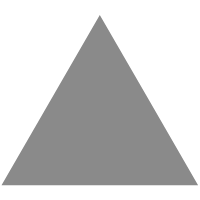
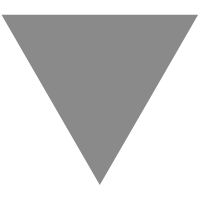
Swoft 2.0.3 重大更新,发布优雅的微服务治理 - Swoft - SegmentFault 思否
source link: https://segmentfault.com/a/1190000019713892?
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
什么是 Swoft ?
Swoft 是一款基于 Swoole 扩展实现的 PHP 微服务协程框架。Swoft 能像 Go 一样,内置协程网络服务器及常用的协程客户端且常驻内存,不依赖传统的 PHP-FPM。有类似 Go 语言的协程操作方式,有类似 Spring Cloud 框架灵活的注解、强大的全局依赖注入容器、完善的服务治理、灵活强大的 AOP、标准的 PSR 规范实现等等。
Swoft 通过长达三年的积累和方向的探索,把 Swoft 打造成 PHP 界的 Spring Cloud, 它是 PHP 高性能框架和微服务治理的最佳选择。
优雅的服务治理
Swoft 官方建议开发者使用 Service mesh 模式,比如 Istio/Envoy 框架,把业务和服务治理分开,但是 Swoft 也为中小型企业快速构建微服务提供了一套微服务组件。
服务注册与发现
服务注册与发现,需要用到 Swoft 官方提供的 swoft-consul 组件,如果其它第三方也类似。
注册与取消服务
监听 SwooleEvent::START
事件,注册服务
/**
* Class RegisterServiceListener
*
* @since 2.0
*
* @Listener(event=SwooleEvent::START)
*/
class RegisterServiceListener implements EventHandlerInterface
{
/**
* @Inject()
*
* @var Agent
*/
private $agent;
/**
* @param EventInterface $event
*/
public function handle(EventInterface $event): void
{
/* @var HttpServer $httpServer */
$httpServer = $event->getTarget();
$service = [
// ....
];
$scheduler = Swoole\Coroutine\Scheduler();
$scheduler->add(function () use ($service) {
// Register
$this->agent->registerService($service);
CLog::info('Swoft http register service success by consul!');
});
$scheduler->start();
}
}
监听 SwooleEvent::SHUTDOWN
事件,取消服务
/**
* Class DeregisterServiceListener
*
* @since 2.0
*
* @Listener(SwooleEvent::SHUTDOWN)
*/
class DeregisterServiceListener implements EventHandlerInterface
{
/**
* @Inject()
*
* @var Agent
*/
private $agent;
/**
* @param EventInterface $event
*/
public function handle(EventInterface $event): void
{
/* @var HttpServer $httpServer */
$httpServer = $event->getTarget();
$scheduler = Swoole\Coroutine\Scheduler();
$scheduler->add(function () use ($httpServer) {
$this->agent->deregisterService('swoft');
});
$scheduler->start();
}
}
定义服务提供者
/**
* Class RpcProvider
*
* @since 2.0
*
* @Bean()
*/
class RpcProvider implements ProviderInterface
{
/**
* @Inject()
*
* @var Agent
*/
private $agent;
/**
* @param Client $client
*
* @return array
* @example
* [
* 'host:port'
* ]
*/
public function getList(Client $client): array
{
// Get health service from consul
$services = $this->agent->services();
$services = [
];
return $services;
}
}
配置服务提供者
return [
'user' => [
'class' => ServiceClient::class,
'provider' => bean(RpcProvider::class)
// ...
]
];
Swoft 使用 @Breaker
注解实现熔断,可以在任何方法上面进行熔断操作。
/**
* Class BreakerLogic
*
* @since 2.0
*
* @Bean()
*/
class BreakerLogic
{
/**
* @Breaker(fallback="funcFallback")
*
* @return string
* @throws Exception
*/
public function func(): string
{
// Do something
throw new Exception('Breaker exception');
}
/**
* @return string
*/
public function funcFallback(): string
{
return 'funcFallback';
}
}
Swoft 中使用 @RateLimiter
注解实现服务限流,可以在任何方法上面限流,不仅仅是控制器,且 KEY 还支持 symfony/expression-language 表达式。
/**
* Class LimiterController
*
* @since 2.0
*
* @Controller(prefix="limiter")
*/
class LimiterController
{
/**
* @RequestMapping()
* @RateLimiter(key="request.getUriPath()", fallback="limiterFallback")
*
* @param Request $request
*
* @return array
*/
public function requestLimiter(Request $request): array
{
$uri = $request->getUriPath();
return ['requestLimiter', $uri];
}
/**
* @param Request $request
*
* @return array
*/
public function limiterFallback(Request $request): array
{
$uri = $request->getUriPath();
return ['limiterFallback', $uri];
}
}
配置中心,需要用到 Swoft 官方提供的 Swoft-apollo 组件,如果其它第三方也类似。
声明Agent
/**
* Class AgentCommand
*
* @since 2.0
*
* @Command("agent")
*/
class AgentCommand
{
/**
* @Inject()
*
* @var Config
*/
private $config;
/**
* @CommandMapping(name="index")
*/
public function index(): void
{
$namespaces = [
'application'
];
while (true) {
try {
$this->config->listen($namespaces, [$this, 'updateConfigFile']);
} catch (Throwable $e) {
CLog::error('Config agent fail(%s %s %d)!', $e->getMessage(), $e->getFile(), $e->getLine());
}
}
}
/**
* @param array $data
*
* @throws ContainerException
* @throws ReflectionException
*/
public function updateConfigFile(array $data): void
{
foreach ($data as $namespace => $namespaceData) {
$configFile = sprintf('@config/%s.php', $namespace);
$configKVs = $namespaceData['configurations'] ?? '';
$content = '<?php return ' . var_export($configKVs, true) . ';';
Co::writeFile(alias($configFile), $content, FILE_NO_DEFAULT_CONTEXT);
CLog::info('Apollo update success!');
/** @var HttpServer $server */
$server = bean('httpServer');
$server->restart();
}
}
}
启动Agent
Agent 只需要在服务(Http/RPC/Websocket)启动前,运行即可。
php bin/swoft agent:index
移除(Remove)
- 移除
request->json()
方法(c9e8f04)
新增(Enhancement):
- 新增接口依赖注入(6169f84)
- 新增
getFile
方法获取文件上传保存之后的信息(fe7e3a6) - 新增
restart()
服务新增重启方法(2ffec37) - 新增调用 1.x RPC 服务支持(30d73c3)
- 新增 AOP 类名匹配支持正则表达式(bc5e479)
- 新增 RPC Server /Http Server 中间件命名空间
use
错误提示(b1cec04) - 新增 验证器排除属性字段
unfields
(b1bf44f) - 新增 自动写入时间戳(dc58011)
- 新增 模型动作事件(dc58011)
- 新增 数据库迁移(26bb464)
- 新增 实体自动与 json 和数组互转(dc58011)
- 新增 模型批量更新方法
batchUpdateByIds
(dc58011)
修复(Fixed):
- 修复 cookies 设置时的一些问题,增加一些 withCookie 相关方法(b05afbb01)
- 修复 在console使用协程方式运行命令时,没有捕获处理错误(8a5418bf)
- 修复 websocket server 重启命令没有先停止旧server问题(db2d935)
- 修复任务返回值为
null
问题(a69347c) - 修复 RPC Server 只有类中间件无法使用问题()204bc7f
- 修复 RPC Server 返回值为
null
问题(4d091be) - 修复 Logger 和 CLog 日志等级无法覆盖和无效问题(8eb8aba)
- 修复 模型里面的属性不支持自定义表达式(dc58011)
更新(Update):
- 验证器优化,支持自定义验证规则(d959a4f)
- 重命名错误处理管理类
ErrorHanlders
为ErrorManager
(f3a8f04b) - console组件的异常处理改为由error组件提供的统一处理风格 (4f47204)
- console组件允许设置禁用命令组(c5a0269)
- 在默认的错误处理中,允许设置错误捕获级别。默认级别是
E_ALL | E_STRICT
(afff9029) - 优化 启动ws server时同时启用了http处理功能,信息面板添加提示(83a81170)
- 优化 启动ws server 并同时添加rpc server启动,信息面板没有显示 rpc server信息(3d1d0d848)
扩展(Extra):
- 文档添加支持通过google进行搜索
- 新增 apollo 组件
- 新增 consul 组件
- 新增 breaker 组件
- 新增 limter 组件
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK