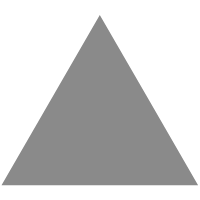
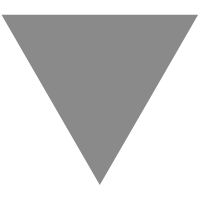
100个非常有用的Python技巧
source link: https://chegva.com/4409.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
100个非常有用的Python技巧
作者:Fatos Morina
编译:ronghuaiyang
Python现在非常流行,主要是因为它简单,容易学习。你可以用它来完成很多任务,比如数据科学和机器学习、web开发、脚本编写、自动化等。
这里总结了100条很有用的tips给你:
1. “for” 循环中的“Else”条件
除了目前为止你已经看到的所有Python代码之外,你有可能错过了下面的“for-else”,这也是我在几周前第一次看到的。
这是一个用于循环遍历列表的“for-else”方法,尽管对列表进行了迭代,但仍然有一个“else”条件,这是非常不寻常的。
这是我在Java、Ruby或JavaScript等其他编程语言中所没有看到的。
让我们看一个实践中的例子。
假设我们要检查列表中是否没有奇数。
让我们来遍历一下:
numbers = [2, 4, 6, 8, 1]
for number in numbers:
if number % 2 == 1:
print(number)
break
else:
print("No odd numbers")
如果我们发现一个奇数,那么这个数字将被打印,因为break将被执行,而else分支将被跳过。
否则,如果break从未被执行,执行流将继续执行else分支。
在这个例子中,我们将输出1。
2. 使用命名的变量从列表中取元素
my_list = [1, 2, 3, 4, 5]
one, two, three, four, five = my_list
3. 使用heapq从列表中获取最大或最小的元素
import heapq
scores = [51, 33, 64, 87, 91, 75, 15, 49, 33, 82]
print(heapq.nlargest(3, scores)) # [91, 87, 82]
print(heapq.nsmallest(5, scores)) # [15, 33, 33, 49, 51]
4. 把列表中的值作为参数传递给方法
可以使用" * "提取列表中的所有元素:
my_list = [1, 2, 3, 4]
print(my_list) # [1, 2, 3, 4]
print(*my_list) # 1 2 3 4
当我们想将列表中的所有元素作为方法参数传递时,这很有用:
def sum_of_elements(*arg):
total = 0
for i in arg:
total += i
return total
result = sum_of_elements(*[1, 2, 3, 4])
print(result) # 10
5. 获取列表的所有中间元素
_, *elements_in_the_middle, _ = [1, 2, 3, 4, 5, 6, 7, 8]
print(elements_in_the_middle) # [2, 3, 4, 5, 6, 7]
6. 一行赋值多个变量
one, two, three, four = 1, 2, 3, 4
7. 列表推导
你可以使用推导如,让我们将列表中的每个数字都取二次方:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [num * num for num in numbers]
print(squared_numbers)
推导不仅仅局限于使用列表。还可以将它们与字典、集合和生成器一起使用。
让我们看另一个例子,使用字典推导将一个字典的值提升到二阶:
Comprehensions are not just limited to working with lists. You can also use them with dictionaries, sets, and generators as well.
dictionary = {'a': 4, 'b': 5}
squared_dictionary = {key: num * num for (key, num) in dictionary.items()}
print(squared_dictionary) # {'a': 16, 'b': 25}
8. 通过Enum枚举相同概念的相关项
来自文档:
Enum是绑定到唯一值的一组符号名。它们类似于全局变量,但它们提供了更有用的repr()、分组、类型安全和其他一些特性。
下面是例子:
from enum import Enum
class Status(Enum):
NO_STATUS = -1
NOT_STARTED = 0
IN_PROGRESS = 1
COMPLETED = 2
print(Status.IN_PROGRESS.name) # IN_PROGRESS
print(Status.COMPLETED.value) # 2
9. 不使用循环来重复字符串
name = "Banana"
print(name * 4) # BananaBananaBananaBanana
10. 像数学式子一样比较3个数字
如果你有一个值,你想比较它是否在其他两个值之间,有一个简单的表达式,你在数学中使用:
1 < x < 10
这是我们在小学学过的代数表达式。但是,你也可以在Python中使用相同的表达式。
是的,你没听错。之前,你可能已经做过这种形式的比较:
1 < x and x < 10
现在,你只需要在Python中使用以下代码:
1 < x < 10
这在Ruby中行不通,Ruby是一种旨在让程序员开心的编程语言。这在JavaScript中也可以工作。
看到这样一个简单的表达没有得到更广泛的讨论,我真的很惊讶。至少,我还没有看到人们这么频繁地提到它。
11. 在单条语句中合并字典
从Python 3.9开始可用:
first_dictionary = {'name': 'Fatos', 'location': 'Munich'}
second_dictionary = {'name': 'Fatos', 'surname': 'Morina', 'location': 'Bavaria, Munich'}
result = first_dictionary | second_dictionary
print(result) # {'name': 'Fatos', 'location': 'Bavaria, Munich', 'surname': 'Morina'}
12. 在tuple中找到元素的索引
books = ('Atomic habits', 'Ego is the enemy', 'Outliers', 'Mastery')
print(books.index('Mastery')) # 3
13. 把字符串列表转换成一个列表
假设你在一个字符串函数中获得输入,但它应该是一个列表:
input = "[1,2,3]"
你不需要那种格式,你需要的是一个列表:
input = [1,2,3]
或者你可能从一个API调用得到以下响应:
input = [[1, 2, 3], [4, 5, 6]]
你所要做的就是导入模块ast,然后调用它的方法literal_eval,而不用去写复杂的正则表达式:
import ast
def string_to_list(string):
return ast.literal_eval(string)
这就是你需要做的。
现在你会得到一个列表作为结果,或者列表的列表,如下所示:
import ast
def string_to_list(string):
return ast.literal_eval(string)
string = "[[1, 2, 3],[4, 5, 6]]"
my_list = string_to_list(string)
print(my_list) # [[1, 2, 3], [4, 5, 6]]
14. 使用命名参数避免 “trivial” 错误
假设你想求两个数的差。差不是可交换的:
a - b != b -a
然而,我们可能会忘记参数的顺序,这可能会导致“trivial”错误:
def subtract(a, b):
return a - b
print((subtract(1, 3))) # -2
print((subtract(3, 1))) # 2
为了避免这种潜在的错误,我们可以简单地使用命名参数,参数的顺序不再重要:
def subtract(a, b):
return a - b
print((subtract(a=1, b=3))) # -2
print((subtract(b=3, a=1))) # -2
15. 使用单个print()语句打印多个元素
print(1, 2, 3, "a", "z", "this is here", "here is something else")
16. 一行打印多个元素
print("Hello", end="")
print("World") # HelloWorld
print("Hello", end=" ")
print("World") # Hello World
print('words', 'with', 'commas', 'in', 'between', sep=', ')
# words, with, commas, in, between
17. 打印多个值,每个值之间使用自定义分隔符
你可以很容易地做高级打印:
print("29", "01", "2022", sep="/") # 29/01/2022
print("name", "domain.com", sep="@") # [email protected]
18. 不能在变量名的开头使用数字
four_letters = “abcd” # this works
4_letters = “abcd” # this doesn’t work
19. 不能在变量名的开头使用操作符
+variable = “abcd” # this doesn’t work
20. 你不能把0作为数字的第一个数字
number = 0110 # this doesn't work
21. 你可以在变量名的任何位置使用下划线字符
这意味着,在任何你想要的地方,在变量名中,你想要多少次就有多少次:
a______b = "abcd" # this works
_a_b_c_d = "abcd" # this also works
我不鼓励你使用它,但如果你看到像这样奇怪的变量命名,要知道它实际上是一个有效的变量名。
22. 可以用下划线分隔较大的数字
这样读起来更容易。
print(1_000_000_000) # 1000000000
print(1_234_567) # 1234567
23. 颠倒列表的顺序
my_list = ['a', 'b', 'c', 'd']
my_list.reverse()
print(my_list) # ['d', 'c', 'b', 'a']
24. 使用step函数对字符串切片
my_string = "This is just a sentence"
print(my_string[0:5]) # This
# Take three steps forward
print(my_string[0:10:3]) # Tsse
25. 反向切片
my_string = "This is just a sentence"
print(my_string[10:0:-1]) # suj si sih
# Take two steps forward
print(my_string[10:0:-2]) # sjs i
26. 只有开始或结束索引的部分切片
表示切片的开始和结束的索引可以是可选的。
my_string = "This is just a sentence"
print(my_string[4:]) # is just a sentence
print(my_string[:3]) # Thi
27. Floor 除法
print(3/2) # 1.5
print(3//2) # 1
28. == 和 “is” 的差别
" is "检查两个变量是否指向内存中的同一个对象。
" == "比较这两个对象的值是否相等。
first_list = [1, 2, 3]
second_list = [1, 2, 3]
# Is their actual value the same?
print(first_list == second_list) # True
# Are they pointing to the same object in memory
print(first_list is second_list)
# False, since they have same values, but in different objects in memory
third_list = first_list
print(third_list is first_list)
# True, since both point to the same object in memory
29. 更改分配给另一个变量的变量的值
当一个变量被赋值给另一个变量时,它的值实际上被复制到第二个变量中。
这意味着第一个变量之后的任何变化都不会反映在第二个变量中:
first = "An initial value"
second = first
first = "An updated value"
print(first) # An updated value
print(second) # An initial value
30. 检查一个字符串是否大于另一个字符串
first = "abc"
second = "def"
print(first < second) # True
second = "ab"
print(first < second) # False
31. 检查字符串是不是从特定字符开始的
my_string = "abcdef"
print(my_string.startswith("b")) # False
32. 使用id()找到变量的唯一id
print(id(1)) # 4325776624
print(id(2)) # 4325776656
print(id("string")) # 4327978288
33. Integers, floats, strings, booleans, sets以及tuples是不可修改的
当将变量赋给整数、浮点数、字符串、布尔值、集合和元组等不可变类型时,该变量将指向内存中的对象。
如果给该变量赋了另一个值,原来的对象仍然在内存中,但是指向它的变量丢失了:
number = 1
print(id(number)) # 4325215472
print(id(1)) # 4325215472
number = 3
print(id(number)) # 4325215536
print(id(1)) # 4325215472
34. Strings 和 tuples 是不可修改的
这一点在上一点中已经提到过,但我想强调一下,因为这是非常重要的。
name = "Fatos"
print(id(name)) # 4422282544
name = "fatos"
print(id(name)) # 4422346608
my_tuple = (1, 2, 3, 4)
print(id(my_tuple)) # 4499290128
my_tuple = ('a', 'b')
print(id(my_tuple)) # 4498867584
35. Lists, sets, 和 dictionaries 是不可修改的
这意味着我们可以在不丢失绑定的情况下更改对象:
cities = ["Munich", "Zurich", "London"]
print(id(cities)) # 4482699712
cities.append("Berlin")
print(id(cities)) # 4482699712
下面是另一个集合的例子:
my_set = {1, 2, 3, 4}
print(id(my_set)) # 4352726176
my_set.add(5)
print(id(my_set)) # 4352726176
36. 你可以把集合转换为不可修改的集合
这样,你就不能再修改它了:
my_set = frozenset(['a', 'b', 'c', 'd'])
my_set.add("a")
如果你这样做,就会抛出一个错误:
AttributeError: 'frozenset' object has no attribute 'add'
37. " if-elif "块可以在没有else块的情况下存在
但是,如果前面没有“if”,“elif”就不能独立存在:
def check_number(number):
if number > 0:
return "Positive"
elif number == 0:
return "Zero"
return "Negative"
print(check_number(1)) # Positive
38. 使用sorted()查看2个字符串是否是相同的字母但次序不一样
def check_if_anagram(first_word, second_word):
first_word = first_word.lower()
second_word = second_word.lower()
return sorted(first_word) == sorted(second_word)
print(check_if_anagram("testinG", "Testing")) # True
print(check_if_anagram("Here", "Rehe")) # True
print(check_if_anagram("Know", "Now")) # False
39. 获取一个字符的Unicode值
print(ord("A")) # 65
print(ord("B")) # 66
print(ord("C")) # 66
print(ord("a")) # 97
40. 一行代码获取字典中所有的keys
dictionary = {"a": 1, "b": 2, "c": 3}
keys = [i for i, _ in dictionary.items()]
print(keys) # ['a', 'b', 'c']
41. 一行代码获取字典中所有的值
dictionary = {"a": 1, "b": 2, "c": 3}
values = [i for _, i in dictionary.items()]
print(values) # [1, 2, 3]
42. 交换字典中的keys和values
dictionary = {"a": 1, "b": 2, "c": 3}
reversed_dictionary = {j: i for i, j in dictionary.items()}
print(reversed) # {1: 'a', 2: 'b', 3: 'c'}
43. 你可以将布尔型值转换为数字
print(int(False)) # 0
print(float(True)) # 1.0
44. 你可以算术操作中使用布尔值
“False”是0,而“True”是1。
x = 10
y = 12
result = (x - False)/(y * True)
print(result) # 0.8333333333333334
45. 你可以将任何数据的类型转换为布尔值
print(bool(.0)) # False
print(bool(3)) # True
print(bool("-")) # True
print(bool("string")) # True
print(bool(" ")) # True
46. 将一个值转换为复数
print(complex(10, 2)) # (10+2j)
也可以将数字转换为十六进制数:
print(hex(11)) # 0xb
47. 把值加到列表的第一个位置
如果你使用append(),你将从右边插入新的值。
也可以使用*insert()*来指定要插入新元素的索引和元素。在我们的例子中,我们想把它插入到第一个位置,所以我们使用0作为下标:
my_list = [3, 4, 5]
my_list.append(6)
my_list.insert(0, 2)
print(my_list) # [2, 3, 4, 5, 6]
48. Lambda方法只能在一行里
在使用lambdas方法的时候,不能超过一行。
让我们试试以下方法:
comparison = lambda x: if x > 3:
print("x > 3")
else:
print("x is not greater than 3")
将抛出以下错误:
result = lambda x: if x > 3:
^
SyntaxError: invalid syntax
49. lambda中的条件语句应该始终包含“else”部分
我们试下下面的:
comparison = lambda x: "x > 3" if x > 3
我们将得到以下错误:
comparison = lambda x: "x > 3" if x > 3
^
SyntaxError: invalid syntax
50. filter() 返回一个新的对象
my_list = [1, 2, 3, 4]
odd = filter(lambda x: x % 2 == 1, my_list)
print(list(odd)) # [1, 3]
print(my_list) # [1, 2, 3, 4]
51. map() 返回一个新的对象
my_list = [1, 2, 3, 4]
squared = map(lambda x: x ** 2, my_list)
print(list(squared)) # [1, 4, 9, 16]
print(my_list) # [1, 2, 3, 4]
52. range() 中有一个步长的参数,但是知道的并不多
for number in range(1, 10, 3):
print(number, end=" ")
# 1 4 7
53. range() 默认从0开始
所以你根本不需要包含它。
def range_with_zero(number):
for i in range(0, number):
print(i, end=' ')
def range_with_no_zero(number):
for i in range(number):
print(i, end=' ')
range_with_zero(3) # 0 1 2
range_with_no_zero(3) # 0 1 2
54. 不需要将长度和0比较
如果长度大于0,则默认为True,所以你不需要将其与0进行比较:
def get_element_with_comparison(my_list):
if len(my_list) > 0:
return my_list[0]
def get_first_element(my_list):
if len(my_list):
return my_list[0]
elements = [1, 2, 3, 4]
first_result = get_element_with_comparison(elements)
second_result = get_element_with_comparison(elements)
print(first_result == second_result) # True
55. 可以在同一范围内多次定义相同的方法
但是,只有最后一个被调用,因为它覆盖了以前的。
def get_address():
return "First address"
def get_address():
return "Second address"
def get_address():
return "Third address"
print(get_address()) # Third address
56. 你可以访问私有属性
class Engineer:
def __init__(self, name):
self.name = name
self.__starting_salary = 62000
dain = Engineer('Dain')
print(dain._Engineer__starting_salary) # 62000
57. 查看对象的内存使用
import sys
print(sys.getsizeof("bitcoin")) # 56
58. 定义一个方法,调用的时候想传多少参数都可以
def get_sum(*arguments):
result = 0
for i in arguments:
result += i
return result
print(get_sum(1, 2, 3)) # 6
print(get_sum(1, 2, 3, 4, 5)) # 15
print(get_sum(1, 2, 3, 4, 5, 6, 7)) # 28
59. 使用super() 或者父类的名字调用父类的初始化
使用*super()*调用父类初始化器:
class Parent:
def __init__(self, city, address):
self.city = city
self.address = address
class Child(Parent):
def __init__(self, city, address, university):
super().__init__(city, address)
self.university = university
child = Child('Zürich', 'Rämistrasse 101', 'ETH Zürich')
print(child.university) # ETH Zürich
使用父类的名称调用父类:
class Parent:
def __init__(self, city, address):
self.city = city
self.address = address
class Child(Parent):
def __init__(self, city, address, university):
Parent.__init__(self, city, address)
self.university = university
child = Child('Zürich', 'Rämistrasse 101', 'ETH Zürich')
print(child.university) # ETH Zürich
注意,使用**init()和super()**调用父类初始化器只能在子类的初始化器中使用。
60. 你可以在自己的类中重新定义“+”操作符
当你在两个int数据类型之间使用**+**操作符时,你将得到它们的和。
然而,当你在两个字符串数据类型之间使用它时,你将合并它们:
print(10 + 1) # Adding two integers using '+'
print('first' + 'second') # Merging two strings '+'
这表示操作符重载。
你也可以在你自己的类中使用它:
class Expenses:
def __init__(self, rent, groceries):
self.rent = rent
self.groceries = groceries
def __add__(self, other):
return Expenses(self.rent + other.rent,
self.groceries + other.groceries)
april_expenses = Expenses(1000, 200)
may_expenses = Expenses(1000, 300)
total_expenses = april_expenses + may_expenses
print(total_expenses.rent) # 2000
print(total_expenses.groceries) # 500
61. 你还可以在自己的类中重新定义“<”和“=”操作符Y
下面是另一个你可以自己定义的操作重载的例子:
class Game:
def __init__(self, score):
self.score = score
def __lt__(self, other):
return self.score < other.score
first = Game(1)
second = Game(2)
print(first < second) # True
类似地,就像前面的两种情况,我们可以根据自己的需要重写*eq()*函数:
class Journey:
def __init__(self, location, destination, duration):
self.location = location
self.destination = destination
self.duration = duration
def __eq__(self, other):
return ((self.location == other.location) and
(self.destination == other.destination) and
(self.duration == other.duration))
first = Journey('Location A', 'Destination A', '30min')
second = Journey('Location B', 'Destination B', '30min')
print(first == second)
你也可以类似地定义:
- sub() for -
- mul() for *****
- truediv() for /
- ne() for !=
- ge() for >=
- gt() for >
62. 你可以为类的对象定义一个自定义的可打印版本
class Rectangle:
def __init__(self, a, b):
self.a = a
self.b = b
def __repr__(self):
return repr('Rectangle with area=' + str(self.a * self.b))
print(Rectangle(3, 4)) # 'Rectangle with area=12'
63. 交换字符串中的字符大小写
string = "This is just a sentence."
result = string.swapcase()print(result) # tHIS IS JUST A SENTENCE.
64. 检查是否所有字符都是字符串中的空格
string = " "
result = string.isspace()print(result) # True
65. 检查字符串中的所有字符是否都是字母或数字
name = "Password"
print(name.isalnum()) # True, because all characters are alphabetsname = "Secure Password "
print(name.isalnum()) # False, because it contains whitespacesname = "S3cur3P4ssw0rd"
print(name.isalnum()) # Truename = "133"
print(name.isalnum()) # True, because all characters are numbers
66. 检查字符串中的所有字符是否都是字母
string = "Name"
print(string.isalpha()) # Truestring = "Firstname Lastname"
print(string.isalpha()) # False, because it contains whitespacestring = “P4ssw0rd”
print(string.isalpha()) # False, because it contains numbers
67. 根据参数从右侧移除字符
string = "This is a sentence with "
# Remove trailing spaces from the right
print(string.rstrip()) # "This is a sentence with"
string = "this here is a sentence…..,,,,aaaaasd"
print(string.rstrip(“.,dsa”)) # "this here is a sentence"
类似地,你可以根据参数从左边删除字符:
string = "ffffffffFirst"
print(string.lstrip(“f”)) # First
68. 检查一个字符串是否代表一个数字
string = "seven"
print(string.isdigit()) # False
string = "1337"
print(string.isdigit()) # True
string = "5a"
print(string.isdigit()) # False, because it contains the character 'a'
string = "2**5"
print(string.isdigit()) # False
69. 检查一个字符串是否代表一个中文数字
# 42673 in Arabic numerals
string = "四二六七三"
print(string.isdigit()) # False
print(string.isnumeric()) # True
70. 检查一个字符串是否所有的单词都以大写字母开头
string = "This is a sentence"
print(string.istitle()) # False
string = "10 Python Tips"
print(string.istitle()) # True
string = "How to Print A String in Python"
# False, because of the first characters being lowercase in "to" and "in"
print(string.istitle())
string = "PYTHON"
print(string.istitle()) # False. It's titlelized version is "Python"
71. 我们也可以在元组中使用负索引
numbers = (1, 2, 3, 4)
print(numbers[-1]) # 4
print(numbers[-4]) # 1
72. 在元组中嵌套列表和元组
mixed_tuple = (("a"*10, 3, 4), ['first', 'second', 'third'])
print(mixed_tuple[1]) # ['first', 'second', 'third']
print(mixed_tuple[0]) # ('aaaaaaaaaa', 3, 4)
73. 快速计算满足条件的元素在列表中出现的次数
names = ["Besim", "Albert", "Besim", "Fisnik", "Meriton"]
print(names.count("Besim")) # 2
74. 使用slice()可以方便的得到最近的元素
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
slicing = slice(-4, None)
# Getting the last 3 elements from the list
print(my_list[slicing]) # [4, 5, 6]
# Getting only the third element starting from the right
print(my_list[-3]) # 4
你也可以使用*slice()*来完成其他常见的切片任务,比如:
string = "Data Science"
# start = 1, stop = None (don't stop anywhere), step = 1
# contains 1, 3 and 5 indices
slice_object = slice(5, None)
print(string[slice_object]) # Science
75. 计算元素在元组中出现的次数
my_tuple = ('a', 1, 'f', 'a', 5, 'a')
print(my_tuple.count('a')) # 3
76. 获取元组中元素的索引
my_tuple = ('a', 1, 'f', 'a', 5, 'a')
print(my_tuple.index('f')) # 2
77. 通过跳转获取子元组
my_tuple = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
print(my_tuple[::3]) # (1, 4, 7, 10)
78. 从索引开始获取子元组
my_tuple = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
print(my_tuple[3:]) # (4, 5, 6, 7, 8, 9, 10)
79. 从列表、集合或字典中删除所有元素
my_list = [1, 2, 3, 4]
my_list.clear()
print(my_list) # []
my_set = {1, 2, 3}
my_set.clear()
print(my_set) # set()
my_dict = {"a": 1, "b": 2}
my_dict.clear()
print(my_dict) # {}
80. 合并2个集合
一种方法是使用方法union(),它将作为合并的结果返回一个新的集合:
first_set = {4, 5, 6}
second_set = {1, 2, 3}
print(first_set.union(second_set)) # {1, 2, 3, 4, 5, 6}
另一个是方法update,它将第二个集合的元素插入到第一个集合中:
first_set = {4, 5, 6}
second_set = {1, 2, 3}
first_set.update(second_set)
print(first_set) # {1, 2, 3, 4, 5, 6}
81. 打印函数内的条件语句
def is_positive(number):
print("Positive" if number > 0 else "Negative") # Positive
is_positive(-3)
82. 一个if语句中包含多个条件
math_points = 51
biology_points = 78
physics_points = 56
history_points = 72
my_conditions = [math_points > 50, biology_points > 50,
physics_points > 50, history_points > 50]
if all(my_conditions):
print("Congratulations! You have passed all of the exams.")
else:
print("I am sorry, but it seems that you have to repeat at least one exam.")
# Congratulations! You have passed all of the exams.
83. 在一个if语句中至少满足一个条件
math_points = 51
biology_points = 78
physics_points = 56
history_points = 72
my_conditions = [math_points > 50, biology_points > 50,
physics_points > 50, history_points > 50]
if any(my_conditions):
print("Congratulations! You have passed all of the exams.")
else:
print("I am sorry, but it seems that you have to repeat at least one exam.")
# Congratulations! You have passed all of the exams.
84. 任何非空字符串都被计算为True
print(bool("Non empty")) # True
print(bool("")) # False
85. 任何非空列表、元组或字典都被求值为True
print(bool([])) # False
print(bool(set([]))) # False
print(bool({})) # False
print(bool({"a": 1})) # True
86. 其他计算为False的值是None、“False”和数字0
print(bool(False)) # False
print(bool(None)) # False
print(bool(0)) # False
87. 你不能仅仅通过在函数中提及全局变量来改变它的值
string = "string"
def do_nothing():
string = "inside a method"
do_nothing()
print(string) # string
你也需要使用访问修饰符global:
string = "string"
def do_nothing():
global string
string = "inside a method"
do_nothing()
print(string) # inside a method
88. 使用“collections”中的Counter计算字符串或列表中的元素数量
from collections import Counter
result = Counter("Banana")
print(result) # Counter({'a': 3, 'n': 2, 'B': 1})
result = Counter([1, 2, 1, 3, 1, 4, 1, 5, 1, 6])
print(result) # Counter({1: 5, 2: 1, 3: 1, 4: 1, 5: 1, 6: 1})
89. 使用Counter检查是否2个字符串包含相同的字符
from collections import Counter
def check_if_anagram(first_string, second_string):
first_string = first_string.lower()
second_string = second_string.lower()
return Counter(first_string) == Counter(second_string)
print(check_if_anagram('testinG', 'Testing')) # True
print(check_if_anagram('Here', 'Rehe')) # True
print(check_if_anagram('Know', 'Now')) # False
你也可以使用*sorted()*检查两个字符串是否具有相同的字符:
def check_if_anagram(first_word, second_word):
first_word = first_word.lower()
second_word = second_word.lower()
return sorted(first_word) == sorted(second_word)
print(check_if_anagram("testinG", "Testing")) # True
print(check_if_anagram("Here", "Rehe")) # True
print(check_if_anagram("Know", "Now")) # False
90. 使用" itertools "中的" Count "计算元素的数量
from itertools import count
my_vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
current_counter = count()
string = "This is just a sentence."
for i in string:
if i in my_vowels:
print(f"Current vowel: {i}")
print(f"Number of vowels found so far: {next(current_counter)}")
这是控制台中的结果:
Current vowel: i
Number of vowels found so far: 0
Current vowel: i
Number of vowels found so far: 1
Current vowel: u
Number of vowels found so far: 2
Current vowel: a
Number of vowels found so far: 3
Current vowel: e
Number of vowels found so far: 4
Current vowel: e
Number of vowels found so far: 5
Current vowel: e
Number of vowels found so far: 6
91. 根据字符串或列表的频率对元素进行排序
来自collections模块的Counter默认情况下不会根据元素的频率来排序。
from collections import Counter
result = Counter([1, 2, 3, 2, 2, 2, 2])
print(result) # Counter({2: 5, 1: 1, 3: 1})
print(result.most_common()) # [(2, 5), (1, 1), (3, 1)]
92. 在一行中找到列表中出现频次最高的元素
my_list = ['1', 1, 0, 'a', 'b', 2, 'a', 'c', 'a']
print(max(set(my_list), key=my_list.count)) # a
93. copy()和deepcopy()的区别
来自文档中的解释:
浅拷贝构造一个新的复合对象,然后(在可能的范围内)在其中插入对原始对象的引用。深拷贝构造一个新的复合对象,然后递归地将在原始对象中找到的对象的副本插入其中。
更全面的描述:
浅拷贝意味着构造一个新的集合对象,然后用对原始集合中的子对象的引用填充它。从本质上说,浅拷贝的深度只有一层。拷贝过程不会递归,因此不会创建子对象本身的副本。深拷贝使拷贝过程递归。这意味着首先构造一个新的集合对象,然后用在原始集合对象中找到的子对象的副本递归地填充它。以这种方式拷贝对象将遍历整个对象树,以创建原始对象及其所有子对象的完全独立的克隆。
这里是copy()的例子:
first_list = [[1, 2, 3], ['a', 'b', 'c']]
second_list = first_list.copy()
first_list[0][2] = 831
print(first_list) # [[1, 2, 831], ['a', 'b', 'c']]
print(second_list) # [[1, 2, 831], ['a', 'b', 'c']]
这个是deepcopy() 的例子:
import copy
first_list = [[1, 2, 3], ['a', 'b', 'c']]
second_list = copy.deepcopy(first_list)
first_list[0][2] = 831
print(first_list) # [[1, 2, 831], ['a', 'b', 'c']]
print(second_list) # [[1, 2, 3], ['a', 'b', 'c']]
94. 当试图访问字典中不存在的键时,可以避免抛出错误
如果你使用一个普通的字典,并试图访问一个不存在的键,那么你将得到一个错误:
my_dictonary = {"name": "Name", "surname": "Surname"}
print(my_dictonary["age"])
下面是抛出的错误:
KeyError: 'age'
我们可以使用defaultdict():来避免这种错误
from collections import defaultdict
my_dictonary = defaultdict(str)
my_dictonary['name'] = "Name"
my_dictonary['surname'] = "Surname"
print(my_dictonary["age"])
95. 你可以构建自己的迭代器
class OddNumbers:
def __iter__(self):
self.a = 1
return self
def __next__(self):
x = self.a
self.a += 2
return x
odd_numbers_object = OddNumbers()
iterator = iter(odd_numbers_object)
print(next(iterator)) # 1
print(next(iterator)) # 3
print(next(iterator)) # 5
96. 可以用一行从列表中删除重复项
my_set = set([1, 2, 1, 2, 3, 4, 5])
print(list(my_set)) # [1, 2, 3, 4, 5]
97. 打印模块所在的位置
import torch
print(torch) # <module 'torch' from '/Users/...'
98. 可以使用" not in "来检查值是否不属于列表
odd_numbers = [1, 3, 5, 7, 9]
even_numbers = []
for i in range(9):
if i not in odd_numbers:
even_numbers.append(i)
print(even_numbers) # [0, 2, 4, 6, 8]
99. sort() 和 sorted()的差别
sort()对原始列表进行排序。
sorted()返回一个新的排序列表。
groceries = ['milk', 'bread', 'tea']
new_groceries = sorted(groceries)
# new_groceries = ['bread', 'milk', 'tea']
print(new_groceries)
# groceries = ['milk', 'bread', 'tea']
print(groceries)
groceries.sort()
# groceries = ['bread', 'milk', 'tea']
print(groceries)
100. 使用uuid模块生成唯一的id
UUID代表统一唯一标识符。
import uuid
# Generate a UUID from a host ID, sequence number, and the current time
print(uuid.uuid1()) # 308490b6-afe4-11eb-95f7-0c4de9a0c5af
# Generate a random UUID
print(uuid.uuid4()) # 93bc700b-253e-4081-a358-24b60591076a
转载自:https://mp.weixin.qq.com/s/79h9nd37HcvwyMJSMhJ30A

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK