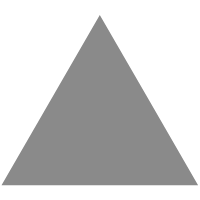
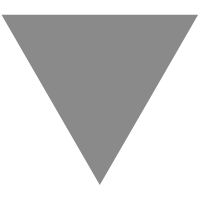
Data Driven Testing Framework using Selenium 4
source link: https://blog.knoldus.com/data-driven-testing-framework-using-selenium-4/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Data Driven Testing Framework using Selenium 4
Reading Time: 4 minutes
Hi folks,
Initially, In this blog we will go through the automation framework and its different types, then we will briefly see what and why part of the data driven testing framework. At the end of this blog, we will implement data driven testing using selenium 4.
Before understanding Data Driven Testing in selenium, Initially understand what exactly a test automation framework is.
We can say that a framework is a set of rules/assumptions/guidelines to achieve the expected goal. Likewise, a test automation framework is a platform that is a combination of programs, compilers and tools etc. It provides a test environment where you can execute your automated test scripts.
Types of Automation Frameworks :
There are a total 6 types of automation frameworks but we will briefly discuss the data driven testing framework.
What is data driven testing framework:
Mostly, when an application is tested by a tester manually then different scenarios are performed against different test data. The test data being used for manual testing is usually stored in some files like excel file, csv file, text file or in some database.
Likewise, for running automated tests against a large set of test data you can have different repositories/database/file to maintain the test data. A data driven testing framework is a technique in which you keep input test data separate from the actual test script.This DDT framework is totally dependent on the input test data. There are majorly two components in the data-driven testing framework. First is the test script and second test data.
The test data set is created in external sources such as an excel file, csv file, xml file, or any database. After that we connect the test script with test data to retrieve multiple sets of data to perform the application under test.
Let’s suppose an example, if there is a login page and you have to test it against distinct test data having a combination of characters, digits and alphanumeric, such test data you can store in excel or csv so that the same data you can input into the test scripts at the time of execution.
Have a look on flow diagram of a data driven testing framework:
In data driven process, There are four major things :
- Collecting test data in a storage like a file or a database.
- Creation of scripts that are capable of reading this data and executing test cases.
- Storing the actual outcomes and after that comparing the results with expected outcomes.
- Repeat the process with the next set of input data.
Why data driven testing framework is important ?
If we want to execute the same test with distinct test data then a data driven method is much better in itself. It saves both time and effort and improves efficiency.
Imagine a scenario that, you have a login system which you want to test for multiple input fields with different data sets.
you can test it using different approaches:
(Normal Approach) For every data set, create separate scripts and execute each of them one by one.
(Data Driven Approach) Import the data in an excel sheet and fetch this data one by one from the excel and run the script.
Executing the test scripts using a normal approach is a very time consuming and lengthy process, the data driven approach or what we call data-driven framework is ideal for such scenarios.
Implementation of data driven testing using selenium 4
Prerequisites
- Any IDE (I have used IntelliJ IDEA)
- Apache POI
- Selenium webdriver
- TestNG (optional)
- Test data in excel sheet
Initially, Make a maven project and add dependencies for Apache poi, Selenium webdriver and TestNG. After that make a Microsoft excel sheet and add your test data to it.
Here are the sample code snippets that I have used for reading the excel sheet and tested a dummy login page using excel test data.
Reading data from excel sheet
public class ExcelDataConfig {
//Making Global Variable for our workbook//
XSSFWorkbook wb;
//Accepting the Excel path through parameter and loading the Excel file//
public ExcelDataConfig(String excelpath) {
try {
FileInputStream fis = new FileInputStream(excelpath);
wb = new XSSFWorkbook(fis);
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
//Fetching data from Excel file//
public String getData(int sheetNumber, int row, int column) {
XSSFSheet sheet1 = wb.getSheetAt(sheetNumber);
if (sheet1.getRow(row).getCell(column).getCellType() == CellType.STRING)
return sheet1.getRow(row).getCell(column).getStringCellValue();
else if (sheet1.getRow(row).getCell(column).getCellType() == CellType.NUMERIC)
return String.valueOf(sheet1.getRow(row).getCell(column).getNumericCellValue());
else
throw new RuntimeException("no value found");
}
//Reading number of rows in Excel file//
public int getRowCount(int sheetIndex) {
int row = wb.getSheetAt(sheetIndex).getLastRowNum();
row = row + 1;
return row;
}
}
Testing a dummy login page using excel data
public class WordPressLogin {
//Making WebDriver's global variable//
WebDriver driver;
//Getting data from Excel sheet//
@DataProvider(name = "wordpressData")
public Object[][] passData() {
ExcelDataConfig config = new ExcelDataConfig("TestData/testdata.xlsx");
int rows = config.getRowCount(0);
Object[][] data = new Object[rows][2];
for (int i = 0; i < rows; i++) {
data[i][0] = config.getData(0, i, 0);
data[i][1] = config.getData(0, i, 1);
}
return data;
}
//Taking data from dataProvider and putting in selenium test//
//Testing a dummy login page using selenium webdriver//
@Test(dataProvider = "wordpressData")
public void loginToWordPress(String username, String password) throws InterruptedException
{
System.out.println(username);
System.out.println(password);
//Providing path of chromedriver//
System.setProperty("webdriver.chrome.driver", "src/test/chromedriver_linux64/chromedriver");
//Opening the chrome browser//
driver = new ChromeDriver();
//Maximizing the browser//
driver.manage().window().maximize();
//Putting implicit wait//
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
//Opening dummy login website//
driver.get("https://learner.demo.edunext.co/login?next=%2F");
//Locating different login fields//
driver.findElement(By.cssSelector("#login-email")).sendKeys(username);
driver.findElement(By.cssSelector("#login-password")).sendKeys(password);
driver.findElement(By.xpath("//button[contains(text(),'Sign in')]")).click();
}
//This tearDown function quits the driver after test executed//
@AfterMethod
public void tearDown() {
driver.quit();
}
}
That’s all for this blog and to explore more you can click here to reach out more related blogs.
References
https://www.selenium.dev/documentation/webdriver/
https://testng.org/doc/documentation-main.html

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK