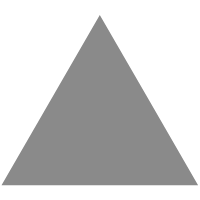
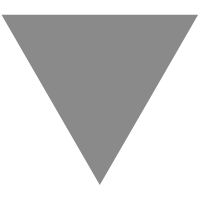
Basics of JavaScript Pure Functions | by Mahdhi Rezvi | Dec, 2020 | Bits and Pie...
source link: https://blog.bitsrc.io/basics-of-javascript-pure-functions-3e6f3437066
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
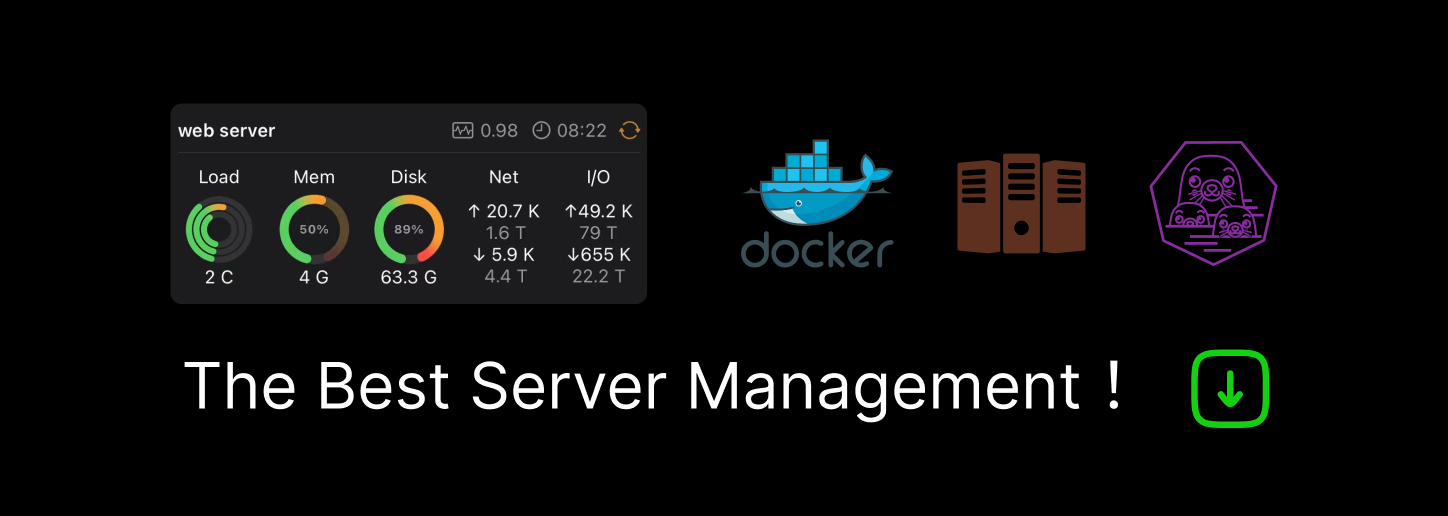
When you grow as a developer, it is essential that you write code that is cleaner and readable. Functional programming is a paradigm that helps you achieve this status. Pure functions are a special type of function that is a core feature of functional programming.
According to JS guru - Eric Elliot,
Functional programming (often abbreviated FP) is the process of building software by composing pure functions, avoiding shared state, mutable data, and side-effects. Functional programming is declarative rather than imperative, and the application state flows through pure functions. Contrast with object-oriented programming, where application state is usually shared and colocated with methods in objects.
In order to understand pure functions, let’s have a closer look at functions.
What is a Function?
Functions are one of the basic building blocks of JavaScript. A typical function accepts inputs also known as arguments, does some processing, and returns an output value. According to Elliot, functions serve several purposes.
- Mapping: The output values are produced based on the input values. A function maps input values to output values.
- Procedures: A function can be called to perform a sequence of steps. The sequence is referred to as a procedure, and programming in this manner is referred to as procedural programming.
- Input/Output(I/O): Some functions provide communications with other parts of the system such as the screen, storage, system logs, network, etc.
Although functions are similar to procedures, for a procedure to qualify as a function, mapping should take place. I strongly believe that functions are a combination of mapping and procedures with I/O as an additional feature as mentioned by Elliot.
What is a Pure Function?
A function that obeys certain conditions will be deemed as a pure function.
- Consistency between arguments and output. Same arguments would always return the same output value, regardless of any external factors.
- No side effects.
Consistency
A pure function will always maintain a relationship where its inputs and output are consistent with one another. A pure function should not depend on any variable outside of its function scope. A pure function must not rely on any external mutable state, because it would no longer be deterministic or referentially transparent. But it is allowed for a pure function to depend on a constant, even outside of the function scope.
Let’s have a look at a function that does not maintain consistency.
Code Snippet by AuthorAs seen above, this function is dependent on a variable that is outside of the function scope. If the variable gets modified, the same input will not provide the same output.
Let’s look at a function that maintains consistency.
The above code snippet can be converted to maintain consistency as shown below.
Code Snippet by AuthorThe deduct
function does not depend on any external variables, rather it depends only on its arguments. You can even use a points
variable as shown in the previous example. But it will still be needed to be passed as an argument to the deduct
function for it to work.
Note
There can be functions that do not receive any inputs and return a value. Although these functions might look like a pure function, most of them are not. If you take a look at the Math.random()
method, it gives a different value every time it’s called. This unpredictable nature disqualifies it as a pure function. Similarly, anything that uses the Date() constructor will also be unpredictable as the constructor returns a different value every moment.
Therefore, a function is only pure if, given the same input, it will always produce the same output.
No Side Effects
Side effects are where your function performs operations that aren’t related to processing the output. In a formal definition, side effects are a change in the program’s state caused by an expression or a function call, or modification of a global variable in a procedure or function.
A few examples of side effects are,
- Mutating your input — modifying your input should only be done after cloning it.
- Modifying a mutable data structure
- console.log — as it prints something on the screen.
- HTTP calls (AJAX/fetch)
- Changing the filesystem (fs)
- Querying the DOM
- Throwing an exception
Immutability is a hot topic these days as it has been often used with React — a popular library. Side effects are mostly avoided in functional programming, which makes the effects of a program much easier to understand, and much easier to test.
Tip: Building components as pure functions can make the difference between a reusable component and a block of code that can only be used once.
You can make the most out of these reusable components using tools like Bit, to share and integrate them into different projects and develop faster.
Components shared on Bit’s component hubWhat is an Impure Function?
Any function that does not fulfill the checklist of pure functions are categorized as impure functions. It is impossible for you to write code without the use of impure functions, but you must try your best to keep it to a minimum. Although it is possible to write a program that maintains consistency between inputs and outputs, impure functions mostly fail in the second requirement in the checklist. Causing side effects cannot be averted in an application code, but it should be avoided as much as possible.
Any example that does not qualify to be a pure function, will automatically be an impure function. The example involving the points
variable will also be considered as an impure function.
Elliot came up with a simpler way to find impure functions.
A dead giveaway that a function is impure is if it makes sense to call it without using its return value. For pure functions, that’s a noop.
Why Should You Use Pure Functions?
There are several reasons why you should use pure functions.
- Makes your function independent and self-sustaining, allowing your programs to be flexible and futureproof.
- Makes unit testing easier as there is no external dependency that will be needed to mock.
- Makes debugging easier as you are certain of what your function would output with a particular input. This makes it easier for you to pinpoint the bug.
- Acts as a basis for functional programming. Therefore would help you implement other functional programming paradigms as well.
- Makes caching easier as the function input and outputs are consistent.
- Enables memorization
- Allows for parallelization as pure functions are independent and self-sustained.
Conclusion
We have gone through the basics of pure functions and how you can write them. You should be able to utilize the power of pure functions to write code that is cleaner, independent, easily movable, readable, and performant.
Thank you for reading & happy coding!
Recommend
-
7
Testable Javascript with pure functions What if I told you that covering javascript code with tests can be easy and pleasant experience? There’s one simple rule you need to follow in order to achieve that:
-
8
Is JavaScript Becoming TypeScript?What might the future hold in store?...
-
7
Duck Typing in JavaScript and TypeScriptDuck Typing for beginnersPhoto by Anne Nygård on
-
16
Namespace in JavaScript — The BasicsOrganized meaningful code...
-
8
Import Assertions in JavaScriptEasier Non-JavaScript Artifact Imports
-
15
JavaScript Typed ArraysAn Introduction to Typed Arrays in JavaScript
-
6
Design Patterns in JavaScriptCommonly Used Design Patterns With Code ExamplesPhoto by Andrew Ridley on
-
6
Introduction to JavaScript Functions A function allows us to place code logically to execute a task. Functions are first-class citizens in the JavaScript programming language. You can create, modify a function, u...
-
6
Pure and Impure Functions in JavaScript: A Complete GuidePure functions and impure functions are two common terms used in JavaScript. On the surface level, both functions look identical.However, if we loo...
-
10
Contents Inspiration About two years ago I created a Webpack setup to suit my specific needs when developing with react. Since then I've mainly been on large projects and beca...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK