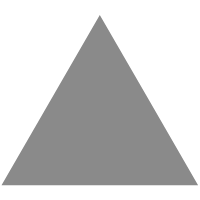
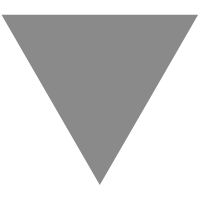
Duck Typing in JavaScript and TypeScript | by Mahdhi Rezvi | Mar, 2021 | Bits an...
source link: https://blog.bitsrc.io/duck-typing-in-javascript-and-typescript-7cc834fadd64
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
JavaScript has come a long way since its birth in the 90s. Major improvements have been incorporated with it along its journey. It should be noted JavaScript started out and is still not an OOP-based language. JavaScript initially did not contain “class” related syntax and inheritance was based on prototypes. Later with the release of ES6, JavaScript introduced the “class” based syntax to provide familiarity to OOP-based developers. But the problem was that these so-called “classes” were still not pure classes. They followed the previous prototypal inheritance and just contained the new syntactic sugar.
Since JavaScript was implementing this new syntactic sugar-based OOP approach, it was unable to provide certain prominent features of OOP languages. We will be discussing one such missing feature and its solution today. We will also be discussing this concept being implemented in TypeScript as well.
What is Duck Typing?
Duck Typing is an implementation of the Duck Test concept. The Duck Test is a form of abductive reasoning which usually denotes the statement “If it walks like a duck and it quacks like a duck, then it must be a duck”. Abductive reasoning is a form of logical inference that seeks to find the simplest and most likely conclusion from the observations. You can read more about abductive reasoning over here.
The above paragraph might sound like Greek for most of you. Let me explain it in much simpler terms. Duck Typing is a concept where you use the below-given logic to determine whether an object can be used for a particular purpose.
“If it walks like a duck and it quacks like a duck, then it must be a duck”
Rather than comparing the object itself, we compare the methods and properties present in both objects and determine whether it is a “duck” or whatever object.
What is an Interface?
An Interface is a programming concept found in OOP languages. In Object-Oriented Programming, an Interface is a description of all functions that an object must have in order to be considered an object of type “X”. Interface defines a standard that implementations must comply with. The purpose of interfaces is to allow the computer to enforce these properties and to know that an object of type X must have functions called A, B, C.
You can read more about interfaces over here.
Duck Typing and Interfaces
If you have understood the above topics clearly, you would have deduced something by now. It is that interfaces and duck typing are somewhat similar to one another. In fact, duck typing is the way of implementing the concept of Interfaces in JavaScript.
As I have mentioned previously, although JavaScript supports the “class” syntax, these classes are not pure classes. This leads to a problem of implementing the concept of Interfaces in JavaScript as it uses the prototypal inheritance approach. To rectify this, developers use the concept of duck typing to provide interfaces to JavaScript.
You will get a better understanding of these concepts once we go through the examples.
Duck Typing in JavaScript
Since we are working with Duck Typing, let’s use an example that uses ducks. We have a Duck class that contains two methods — walk and quack.
Code snippet by AuthorWe have another class named Platypus that also contains two methods — walk and growl.
Code snippet by AuthorAccording to the Duck Typing concept, we can conclude that Platypus does not belong to the Duck type. This is because the class Platypus does not contain the method quack found in the class Duck. According to the Duck Typing concept, object B should contain the methods found in object A to be deemed as a type of object A.
Let’s take another class, Pelican which contains three methods — walk, quack and dive.
Code snippet by AuthorIn this scenario, we can conclude that Pelican is of Duck type as it contains the methods walk and quack found in the Duck class. You might also note that the Pelican class has one additional method which cannot be found in the Duck class. This does not cause any problems according to the Duck Type concept. This is also the usual implementation with interfaces as well.
We can use the below piece of code to identify whether an object is of type Duck.
Code snippet by AuthorCode snippet by AuthorDuck Typing in TypeScript
When we consider TypeScript, it is an open-source programming language developed by Microsoft that compiles into JavaScript. Since its release in 2012, the language has remained in active development and continues to gain in popularity every year.
TypeScript has the feature to be statically typed, which can be turned off if required. TypeScript provides several other features not present natively in JavaScript and one such feature would be Interfaces. One of TypeScript’s core principles is that type checking focuses on the shape that values have. This is quite similar to the Duck Typing principle we saw above. This approach can also be referred to as structural subtyping.
Let’s discuss the same example we used with JavaScript, but this time, we’ll use interfaces.
We will be looking at our Duck class first. Since TypeScript supports Interfaces, we will be using their help as well.
Code snippet by AuthorLet’s have a look at our Pelican class which implements an interface as well.
Code snippet by AuthorAnd finally our Platypus class, but we have refrained from using an interface. This is just to keep the example try out some possibilities of variations.
Code snippet by AuthorSince TS is statically checked, we will not have to implement our own checking mechanism like our JS example. We will be using a print()
function that expects an input of type IDuck
.
Once you run the example code, you will realize that the print
function accepts the object pelican
as an argument, even though it is of a different class implementing a totally different interface. Regardless of the interface or class used, all the properties(methods) found in the duck
object can also be found in the platypus
object as well. Hence TS accepts this as an argument. This highlights the use of Duck Typing in TS as noted before.
When you try to input the object platypus
to the print
function, you will receive an error as below.
Argument of type 'Platypus' is not assignable to parameter of type 'IDuck'. Property 'quack' is missing in type 'Platypus' but required in type 'IDuck'.
This error pretty much explains the implementation of duck typing in this scenario. The platypus
object does not contain the quack
method found in Duck. Hence the error.
You should also note that the example only uses methods to showcase Duck Typing. You can expand this example to check for class properties as well. It will work similarly to the concept we’ve discussed.
The full code for the TS version of the example can be found below.
Code snippet by AuthorIt is essential that we understand the core implementations of the languages we work on. We discussed the implementation of Duck Typing in JS and TS in this article.
Thank you for reading and happy coding.
Build & share independent components with Bit
Bit is an ultra-extensible tool that lets you create truly modular applicationswith independently authored, versioned, and maintained components.
Use it to build modular apps & design systems, author and deliver micro frontends, or simply share components between applications.
Material UI components shared individually on Bit.devRecommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK