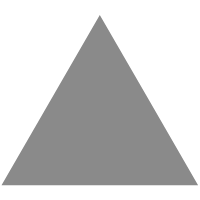
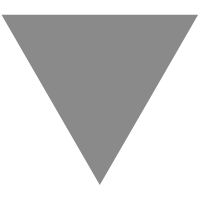
Replace NaN Values with Zeros in Pandas DataFrame - GeeksforGeeks
source link: https://www.geeksforgeeks.org/replace-nan-values-with-zeros-in-pandas-dataframe/?ref=leftbar-rightbar
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
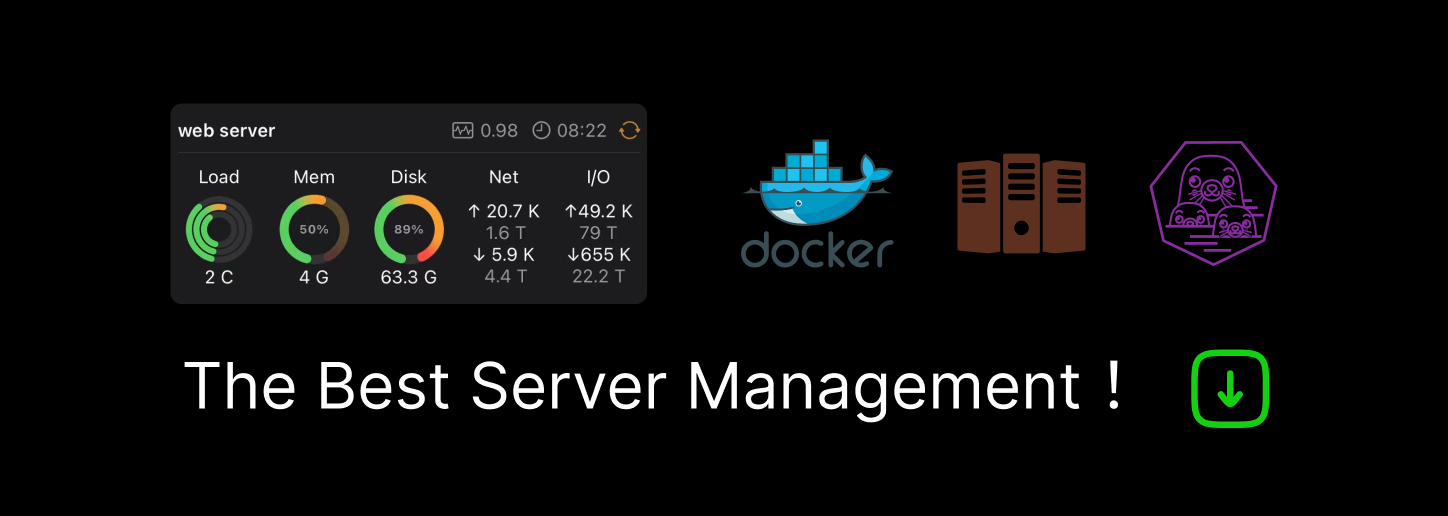
Replace NaN Values with Zeros in Pandas DataFrame
Last Updated: 03-07-2020NaN stands for Not A Number and is one of the common ways to represent the missing value in the data. It is a special floating-point value and cannot be converted to any other type than float. NaN value is one of the major problems in Data Analysis. It is very essential to deal with NaN in order to get the desired results.
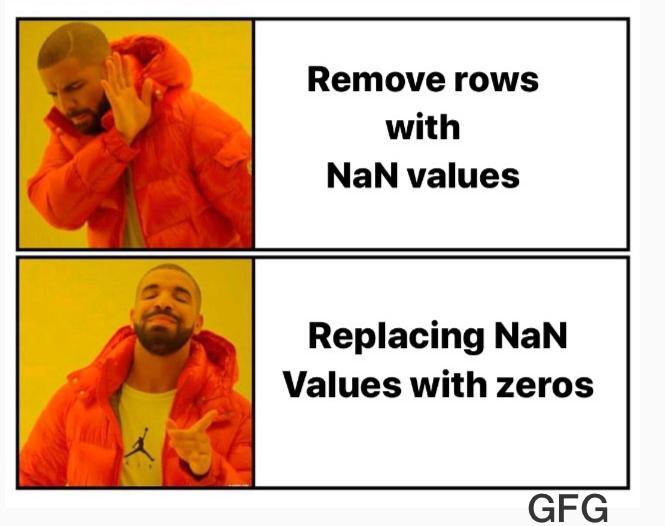
Methods to replace NaN values with zeros in Pandas DataFrame:
- fillna()
Thefillna()
function is used to fill NA/NaN values using the specified method. - replace()
Thedataframe.replace()
function in Pandas can be defined as a simple method used to replace a string, regex, list, dictionary etc. in a DataFrame.
Steps to replace NaN values:
- For one column using pandas:
df['DataFrame Column'] = df['DataFrame Column'].fillna(0)
- For one column using numpy:
df['DataFrame Column'] = df['DataFrame Column'].replace(np.nan, 0)
- For the whole DataFrame using pandas:
df.fillna(0)
- For the whole DataFrame using numpy:
df.replace(np.nan, 0)
Method 1: Using fillna() function for a single column
Example:
filter_none
edit
close
play_arrow
link
brightness_4
code
# importing libraries
import
pandas as pd
import
numpy as np
nums
=
{
'Set_of_Numbers'
: [
2
,
3
,
5
,
7
,
11
,
13
,
np.nan,
19
,
23
, np.nan]}
# Create the dataframe
df
=
pd.DataFrame(nums, columns
=
[
'Set_of_Numbers'
])
# Apply the function
df[
'Set_of_Numbers'
]
=
df[
'Set_of_Numbers'
].fillna(
0
)
# print the DataFrame
df
Output:
Method 2: Using replace() function for a single column
Example:
filter_none
edit
close
play_arrow
link
brightness_4
code
# importing libraries
import
pandas as pd
import
numpy as np
nums
=
{
'Car Model Number'
: [
223
, np.nan,
237
,
195
, np.nan,
575
,
110
,
313
, np.nan,
190
,
143
,
np.nan],
'Engine Number'
: [
4511
, np.nan,
7570
,
1565
,
1450
,
3786
,
2995
,
5345
,
7777
,
2323
,
2785
,
1120
]}
# Create the dataframe
df
=
pd.DataFrame(nums, columns
=
[
'Car Model Number'
])
# Apply the function
df[
'Car Model Number'
]
=
df[
'Car Model Number'
].replace(np.nan,
0
)
# print the DataFrame
df
Output:
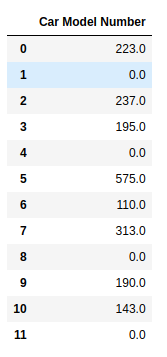
Method 3: Using fillna() function for the whole dataframe
Example:
filter_none
edit
close
play_arrow
link
brightness_4
code
# importing libraries
import
pandas as pd
import
numpy as np
nums
=
{
'Number_set_1'
: [
0
,
1
,
1
,
2
,
3
,
5
, np.nan,
13
,
21
, np.nan],
'Number_set_2'
: [
3
,
7
, np.nan,
23
,
31
,
41
,
np.nan,
59
,
67
, np.nan],
'Number_set_3'
: [
2
,
3
,
5
, np.nan,
11
,
13
,
17
,
19
,
23
, np.nan]}
# Create the dataframe
df
=
pd.DataFrame(nums)
# Apply the function
df
=
df.fillna(
0
)
# print the DataFrame
df
Output:
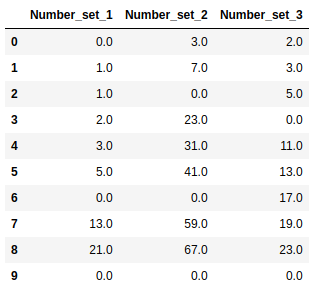
Method 4: Using replace() function for the whole dataframe
Example:
filter_none
edit
close
play_arrow
link
brightness_4
code
# importing libraries
import
pandas as pd
import
numpy as np
nums
=
{
'Student Name'
: [
'Shrek'
,
'Shivansh'
,
'Ishdeep'
,
'Siddharth'
,
'Nakul'
,
'Prakhar'
,
'Yash'
,
'Srikar'
,
'Kaustubh'
,
'Aditya'
,
'Manav'
,
'Dubey'
],
'Roll No.'
: [
18229
,
18232
, np.nan,
18247
,
18136
,
np.nan,
18283
,
18310
,
18102
,
18012
,
18121
,
18168
],
'Subject ID'
: [
204
, np.nan,
201
,
105
, np.nan,
204
,
101
,
101
, np.nan,
165
,
715
, np.nan],
'Grade Point'
: [
9
, np.nan,
7
, np.nan,
8
,
7
,
9
,
10
,
np.nan,
9
,
6
,
8
]}
# Create the dataframe
df
=
pd.DataFrame(nums)
# Apply the function
df
=
df.replace(np.nan,
0
)
# print the DataFrame
df
Output:
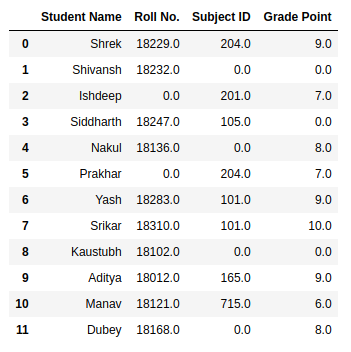
Attention geek! Strengthen your foundations with the Python Programming Foundation Course and learn the basics.
To begin with, your interview preparations Enhance your Data Structures concepts with the Python DS Course.
If you like GeeksforGeeks and would like to contribute, you can also write an article using contribute.geeksforgeeks.org or mail your article to [email protected]. See your article appearing on the GeeksforGeeks main page and help other Geeks.
Please Improve this article if you find anything incorrect by clicking on the "Improve Article" button below.
7
No votes yet.
Recommend
-
59
In this article we will discuss how to sort rows in ascending and descending order based on values in a single or multiple columns . Also, how to sort columns based on values in rows using DataFrame.sort_values()
-
12
How to drop rows in Pandas DataFrame by index labels? Last Updated: 02-07-2020 Pandas provide data analysts a way to delete and filter data frame using
-
14
How to create an empty DataFrame and append rows & columns to it in Pandas? ...
-
4
Count Unique Values in all Columns of Pandas Dataframe – thisPointer.comSkip to content This article will discuss different ways to...
-
122
Count number of Zeros in Pandas Dataframe Column This article will discuss how to count the number of zeros in a single or all column of a Pandas Dataframe. Let’s first create a Dataframe from a list of...
-
10
Pandas | Count non-zero values in Dataframe Column This article will discuss how to count the number of non-zero values in one or more Dataframe columns in Pandas. Let’s first create a Dataframe from a...
-
15
Pandas – Count True Values in a Dataframe Column In this article, we will discuss different ways to count True values in a Dataframe Column. First of all, we will create a Dataframe from a list of tuples...
-
5
Replace Header With First Row In Pandas Dataframe – thisPointer This article will discuss how to replace the header with the first row in Pandas DataFrame. A DataFrame is a data structure that stores the data in rows and column...
-
11
Pandas: Check if all values in column are zeros This article will discuss checking if all values in a DataFrame column are zero (0) or not. First of all, we will create a DataFrame from a list of tuples,
-
7
Replace NaN Values with Zeros in Pandas DataFrameReplace NaN Values with Zeros in Pandas DataFrame10 Views06/06/2022In this video, we are...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK