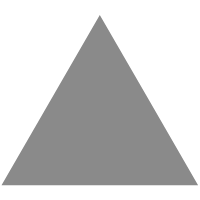
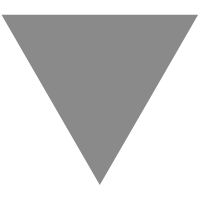
How to get a list of files in a directory using node.js
source link: https://typeofnan.dev/how-to-get-a-list-of-files-in-a-directory-using-nodejs/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to get a list of files in a directory using node.js
Nick Scialli • May 24, 2021 • 🚀 1 minute read
Automation is your friend. Let’s use node.js to automatically get a list of files in a directory.
First, let’s quickly create a few files in our current directory and then an index.js
file:
touch file1.txt file2.txt file3.txt index.js
Next up, let’s edit the index.js
file. We will use the fs
(file system) module, which we have to import.
const fs = require('fs');
At this point, we have a couple options: we can get the files asynchronously, which means we’ll need a callback function, or we can get the files synchronously.
fs.readdir (asynchronously)
fs.readdir
takes two arguments: the file path to the directory you want to read and then a callback function. The callback function takes an error
argument in first spot and a list of files in the second spot.
const fs = require('fs');
fs.readdir('./', (err, files) => {
if (err) {
return console.error(err);
}
console.log(files);
// ["file1.txt", "file2.txt", "file3.txt", "index.js"]
});
Assuming there are no errors, this lists our three text files and the index.js
file in the current directory.
fs.readdirSync (synchronously)
fs.readdirSync
has a simpler interface, but there are a couple downsides: 1) we end up blocking the execution of future code until the directory read is complete and 2) we have to make sure we handle any errors using try/catch
.
const fs = require('fs');
try {
console.log(fs.readdirSync('./'));
// ["file1.txt", "file2.txt", "file3.txt", "index.js"]
} catch (e) {
console.error(e);
}
And those are two quick ways to get a list of files in a directory using node.js!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK