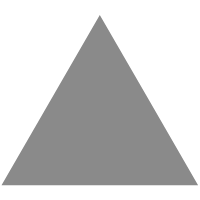
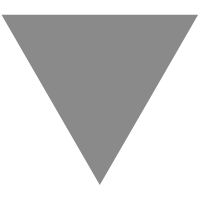
How to use Exception Handling Mechanism
source link: https://blog.knoldus.com/exception-handling-mechanism/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Reading Time: 4 minutes
Introduction:
One of the fundamental and important aspects of programming is Exception Handling. A program needs an environment to handle the potential errors and abnormal conditions. Programming does not end with writing code that executes and creates a result, as we also have to handle errors in a user-friendly manner.
There are several types of errors that could occur when we run a program. Some occur when the user enters bad data in their code. While some, such as a disk read error, occur when the hardware fails. And some occur when a programming error, such as a bad pointer, causes the program to fail.
We can manage and categorize error handling based on the property of error. For example, invalid user input is not a serious problem, And it prompts the user to re-enter the data. we can use provision in the code. On the other hand, a disk read or write failure is a non-recoverable error. Thus, to get a portion of the errors to be recuperated, they must be handled appropriately.
The exceptional handling mechanism we discuss here separates the code that discovers an error from the code that handles it.
Exception Hierarchy:
Handling Errors:
In general, we have two options to handle errors, especially those detected by the system:
1- Include code to intercept and handle the error.
2- Let the system handle it,i.e., ignore it.
Assuming we decide to handle the error, we can handle the error in the code that detects the error or we can use the exceptional handling approach that separates the detection and handling of errors.
A better way of optimizing errors is to use the exception handling approach of any of the programming languages, which we are using. An exception is an event that signals the occurrence of an error. Any language allows us to separate the code that may generate the error from the code that handles it.
The try statement throws an exception that is caught by the Catch statement.
By using exception handling, the problem or error can be thrown in the same function or different functions.
we can catch an exception in the same function that throws it. For example, we can catch and handle a divide-by-zero error or file-not-found error in main using the try and catch statement.
try {
Code that contains logic to throw an exception
}
catch {
case _ => errorHandler(e)
}
Try statement:
The try statement permits the programmer to bind the code that may cause an error. The try statement gives the power to the catch statement by throwing an exception. If there is no error, the try statement acts as a simple code, and the catch statement is ignored because the code works fine without it.
Catch statement:
The catch statement is an exception handler. It contains the logic which is important to manage the error. If no catch statement is provided when an error occurs, the problem aborts.
Using Exception Handling Mechanism:
Let’s understand it by an example. Following is the code for opening and reading a text file –
import scala.io.Source
val file_Name = "Knoldus.scala"
for (line <- Source.fromFile(file_Name).getLines) {
println(line)
}
file_Name.close
In this code, the File Not Found exception or IO exception can occur after passing the above content, as the source could be missing, as shown in the below line.
Exception: FileNotFoundException
Using Try Catch to remove this error. we insert the error type in the Catch statement and we can pass the message or command or any code in that condition.
And by using this Try and Catch statement, as shown below, our problem will get resolved.
var file_Name = "Knoldus.scala"
try {
text = openAndReadAFile(file_Name)
}
catch {
case e: FileNotFoundException => println("Couldn't find that file.")
case e: IOException => println("Had an IOException trying to read that file")
}
file_Name.close
Conclusion:
This topic will be used in plenty of useful places in your application. Don’t put the entire code in every place rather define the above method and use it everywhere. Make it a practice for all exceptions which a small of code may throw at runtime. Also, try to include a possible course of action, the user should follow in case these exceptions occur.
That’s all basic, I have in my mind as of now related to exception handling best practices, which could be understood by any beginner level programmer. The language does not matter. You can write in any language you want to write. If you are working on python, Except is used in place of Catch. So, focus on the logic. If you found anything missing or you do not relate to my view on any point, drop me a comment. I will be happy to discuss. For more blogs, click here
Happy Learning !!
References:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK