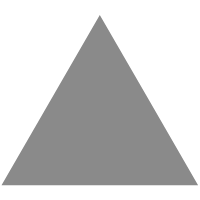
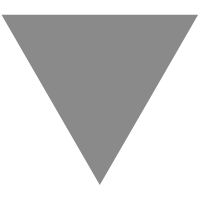
Maximize minimum distance between repetitions from any permutation of the given...
source link: https://www.geeksforgeeks.org/maximize-minimum-distance-between-repetitions-from-any-permutation-of-the-given-array/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Maximize minimum distance between repetitions from any permutation of the given Array
Last Updated: 02-09-2020Given an array arr[], consisting of N positive integers in the range [1, N], the task is to find the largest minimum distance between any consecutive repetition of an element from any permutation of the given array.
Examples:
Input: arr[] = {1, 2, 1, 3}
Output: 3
Explanation: The maximum possible distance between the repetition is 3, from the permutation {1, 2, 3, 1} or {1, 3, 2, 1}.
Input: arr[] = {1, 2, 3, 4}
Output: 0
Approach: Follow the steps below to solve the problem:
- Store the frequency of each array element.
- Find the element which contains the maximum frequency, say maxFreqElement.
- Count the number of occurrences of elements having a maximum frequency, say maxFreqCount.
- Calculate the required distance by the equation (N- maxFreqCount)/( maxFreqElement- 1))
Below is the implementation of the above approach.
- Python3
filter_none
edit
close
play_arrow
link
brightness_4
code
// C++ Program to implement
// the above approach
#include <bits/stdc++.h>
using
namespace
std;
int
findMaxLen(vector<
int
>& a)
{
// Size of the array
int
n = a.size();
// Stores the frequency of
// array elements
int
freq[n + 1];
memset
(freq, 0,
sizeof
freq);
for
(
int
i = 0; i < n; ++i) {
freq[a[i]]++;
}
int
maxFreqElement = INT_MIN;
int
maxFreqCount = 1;
for
(
int
i = 1; i <= n; ++i) {
// Find the highest frequency
// in the array
if
(freq[i] > maxFreqElement) {
maxFreqElement = freq[i];
maxFreqCount = 1;
}
// Increase count of max frequent element
else
if
(freq[i] == maxFreqElement)
maxFreqCount++;
}
int
ans;
// If no repetition is present
if
(maxFreqElement == 1)
ans = 0;
else
{
// Find the maximum distance
ans = ((n - maxFreqCount)
/ (maxFreqElement - 1));
}
// Return the max distance
return
ans;
}
// Driver Code
int
main()
{
vector<
int
> a = { 1, 2, 1, 2 };
cout << findMaxLen(a) << endl;
}
2
Time Complexity: O(N)
Auxiliary Space: O(N)
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready.
If you like GeeksforGeeks and would like to contribute, you can also write an article using contribute.geeksforgeeks.org or mail your article to [email protected]. See your article appearing on the GeeksforGeeks main page and help other Geeks.
Please Improve this article if you find anything incorrect by clicking on the "Improve Article" button below.
Be the First to upvote.
No votes yet.
first_page Minimum Subarray flips required to convert all elements of a Binary Array to K last_page Lead a life problem
Writing code in comment? Please use ide.geeksforgeeks.org, generate link and share the link here.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK