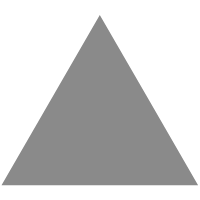
3
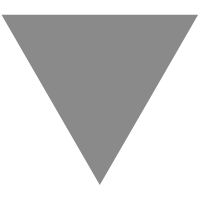
[Golang] Minify HTML
source link: http://siongui.github.io/2016/03/10/go-minify-html/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
[Golang] Minify HTML
March 10, 2016
Minify HTML via Golang.
The steps:
- Remove HTML comments [2]
- Remove all leading and trailing white space of each line.
- Pad a single space to the line if its length > 0.
package minhtml import ( "bufio" "bytes" "regexp" "strings" ) func RemoveHTMLComments(content []byte) []byte { // https://www.google.com/search?q=regex+html+comments // http://stackoverflow.com/a/1084759 htmlcmt := regexp.MustCompile(`<!--[^>]*-->`) return htmlcmt.ReplaceAll(content, []byte("")) } func MinifyHTML(html []byte) string { // read line by line minifiedHTML := "" scanner := bufio.NewScanner(bytes.NewReader(RemoveHTMLComments(html))) for scanner.Scan() { // all leading and trailing white space of each line are removed lineTrimmed := strings.TrimSpace(scanner.Text()) minifiedHTML += lineTrimmed if len(lineTrimmed) > 0 { // in case of following trimmed line: // <div id="foo" minifiedHTML += " " } } if err := scanner.Err(); err != nil { panic(err) } return minifiedHTML }
minhtml_test.go | repository | view raw
package minhtml import ( "io/ioutil" "testing" ) var html = []byte(` <!--- hello ---> <div id="foo" name="foo2"></div> <!-- world --> <span></span><!--ddd--> `) var htmlNoComments = []byte(` <div id="foo" name="foo2"></div> <span></span> `) var htmlMinified = `<div id="foo" name="foo2"></div> <span></span> ` func TestRemoveHTMLComments(t *testing.T) { if string(RemoveHTMLComments(html)) != string(htmlNoComments) { t.Error("Remove HTML comments failure!") } //println(string(RemoveHTMLComments(html))) } func TestMinifyHTML(t *testing.T) { if MinifyHTML(html) != htmlMinified { t.Error("Minify HTML failure!") } //println(MinifyHTML(html)) } func TestHTMLFile(t *testing.T) { htmlPath := "../../../output/index.html" b, err := ioutil.ReadFile(htmlPath) if err != nil { panic(err) } minifiedHTML := MinifyHTML(b) println(minifiedHTML) if err = ioutil.WriteFile(htmlPath, []byte(minifiedHTML), 0644); err != nil { panic(err) } }
Tested on: Ubuntu Linux 15.10, Go 1.6.
References:
[3]regexp - The Go Programming Language
[4]ioutil - The Go Programming Language
[5]bufio - The Go Programming Language
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK