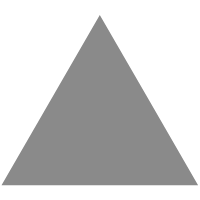
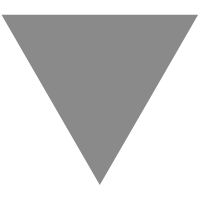
Namespace in JavaScript — The Basics | by Mahdhi Rezvi | Bits and Pieces
source link: https://blog.bitsrc.io/namespace-in-javascript-the-basics-452e6e8adc48
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
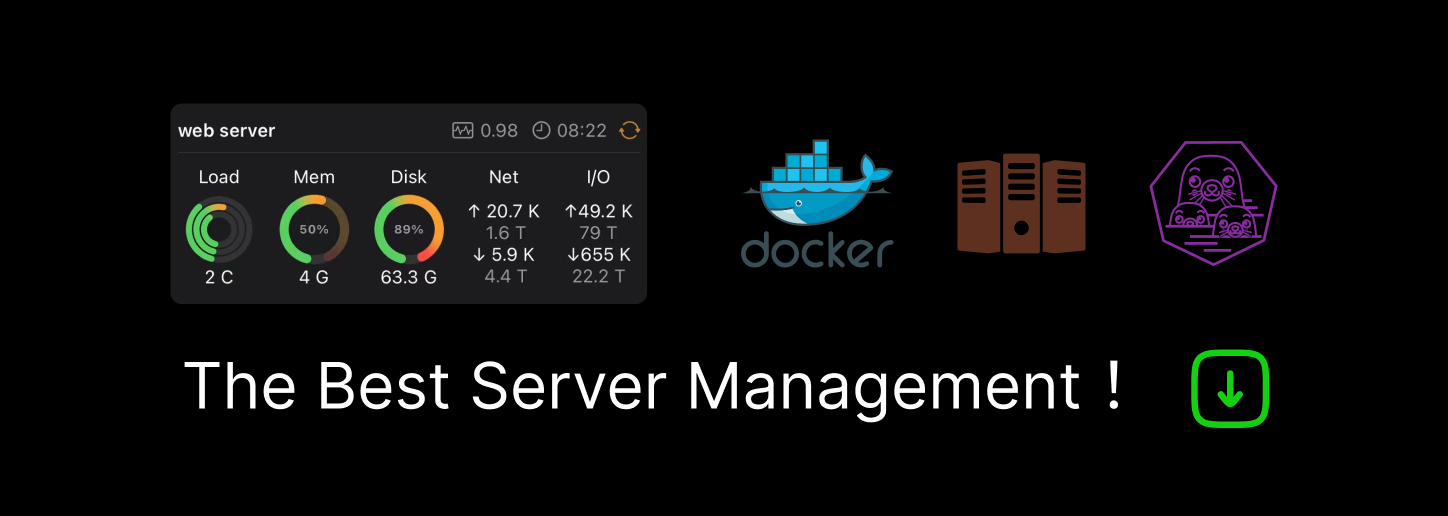
Once you start building complex applications, there are several factors that will you start to take into consideration. One of those factors is code organization. Although you might not realize the value of code organization when your applications are small in size, when your applications become complex, you will know the value of organized code.
One of the techniques in achieving code organization is called namespacing. Namespaces also help you to avoid collisions with other objects or variables in the global namespace. This is a common problem when working with multiple developers and libraries.
Namespace in JavaScript
Namespacing is essential in an enterprise-level application as you will be integrating several 3rd party scripts that might end up using the same variable or method names as yours. This will lead your code to break as your variables and methods will get overwritten.
It must be noted that JavaScript does not support namespace out of the box, unlike several other programming languages such as C++. But due to the importance of this feature, developers use namespacing patterns to achieve namespacing in JavaScript with the help of objects and closures.
This article discusses only the basic namespacing patterns in JavaScript. For more intermediate and advanced patterns, I would recommend you read Addy Osmani’s book, Learning JavaScript Design Patterns.
Let’s have a look at these patterns.
Single Global Variables
This is one of the most basic and commonly used approaches. This approach uses a single global variable as your point of reference. You will access your methods and variables as properties of this object.
Code SnippetThe above code snippet returns an object with references to the functions from an IIFE.
One common problem of the above pattern is to make sure that no one else uses the same global variable as ours. If someone else also uses the same global variable in their script, our global variable would simply get overwritten.
The next pattern provides a solution to this challenge.
The single global variable pattern can also cause problems by the improper use of thethis
keyword.
You can use the call
function to call the sayHello
function with the appropriate value for this
as shown below.
Prefix Namespacing
As a solution to the above problem, Peter Michaux introduced a concept called Prefix Namespacing. This is quite a simple concept which is an extended version of the single global variable pattern. In the prefix namespacing pattern, we use the unique single global variable as before, but with an underscore followed by the method or property name. This would end up with not one, but multiple global variables which are named as sampleApplication_value
, sampleApplication_add()
, sampleApplication_minus()
in accordance with the previous example.
Prefix namespacing is pretty much used in C as well. It can also be found in JavaScript under Macromedia’s MM_
functions.
One of the issues with this pattern is that you will end up with several global variables when your application grows in size. This might throw problems in earlier versions of some browsers as they perform poorly with a large number of global variables.
Object Literal Notation
Object Literal Notion can be considered as a simple object containing a collection of key-value pairs. These key-value pairs can themselves contain new namespaces. This would allow you to organize your code logically without polluting the global environment. Their clean syntax is easily understandable and provides a foundation for namespaces to be extended with ease.
Code SnippetYou can also directly assign these key-value pairs by directly accessing the sampleApplication
object.
You must also make sure that the global variable hasn’t been defined elsewhere. If it has been defined, make sure to use that variable. If not, initialize the variable to an empty object.
Most developers use either one of the below methods to verify whether a variable exists in the global scope.
Code SnippetOne disadvantage of this approach is that the object syntax tends to grow longer when your application grows in size.
Tip: Share your components between projects using Bit.
Use Bit to author and share independent components that can be reused across projects.
With Bit, every component can be independently maintained and developed so that you don’t need to set up a full dev environment just to make a few changes.
Bit (Github) supports Node, TypeScript, React, Vue, Angular, and more.
Exploring shared component on Bit.devNested Namespacing
The Nested Namespacing pattern is an extended version of the Object Literal Notation pattern. This can help your namespaces to contain several namespaces nested within themselves, and thereby keeping your code organized logically.
As seen in the previous patterns as well, you should make sure your variables are initialized before accessing them. It should also be noted that a level 2 namespace should be initialized in order for a level 3 namespace to be defined or initialized. If not, you will receive a type error cannot set property of undefined
.
You can also define values by using the bracket property accessor notation. This notation allows you to use variables to access object properties.
Code SnippetYou might wonder whether accessing deeply nested objects can affect your application performance. For example, to access a function belonging to a namespace nested several levels below will take more time than accessing a function at level 1. But according to Addy, developers such as Juriy Zaytsev have tested and found out that this difference is negligible.
Immediately-Invoked Function Expressions(IIFEs)
An Immediately-Invoked Function Expression is a JavaScript function that runs as soon as it is defined. IIFEs have several uses including private properties and methods, aliasing, and more.
You can read more about IIFEs in my article over here.
Another important use of IIFEs is that they provide namespacing as well. We are able to pass the namespace object as a parameter to the IIFE and assign public properties to the namespace object as shown below. You can also expand this example to contain public/private properties for the namespace.
Code SnippetFurthermore, you can easily add additional properties/functions to your namespaces by following this pattern as well. All you have to do is pass an existing namespace as the parameter to IIFE and add public properties to it.
Namespace Injection
This pattern is the extension of the IIFE pattern. In this pattern, we “inject” the methods and properties for a specific namespace from within a function wrapper using this
as a namespace proxy.
This pattern makes it easier to apply functional behavior to multiple namespaces and even apply base methods such as getters and setters and toString which can be built on later.
But there can be easier, more optimal approaches to achieving the same goal of deep namespace extension as we have discussed before.
Code SnippetNamespace has been one of the most expected JS features that have not yet been supported out of the box. But with the availability of objects and closures in JavaScript, we were able to replicate the aim of namespaces. Although ES6 modules have made namespaces almost obsolete, you still need to know them to work with older applications and unsupported browsers.
This article is only an introduction to JS namespaces as I have only discussed the basic namespacing patterns as per Addy’s book. I would highly recommend you to go through the below-mentioned resources to get an overall understanding of namespaces in JavaScript.
Learn More
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK