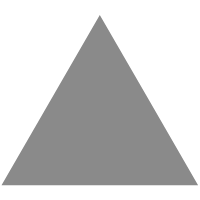
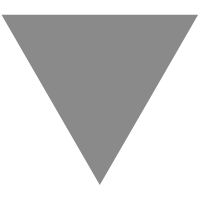
GitHub - hyperapp/hyperapp: 1 KB JavaScript library for building frontend applic...
source link: https://github.com/jbucaran/hyperapp
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Hyperapp
The tiny framework for building hypertext applications.
- Do more with less—We have minimized the concepts you need to learn to get stuff done. Views, actions, effects, and subscriptions are all pretty easy to get to grips with and work together seamlessly.
- Write what, not how—With a declarative API that's easy to read and fun to write, Hyperapp is the best way to build purely functional, feature-rich, browser-based apps in JavaScript.
- Smaller than a favicon—1 kB, give or take. Hyperapp is an ultra-lightweight Virtual DOM, highly-optimized diff algorithm, and state management library obsessed with minimalism.
Here's the first example to get you started—look ma' no bundlers! Try it in your browser.
<!DOCTYPE html> <html lang="en"> <head> <script type="module"> import { h, text, app } from "https://unpkg.com/hyperapp" const AddTodo = (state) => ({ ...state, value: "", todos: state.todos.concat(state.value), }) const NewValue = (state, event) => ({ ...state, value: event.target.value, }) app({ init: { todos: [], value: "" }, view: ({ todos, value }) => h("main", {}, [ h("h1", {}, text("To do list")), h("input", { type: "text", oninput: NewValue, value }), h("ul", {}, todos.map((todo) => h("li", {}, text(todo))) ), h("button", { onclick: AddTodo }, text("New!")), ]), node: document.getElementById("app"), }) </script> </head> <body> <main id="app"></main> </body> </html>
Now that you have poked around the code a bit, you may have some questions. What is init
and view
, and how do they fit together? The app starts off with init
, where we set the initial state. The view
returns a plain object that represents how we want the DOM to look (the virtual DOM) and Hyperapp takes care of modifying the actual DOM to match this specification whenever the state changes. That's really all there is to it.
Ready to dive in? Learn the basics in the tutorial or visit the API reference for more documentation. If you prefer to learn by studying real-world examples, you can browse our awesome list of resources too.
Installation
Install Hyperapp with npm or Yarn:
npm i hyperapp
Then with a module bundler like Rollup or Webpack import it in your application and get right down to business.
import { h, text, app } from "hyperapp"
Don't want to set up a build step? Import Hyperapp in a <script>
tag as a module. Don't worry; modules are supported in all evergreen, self-updating desktop, and mobile browsers.
<script type="module"> import { h, text, app } from "https://unpkg.com/hyperapp" </script>
Packages
Official packages provide access to The Web Platform, and ensure that the APIs are exposed in a way that makes sense for Hyperapp, and the underlying code is stable. We already cover a decent amount of features, but you can always create your own effects and subscriptions if something is not available yet.
Package | Status | About |
---|---|---|
@hyperapp/dom |
Inspect the DOM, focus and blur. | |
@hyperapp/svg |
Draw SVG with plain functions. | |
@hyperapp/html |
Write HTML with plain functions. | |
@hyperapp/time |
Subscribe to intervals, get the time now. | |
@hyperapp/http |
Talk to servers, make HTTP requests. | |
@hyperapp/events |
Subscribe to mouse, keyboard, window, and frame events. | |
@hyperapp/random |
Declarative random numbers and values. | |
@hyperapp/navigation |
Subscribe and manage the browser URL history. |
Help, I'm stuck!
Don't panic! If you've hit a stumbling block, hop on our Discord server to get help, and if you don't receive an answer, or if you remain stuck, please file an issue, and we'll figure it out together.
Contributing
Hyperapp is free and open source software. If you love Hyperapp, becoming a contributor or sponsoring is the best way to give back. Thank you to everyone who already contributed to Hyperapp!
License
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK