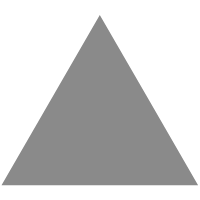
6
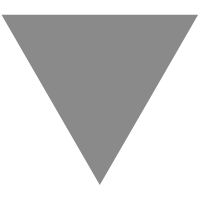
C++中,static 成员函数可以表现出运行时多态性吗?
source link: https://www.zhihu.com/question/440174887/answer/1687656318
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
C++中,static 成员函数可以表现出运行时多态性吗?
登录一下,更多精彩内容等你发现
贡献精彩回答,参与评论互动
C++中内置的运行时多态特性即类的虚函数机制,若静态函数需要表现运行时多态性,得做额外的工作。这也是传说中的值语义多态,无需动态分配内存实现多态效果!
通过union来模拟加法类型Shape
,使得静态函数通过统一的Shape
类型,运行时根据不同的Subtype做出不同的算法:
struct Shape {
enum { Circle, Retangle } type;
union {
struct {
double r;
} circle;
struct {
double w;
double h;
} retangle;
};
};
struct Algorithm {
static double area(const Shape& s) {
switch (s.type) {
case Shape::Circle:
return 3.14 * s.circle.r * s.circle.r;
case Shape::Retangle:
return s.retangle.w * s.retangle.h;
}
}
};
Shape s {
.type = Shape::Circle,
.circle = {2}
};
std::cout << Algorithm::area(s) << std::endl;
s = {
.type = Shape::Retangle,
.retangle = {2, 3}
};
std::cout << Algorithm::area(s) << std::endl;
然而union是非类型安全的,并且无法直接与非平凡类组合,在实际中不那么实用了。可以采用C++17引入的std::variant
来解决这个问题。
struct Circle {
double r;
double area() const {
return 3.14* r * r;
}
};
struct Retangle {
double w;
double h;
double area() const {
return w * h;
}
};
using Shape = std::variant<Circle, Retangle>;
struct Algorithm {
static double area(const Shape& s) {
return std::visit([](const auto& c) {
return c.area();
}, s);
};
};
Shape s = Circle{2};
std::cout << Algorithm::area(s) << std::endl;
s = Retangle{2, 3};
std::cout << Algorithm::area(s) << std::endl;
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK