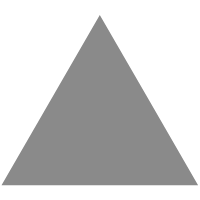
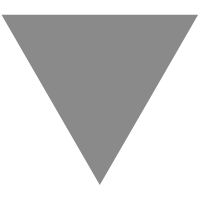
Working with JSON data in Oracle databases
source link: https://schneide.blog/2019/12/03/working-with-json-data-in-oracle-databases/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In my last post I showed how to work with JSON data in PostgreSQL . This time I want show how it is done with an Oracle database for comparison. I will use the same example scenario: a table named “events” where application events are stored in JSON format.
JSON data types
In Oracle there is no special data type for JSON data. You can use character string datatypes like VARCHAR2 or CLOB. However, you can add a special CHECK constraint to a column in order to ensure that only valid JSON is inserted:
CREATE TABLE events ( datetime TIMESTAMP NOT NULL, event CLOB NOT NULL CONSTRAINT event_is_json CHECK (event IS JSON) );
If you try to insert something other than JSON you will get a constraint violaiton error:
INSERT INTO events (datetime, event) VALUES (CURRENT_TIMESTAMP, 'This is not JSON.');
ORA-02290: check constraint (EVENT_IS_JSON) violated
Let’s insert some valid JSON data:
INSERT INTO events (datetime, event) VALUES (CURRENT_TIMESTAMP, '{"type": "add_shelf", "payload": {"id": 1}}'); INSERT INTO events (datetime, event) VALUES (CURRENT_TIMESTAMP, '{"type": "add_book", "payload": {"title": "Ulysses", "shelf": 1}}'); INSERT INTO events (datetime, event) VALUES (CURRENT_TIMESTAMP, '{"type": "add_book", "payload": {"title": "Moby Dick", "shelf": 1}}'); INSERT INTO events (datetime, event) VALUES (CURRENT_TIMESTAMP, '{"type": "add_shelf", "payload": {"id": 2}}'); INSERT INTO events (datetime, event) VALUES (CURRENT_TIMESTAMP, '{"type": "add_book", "payload": {"title": "Don Quixote", "shelf": 2}}');
Querying
In Oracle you use the JSON_VALUE function to select a value from a JSON structure. It uses a special path syntax for navigating JSON objects where the object root is represented as ‘$’ and properties are accessed via dot notation. This function can be used both in the SELECT clause and the WHERE clause:
SELECT JSON_VALUE(event, '$.type') AS type FROM events;
<strong>TYPE</strong> add_shelf add_book add_book add_shelf
SELECT event FROM events WHERE JSON_VALUE(event, '$.type')='add_book' AND JSON_VALUE(event, '$.payload.shelf')=1;
<strong>EVENT</strong> {"type":"add_book","payload":{"shelf":1,"title":"Ulysses"}} {"type":"add_book","payload":{"shelf":1,"title":"Moby Dick"}}
Constructing JSON objects
JSON objects can be constructed from values via the JSON_OBJECT and JSON_ARRAY functions:
SELECT JSON_OBJECT( 'id' VALUE 1, 'name' VALUE 'tree', 'isPlant' VALUE 'true' FORMAT JSON, 'colors' VALUE JSON_ARRAY('green', 'brown') ) FROM dual;
{"id":1,"name":"tree","isPlant":true,"colors":["green","brown"]}
Note that you have to use string values with the additional FORMAT JSON clause for boolean values.
Updating
Modifying JSON object fields has become feasible with the introduction of the JSON_MERGEPATCH function in Oracle 19c. It takes two JSON parameters:
1) the original JSON data
2) a JSON “patch” snippet that will be merged into the original JSON data. This can either add or update JSON properties.
It can be used in combination with JSON_VALUE and JSON_OBJECT. In this example we convert all the event “type” fields from lower case to upper case:
UPDATE events SET event=JSON_MERGEPATCH( event, JSON_OBJECT('type' VALUE UPPER(JSON_VALUE(event, '$.type'))) );
Oracle provides a lot more functions for working with JSON data. This post only covered the most basic ones. See the Oracle JSON reference for more.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK