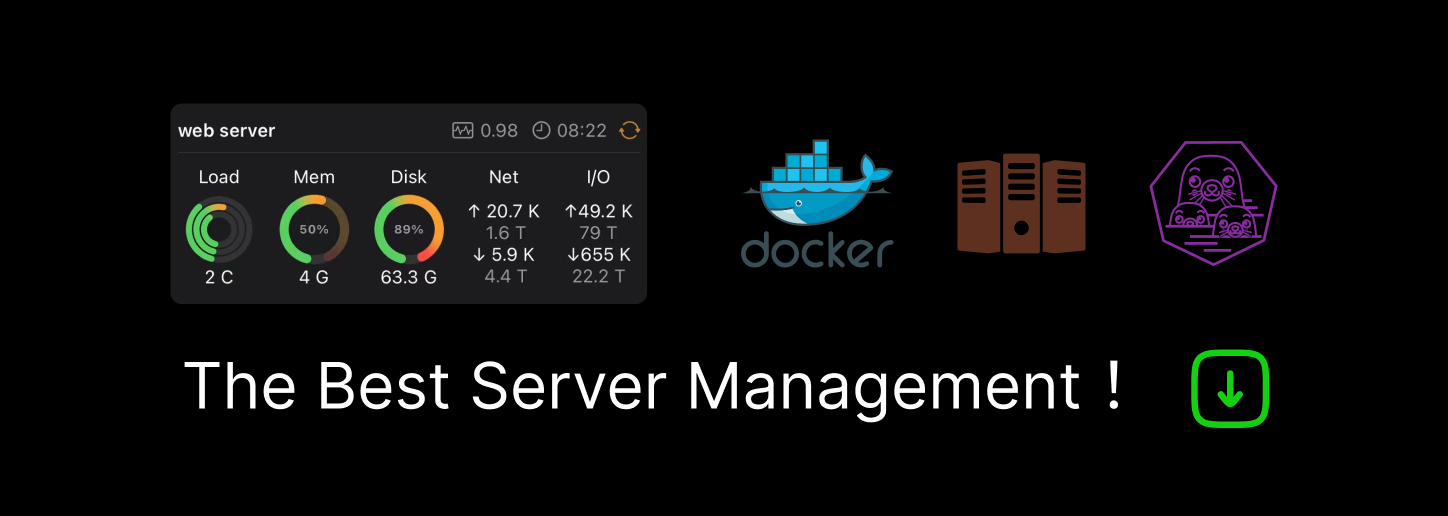
Online Sieve of Eratosthenes Demo via Go and Vue.js
May 01, 2018
Online Sieve of Eratosthenes demo via Go/GopherJS/gopherjs-vue (Go binding for Vue.js). Sieve of Eratosthenes is a method to find prime numbers up to a given limit. The algorithm comes from GeeksforGeeks [1] and implemented in Go. gopherjs-vue is used to build to UI interaction.
Although the following demo is written in JavaScript/Vue.js, the effect is the same as that written in Go.
The link of full source code is available on my GitHub repo.
HTML
<div id="vueapp"> <input v-model="intn" placeholder="Input even integer > 2"> <p>{{ result }}</p> </div>
Go: go get github.com/gopherjs/gopherjs and github.com/oskca/gopherjs-vue before compiling the Go code.
package main import ( "strconv" "github.com/gopherjs/gopherjs/js" "github.com/oskca/gopherjs-vue" ) type Model struct { *js.Object // this is needed for bidirectional data bindings Intn string `js:"intn"` Result string `js:"result"` } func SieveOfEratosthenes(n int) []int { // Create a boolean array "prime[0..n]" and initialize // all entries it as true. A value in prime[i] will // finally be false if i is Not a prime, else true. integers := make([]bool, n+1) for i := 2; i < n+1; i++ { integers[i] = true } for p := 2; p*p <= n; p++ { // If integers[p] is not changed, then it is a prime if integers[p] == true { // Update all multiples of p for i := p * 2; i <= n; i += p { integers[i] = false } } } // return all prime numbers <= n var primes []int for p := 2; p <= n; p++ { if integers[p] == true { primes = append(primes, p) } } return primes } func main() { m := &Model{ Object: js.Global.Get("Object").New(), } // field assignment is required in this way to make data passing works m.Intn = "4" m.Result = "" // create the VueJS viewModel using a struct pointer app := vue.New("#vueapp", m) opt := js.Global.Get("Object").New() opt.Set("immediate", true) app.Call("$watch", "intn", func(newVal, oldVal string) { n, err := strconv.Atoi(newVal) if err != nil { m.Result = "input must be integer" return } m.Result = "" primes := SieveOfEratosthenes(n) for _, prime := range primes { m.Result += (strconv.Itoa(prime) + " ") } }, opt) }
The JavaScript equivalent is available on my previous post [2].
Tested on:
- Chromium 65.0.3325.181 on Ubuntu 17.10 (64-bit)
- Go 1.10.1
- GopherJS 1.10-3
References:
[3][Golang] Sieve of Eratosthenes
[4][Vue.js] Online Goldbach's Conjecture Demo
[5]vue - GoDoc
Recommend
-
38
Lately, on invitation of my right honourable friend Michal , I've been trying to solve some problems from the Euler project and felt the need t...
-
9
RAGANWALD.COM Implementing the Sieve of Eratosthenes with Functional Programming Programming interviews often include a “Fizzbuzz” test,...
-
6
The sieve of Eratosthenes finds all prime numbers up to a given limit. Method The algorithm st...
-
11
In this article, we will learn to implement the Sieve of Eratosthenes using C++. If you’re a competitive programmer, most probably you’d be familiar with this term. But if you’re not, sit back and relax, we’ll see in the following sections wh...
-
9
Large Prime Check Using The Sieve Of Eratosthenes in C++ Filed Under: C++In this article, we will learn...
-
13
Prime Factorization Using The Sieve Of Eratosthenes in C++ Filed Under: JavaToday we will learn prime factoriz...
-
7
[JavaScript] Sieve of Eratosthenes April 28, 2018 JavaScript implementation...
-
21
[Vue.js] Online Sieve of Eratosthenes Demo May 02, 2018
-
7
[Golang] Sieve of Eratosthenes April 17, 2017 Go implementation of
-
10
The Ancient Greek polymath Eratosthenes of Cyrene made the first serious attempt at calculating the size of the Earth. Using very simple observations and mathematics he achieved a surprisingly accurate result and in this article I will replicate h...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK