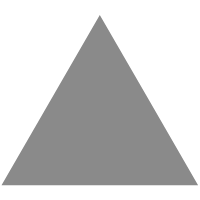
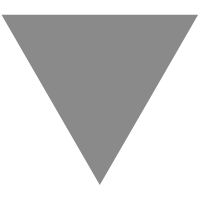
How to use GIT to increase productivity
source link: https://www.ashishjha.com/technology/devops/how-to-use-git-to-increase-productivity.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.

We as a developer know the importance of GIT in our day to day life. Many of us use GIT for development but most of us never think beyond commit, push and pull. So, the main question is – Are we using GIT to its full potential? Are we thinking beyond GIT PUSH, PULL & COMMIT? In this article, we will see how can we become a pro in using GIT and make most out of GIT so that we can make our life as a developer more productive.
What is Git and how it works?
GIT is a system to track changes in the source code that happens during the software development life cycle and helps multiple developers collaborate in a project. Git is a free and open-source distributed version control system designed to handle small to very large projects with speed and efficiency. When we say distributed, we mean that GIT repository is not in any single central location it is distributed across the people on the project. There is however a central remote repository location that keeps track of all the distributed local repositories. The image below will explain how GIT works?
Pro-tip: Its always recommended to use command-line utilities like GIT bash for performing GIT operations. Itis quick and easy and allows you to work on any platform without any hiccups.
Create a Local Repository
First step in using GIT is either to initialize a git folder on your local computer or clone already existent git repository on your local computer.
Creating a local repository from scratch//When you have already created the directory which you plan to use $ git init Initialized empty Git repository in D:/GIT/created-folder/.git/ //When you plan to create the directory for you using the command directory $ git init create-directory Initialized empty Git repository in D:/GIT/create-directory/.git/Clone already existent repository
//When you have already created the directory which you plan to use $ git init Initialized empty Git repository in D:/GIT/created-folder/.git/ //When you plan to create the directory for you using the command directory $ git init create-directory Initialized empty Git repository in D:/GIT/create-directory/.git/
Useful GIT Operations
Once you have created the local repository, its time to start working. Let’s us go the important commands needed to start working with GIT
GIT Config Command
Every GIT repository has certain configurations associated with it. Although, most of the configurations will not be needed except two. Whenever you do any edits or commits, your username and email is logged along with the modification done. For this to happen, its important that your username and email is properly updated in the GIT repository. Below are the set of command examples explaining how to list all git config’s and how to update git username and email and verifying whether the configurations updated or not. Commands are listed below:
//This command lists all the configurations available for the GIT repository. Username and email is most important configuration which needs checking $ git config --list core.symlinks=false core.autocrlf=true core.fscache=true color.diff=auto color.status=auto color.branch=auto color.interactive=true help.format=html rebase.autosquash=true http.sslcainfo=C:/Program Files/Git/mingw64/ssl/certs/ca-bundle.crt http.sslbackend=openssl diff.astextplain.textconv=astextplain filter.lfs.clean=git-lfs clean -- %f filter.lfs.smudge=git-lfs smudge -- %f filter.lfs.process=git-lfs filter-process filter.lfs.required=true credential.helper=manager user.name={name} user.email={email} credential.helper=manager alias.decorator=log --oneline --graph --decorate gui.recentrepo=D:/GIT/aws/test core.repositoryformatversion=0 //Updating configuration git config --global user.name "Ashish" //checking the config value git config user.name
GIT Status Command
Git status is something that will tell you the current status of your local git repository and is one of the most used git commands. Git status tells you about the current status of the repository you are working on like what changes are done, changes to be committed, etc.
$ git status On branch master Your branch is up to date with 'origin/master'. Changes not staged for commit: (use "git add <file>..." to update what will be committed) (use "git checkout -- <file>..." to discard changes in working directory) modified: Algorithms/Search_Algorithms/Binary_Search/binarySearch.js modified: Algorithms/Sorting_Algorithms/Quick_Sort/QuickSort.py modified: Algorithms/Sorting_Algorithms/Quick_Sort/quicksort.C no changes added to commit (use "git add" and/or "git commit -a")
GIT Add Command
Once you have created/cloned the repository, you can add files to the repository and start working on them. After done working on the files, lets add the files to the repository. If the files are added to the local repository for the first time, it has to be added to GIt to get it tracked.
//you can use git status command to check the status of the repository to know whether files need to get added or not // If all the files has to be added git add . //If single files has to be added, use the below format git add filename
Renaming & Deleting files in GIT
Once the files are added to the repository, it starts getting tracked. There are times when you want the tracked files to be renamed and deleted. GIT tracked files are generally deleted using and renamed using GIT command listed below.
//rename already tracked file git mv filename newfilename // ex: git mv readme2.html readnew.html //git delete existing files git rm filename //git rm readme2.html
GIT Commit Command
Any change that you do in your local working repository should be committed in order to move it to the local repository. You can refer to the above image to get an idea. GIT commit command is listed below
// every git commit requires a message so that later we can refer to messages to know more about commits git commit -m "message"
GIT Reset Command
Referring to the image above it’s clear that files go to staging area once its GIT added. If the files are staged, it means that its ready for next commit. There might be times when you want the staged files to be removed from staging so that it does not get committed and finally pushed to remote directory. This can be done using GIT reset command which is listed below
git reset --soft git reset --hard
GIT Diff Command
GIT diff command is used see the changes in your local file when compared with the files present in remote repository. This can be done using GIT diff command which is listed below
// GIT DIFF to check happened in the local repository git diff git diff filename
GIT Blame Command
Suppose you want to see all the changes done in a particular file and other details like what was changed, who changed in and when was it changed. How would you do that? This can be done using GIT blame command which is listed below
// seeing all the details of changes in a particular file using blame command git blame filename
GIT Show Command
Suppose you want to see all the changes done in a particular commit and other details like what was changed, who changed in and when was it changed. How would you do that? This can be done using GIT blame command which is listed below
// showing changes in a particular commit git show commit_id
GIT Log Command
Now since you have started using GIT actively, at some point of time there might be a need to now what kind of changes has happened in the repository. How would you do that? This can be done using GIT log command which is listed below
// checking logs of entire repository git log
Working with GIT Branches
In GIT we work with branches. Whenever we clone a repository, we infact clone a branch of repository. To know which branch we are working on we can use below commands
git branch git branch -r git branch -av git branch new_branch git branch -d new_branch
GIT Checkout Command
git add . git add filename git commit -m "message"
GIT tag Command
git add . git add filename git commit -m "message"
GIT Merge Command
Finally, once we have completed our feature development in your new branch, we will need to merge it back into the main branch. So first of all go to the main branch you want to merge your feature branch into. In this case we’ll run git checkout master. Next we call the merge command passing in the branch which we want to merge into our active branch. So we run git merge feature/feature_name.
git merge branch-to-merge
Synchronize with Remote Repository
GIT Fetch Command
GIT fetch basically fetches latest changes from the remote repository and add it to the local repository, but it does not update your working copy. This can be done using GIT fetch command explained below.
//fetching the recent changes from remote repository without merging git fetch
GIT Pull Command
GIT pull basically runs 2 commands in one i.e. git fetch + git merge. Git pull basically fetches latest changes from the remote repository and add it to the local repository, along with that it updates my working copy also. GIT pull command is explained below.
//syncing local repository and working with remote repository git pull
GIT Push Command
Now that we have worked on our codes and committed it to local repository, we would want this code to be accessible to other developers in the team or want this code to be the part of main project. For this we will have to push all our code modifications to remote repository which we can do using GIT push command explained below:
//Sending local code modifications to remote repository git push
Bonus Tips
Using GIT Alias command
GIT alias as the name suggest serves as an alias for any long command. Suppose there are cases when you have to command with long parameters every now and then, it becmes very problematic to type complete command and parameters again and again. What you can do is that you can create an alias for that command and from next time instead of typing complete command just type the alias. In this case first you will have to create an alias and then after its created you can start using the alias. Example is given below:
//create the alias first git config --global alias.decorator "log --oneline --graph --decorate" //Use alias now git decorator
Using Gitignore
Guys, we have seen cases that we start coding and running the file to test it or debug it, multiple temp files or cache or compiled files are created in the directory. Now obviously we dont want to add those files to the central remote repository. But whenever we do a commit, git will always prompt that there are untracked files in the directry, which might create confusion for a developer at some point of time. What to do in this case? Solution is to create a .gitignore file in root directory. gitignore is a git system file which you can create in directory and whatever file name or pattern you mention in the gitignore file will be ignored by git for tracking.
Incase you are interested in knowing how gitignore looks like or want a predefined gitignore file for your project , you can download it from the link below: This link contains sample gitignore file for most of the programming languages.
Using GIT Stash
Lets take an example that we are working on a codebase and we are in between a release of a new feature. In the meantime, something goes wrong and code stops working or someone from the team asks for the code files of last working version. You would want to share the old cleaner and working versin without losing the current work. In this scenario, we use GIT stash. Use git stash
when you want to record the current state of the working directory and the index, but want to go back to a clean working directory. The command saves your local modifications away and reverts the working directory to match the HEAD
commit.
git stash list git stash pop
Now that you have gone through the article, you can start implementing in your workflows. I will keep updating this article with more examples and tips in times to come, so do share the article with your friends who might hep from this. If you have any queries, you can comment below If you have any tips/tricks about GIT, do share them in the comment below and i will add them in the article for sure.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK