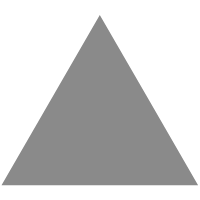
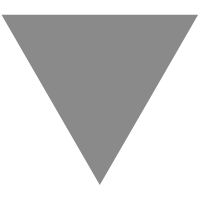
[Vue.js] Online Sieve of Eratosthenes Demo
source link: http://siongui.github.io/2018/05/02/vuejs-online-sieve-of-eratosthenes-demo/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
[Vue.js] Online Sieve of Eratosthenes Demo
May 02, 2018
Sieve of Eratosthenes is a simple and ancient method to find prime numbers up to a given limit. Given a limit, this online demo returns all prime numbers below the limit. The UI interaction is written using Vue.js, and the algorithm comes from GeeksforGeeks [1] and implemented using JavaScript.
[ 2, 3, 5 ]
HTML:
<div id="vueapp"> positive integer limit: <input v-model="intn" placeholder="Input positive integer"> <p>{{ primes }}</p> </div> <script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.js"></script>
Given an input from user, we check if the input is an integer. Then we run the sieve algorithm to check if the number is prime.
JavaScript:
'use strict'; function SieveOfEratosthenes(n) { // Create a boolean array "prime[0..n]" and initialize // all entries it as true. A value in prime[i] will // finally be false if i is Not a prime, else true. var integers = []; for (var i = 2; i < n+1; i++) { integers[i] = true; } for (var p = 2; p*p <= n; p++) { // If integers[p] is not changed, then it is a prime if (integers[p] == true) { // Update all multiples of p for (var i = p * 2; i <= n; i += p) { integers[i] = false; } } } // return all prime numbers <= n var primes = []; for (var p = 2; p <= n; p++) { if (integers[p] == true) { primes.push(p); } } return primes; } new Vue({ el: '#vueapp', data: { intn: 5, primes: "" }, watch: { intn: { immediate: true, handler(val, oldVal) { var n = parseInt(val); if (isNaN(n)) { this.primes = "please input integer"; return; } this.primes = SieveOfEratosthenes(n); } } } });
Tested on:
- Chromium 65.0.3325.181 on Ubuntu 17.10 (64-bit)
- Vue.js 2.5.16
References:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK