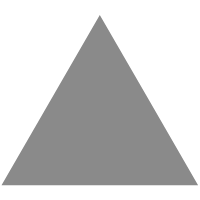
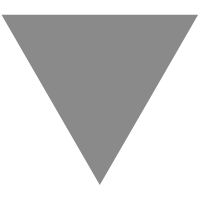
Spring Interceptors: How to use them correctly?
source link: https://blog.knoldus.com/spring-interceptors/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Spring Interceptors: How to use them correctly?
Reading Time: 2 minutes
Hi folks! we’ll focus on understanding the Spring Interceptors and how to use it correctly.
What is Spring interceptors
When a request is sent to spring controller, it will have to pass through Spring Interceptors (0 or more) before being processed by Controller.
Spring Interceptor is only applied to requests that are sending to a Controller.
Spring Interceptor – HandlerInterceptor
- preHandle(): This method is used to intercept the request before it’s handed over to the Controller. This method should return ‘true’ to let Spring know to process the request through another spring interceptor or to send it to handler method if there are no further spring interceptors. If this method returns ‘false’ Spring Framework assumes that request has been handled by the spring interceptor itself and no further processing is needed. We should use response object to send response to the client request in this case. Object handler is the chosen handler object to handle the request. This method can throw Exception also, in that case Spring MVC Handling Exception should be useful to send error page as response.
- postHandle(): This HandlerInterceptor interceptor method is called when HandlerAdapter has invoked the handler but DispatcherServlet is yet to render the view. This method can be used to add additional attribute to the ModelAndView object to be used in the view pages. We can use this spring interceptor method to determine the time taken by handler method to process the client request.
- afterCompletion(): This is a HandlerInterceptor callback method that is called once the handler is executed and view is rendered.
Following is the example on how to use interceptors
@Component
public class ProductServiceInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(
HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
// You can do your processing here
return true;
}
@Override
public void postHandle(
HttpServletRequest request, HttpServletResponse response, Object handler,
ModelAndView modelAndView) throws Exception {}
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response,
Object handler, Exception exception) throws Exception {}
}
You will have to register this Interceptor with InterceptorRegistry by using WebMvcConfigurerAdapter as shown below
@Component
public class ProductServiceInterceptorAppConfig extends WebMvcConfigurerAdapter {
@Autowired
ProductServiceInterceptor productServiceInterceptor;
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(productServiceInterceptor);
}
}
You can find the source code here
https://github.com/Munandermaan/Spring-boot-template
Conclusion
After going through this blog, you can use interceptors in an effective way. Just like adding request header(s) before sending the request to the controller or adding the response header(s) before sending the response to the client.
Reference
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK