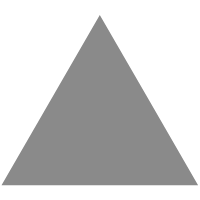
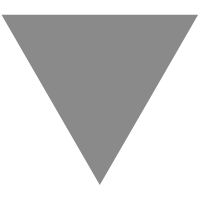
2 Ways Get File Extension in Javascript?
source link: https://codepedia.info/get-file-extension-javascript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
2 Ways Get File Extension in Javascript?
Get file extension in Javascript: In a web application, we use form tag to save user input. Primary form tag contains textbox, textarea and input file .i.e ( file upload ), etc. While data entering user can upload any file format, so we need to validate it on the client side as well as serverside. We as the developer knows which file extension or file type should be upload on the respective webpage. For that, file validation should be necessary, and for that, we need to know the extension of the uploaded file.
Here in this article, we going to learn how to get file extension in javascript ( client side), so we can validate it. We are using 2 different approaches to achieve it.
Two ways to get the file extension.
- Use Regex to get file type.
- Using split and pop method to get file type.
HTML Markup:
Here we added a form tag, with a file upload control and button tag. On button click, we call getFileExtention method, which gives an alert message and display the extension of the uploaded file. The code looks like as written below. <form action="/uploadFile">
<input id="myFile" type="file" name="file">
<button onclick="fnGetExtension()">Get Extension</button>
</form>
Method 1: Using regex to get file extension
Here we have regex value which gives us the file extension. This Regex is useful for extracting file extensions from URLs, even if URL contains ?foo=123 query strings or #hash values. Javascript Code: function fnGetExtension() {
//Get the file input element by its id
var fileInput = document.getElementById('myFile');
//Get the file name
var fileName = fileInput.files[0].name;
// Regular expression for file extension.
var patternFileExtension = /\.([0-9a-z]+)(?:[\?#]|$)/i;
//Get the file Extension
var fileExtension = (fileName).match(patternFileExtension);
alert(fileExtension);
}
Method 2: Using split and pop method to get file type.
Here we use split() method and use a dot (.) as a separator, and with pop() method we get the extension.
Javascript Code:
function fnGetExtension() {
//Get the file input element by its id
var fileInput = document.getElementById('myFile');
//Get the file name
var fileName = fileInput.files[0].name;
//Get the file Extension
var fileExtension = fileName.split('.').pop();
alert(fileExtension);
}
Conclusion: Here we have shown how to get file extension with and without using regular expression in javascript. This further can we used to file validation while uploading files.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK