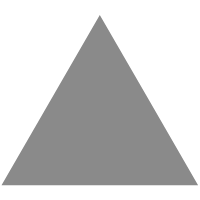
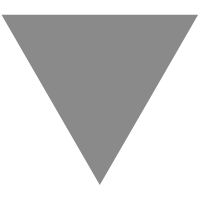
How to deal with Option data type in Scala
source link: https://blog.knoldus.com/how-to-deal-with-option-data-type-in-scala/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this blog post, we will talk about Option data type in Scala. Then we will discuss some of the methods for dealing with options.
Options
In Scala, an Option is a carrier of a single or no element for a specified type. An Option[T] object can be either Some[T] or None object, which represents a value that is not present. Thus, options mainly come into picture when we are dealing with a missing value or when we encounter null values.
sealed trait Option[+A]
case class Some[+A](get: A) extends Option[A]
case object None extends Option[Nothing]
Key Points :
- In Scala, the returned instance of an Option can be an instance of the Some or None classes, where Some and None are the children of Option class.
- When the value of a given key is obtained then Some class is generated.
- When the value of a given key is not obtained then None class is generated.
Let’s take an example
def findAverage(list: List[Double]): Option[Double] = {
if (list.isEmpty) None
else Some(list.sum / list.length)
}
In the above code, the return type shows the possibility that the result may not always be defined. We will always be able to retrieve a result of declared type (Option[Double]) from our function, so the findAverage is now atotal function.
For an instance, if a value corresponding to a given key is found, the get method of Scala’s Map returns Some(value), or None if the provided key is not defined in the Map.
Thus, option data type is a way of preventing null pointer exceptions.
Basic Functions to deal with Options
Option data type is frequently used in Scala programmes, and it can be compared to the null value available in Java, which indicates no value. For instance, the get method of java.util. HashMap returns either a value from the HashMap or null if no value was found. Let’s see some functions related to options.
1) getOrElse() Method
This method is used to return a value if it is present or a default value if it is not present. In this case, a value is returned for Some class and a default value is returned for None class.
object DemoOfOptions extends App {
val valueOne: Option[Int] = Some(8)
val valueTwo: Option[Int] = None
println("valueOne = " + valueOne.getOrElse(0))
println("valueTwo = " + valueTwo.getOrElse(15))
}
Output :- valueOne = 8
valueTwo = 15
Let's take a look at a code snippet that demonstrates getOrElse() method
2) isEmpty() Method
This method is used to determine whether the Option has a value or not. In this case, IsEmpty returns false for Some because it is not empty, but true for None because it is empty.
object DemoOfOptions extends App {
val valueOne: Option[Int] = Some(10)
val valueTwo: Option[Int] = None
println("valueOne = " + valueOne.isEmpty)
println("valueTwo = " + valueTwo.isEmpty)
}
Output :- valueOne = false
valueTwo = true
Let's take a look at a code snippet that demonstrates isEmpty() method
Conclusion
Thank you guys for making it to the end of the blog I hope you gained some knowledge on how we can implement Options in Scala. Then, we learned about some functions to deal with options.
Reference
For getting more knowledge regarding Options please refer to the following link
https://www.scala-lang.org/api/current/scala/Option.html
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK