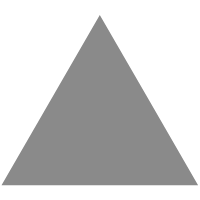
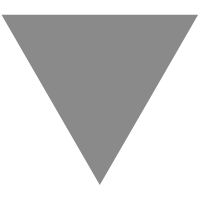
SpringBoot魔法堂:说说带智能提示的spring-boot-starter
source link: https://segmentfault.com/a/1190000038136847
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
前言
前几个月和隔壁组的老王闲聊,他说项目的供应商离职率居高不下,最近还有开发刚接手ESB订阅发布接口才两周就提出离职,而他能做的就只有苦笑和默默地接过这个烂摊子了。
而然幸福的家庭总是相似的,而不幸的我却因业务变革走上了和老王一样的道路。单单是接口的开发居然能迫使一位开发毅然决然地离职,我既不相信是人性的扭曲,更不信是道德的沦丧。
抛开这个富有色彩的故事而言,我发现原来的项目存在如下问题:
- 没有使用任何现代依赖管理和构建工具(如Maven, Gradle),直接把所依赖的Jar包存放在项目目录下的lib目录中,日积月累导致lib目录下存放大量无用Jar包;
- 没有使用代码版本管理工具管理代码;
- 技术文档欠缺,全靠师傅带徒弟的方式传授框架使用方式和开发流程;
- 机械性配置项多,而后来的开发人员大多只能依葫芦画瓢添加配置,既容易出错同时又增加问题排查的难度。
针对前两个问题,我们只需梳理出必须的依赖项并加入Maven或Gradle管理,然后托管到Git即可。
而后两者则可以通过spring-boot-starter将必选依赖项和配置统一管理,并附上相关技术文档;然后通过模板模式和注解简化开发流程,提供Demo降低入门难度。
最后就可以把具体的业务功能开发交给供应商处理,我们专心做好过程管理和验收即可。
本文将着重分享spring-boot-starter开发的事项,请坐好扶稳!
命名规范
在自定义starter前我们总要思考如何命名我们的starter,而官方提供如下的命名规范:
-
官方的starter以spring-boot-starter作为前缀命名项目
如:spring-boot-starter-web -
非官方的则以spring-boot-starter作为后缀命名项目
如:mybatis-spring-boot-starter
项目结构
通过Spring Initializr或Spring Boot CLI创建项目结构后,将pom.xml的相关项目修改为如下内容
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifacId> <version>2.3.1.RELEASE</version> <relativePath/> </parent> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <!-- 下面为自定义Starter的依赖项 --> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-source-plugin</artifactId> <version>2.4</version> <executions> <execution> <goals> <goal>jar</goal> </goals> </execution> </executions> </plugin> </plugins> </build>
在starter中我们会定义SpringBean的注册配置和属性配置,如ESB订阅服务的配置项目为
@Configuration @EnableConfigurationProperties({EsbServerProperties.class}) public class EsbServerConfiguration { @Bean public SpringBus springBus(){ return new SpringBus(); } @Bean public LoggingFeature loggingFeature(){ return new LoggingFeature(); } @Bean public List<JMSConfigFeature> jmsConfigFeatures(EsbServerProperties props) throws JMSException { List<JMSConfigFeature> features = new ArrayList<>(); /** * 这里会使用EsbServerProperties的属性构建Bean实例 */ return features; } }
属性配置项
// 从application.yml等配置文件中读取并绑定esb.server.destination等属性值 @Data @ConfigurationProperties("esb.server") public class EsbServerProperties { String destination; int currConsumers = 1; String channel; int ccsid = 1205; int transportType = 1; List<String> connectionNameLists; boolean replyError = false; String replySuccessText = "Success"; String replyErrorText = "Failure"; }
到这里我们已经完成一个基本的starter的功能
@ConfigurationProperties @Configuration
但引用该starter的项目要如何启用配置呢?其实有两种方式,分别为手动和自动,其中我们会着重讲解自动启用配置。
手动启用配置
所谓手动启用配置其实就是在SpringBoot入口类上添加启用配置用的自定义注解,针对上面的EsbServerConfiguration我们可以自定义EnableESBSrv注解
@Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) @Documented @Import({EsbServerConfiguration.class}) public @interface EnableEsbSrv { }
然后入口类的 @SpringBootApplication
注解前后添加 @EnableEsbSrv
即可。
让人省心省力的自动启用配置
自动启用配置即只需在pom.xml中引入所依赖的starter,然后启用应用即可自动启用该starter的 @Configuration
所注解的类从而注册Bean和读取属性配置。
而这一切都是由 AutoConfigurationImportSelector
来操刀,而我们可以通过 @EnableAutoConfiguration
或 @SpringBootApplication
等实例化 AutoConfigurationImportSelector
类,配合菜谱resources/META-INF/spring.factories实现自动化配置的功能。
具体手法就是:将EsbServerConfiguration的全限类名称写在resources/META-INF/spring.factories的 org.springframework.boot.autoconfigure.EnableAutoConfiguration
下, 若存在多个则用逗号分隔。
org.springframework.boot.autoconfigure.EnableAutoConfiguration = \ com.john.starter.EsbServerConfiguration,\ com.john.starter.OtherConfiguration
好与更好——集成IDE智能提示
应用启动时会将application.yml中对应的配置项绑定到 @ConfigurationProperties
标注的类实例上,那么对于应用开发人员而言日常工作就是修改application.yml的配置项。但IDE又缺少配置项的智能提示,那就很低效了。幸亏Spring Boot早就为我们提供好解决方案,分为手工和自动两种。为了效率当然是可以自动就不用手动的了。
Starter项目的工作
- 引入spring-boot-configuration-processor依赖项;
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency>
mvn compile
业务系统项目的工作
- 引入spring-boot-configuration-processor依赖项;
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency>
- IDEA安装Spring Assistant插件,并启用Enable annotation processing(勾选 Settings/Build, Execution & Deployment/Compiles/Annotation Processors/Enable annotation processing)。
总结
spring-boot-starter非常适合用于团队的技术积累和沉淀,不过想恰到好处地应用起来,不仅要需要深入Spring内部原理还要梳理清楚业务逻辑。后续我们再深入探讨Spring内核的事情吧!
转载请注明来自: https://www.cnblogs.com/fsjoh... —— ^_^肥仔John
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK