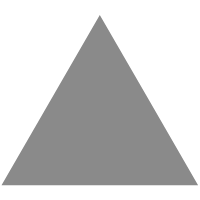
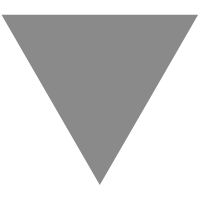
📹 3 ways to loop over Object properties with Vanilla JavaScript (ES6...
source link: https://blog.mrfrontend.org/2017/09/3-ways-to-loop-over-object-properties-with-vanilla-javascript/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
📹 3 ways to loop over Object properties with Vanilla JavaScript (ES6 included)
Raymon S
—September 29, 2017
It happens a lot that you need to loop over an Array with JavaScript Objects! But sometimes you just don’t know what kind of properties that Object has. Lucky we are that JavaScript offers a few ways of looping over JavaScript Object properties.
I wanted to share 3 methods with you. Hopefully this will help you in the right direction. Check out the video! If you prefer to read, check the text below the video 😉
Notes & Resources of the Video!
The 3 methods to loop over Object Properties in JavaScript are:
ES6/ES2015
Maybe you heard about ES6 or ES2015. That is the new modern specification of JavaScript nowadays. ES6 has a lot of new cool features like the Arrow Function and Template strings. It's making a lot of things easier in my opinion!
In these examples, I will use some ES6 stuff. Not every browser will support everything that I will show you, so keep that in mind while using it in production web applications/websites.
If you want to learn more about ES6/ES2015 please check out the course of our friend Wes Bos (and my personal hero in JavaScript Teaching) 😎. I have followed it myself and it is super-duper-awesome!
Wes Bos also have a great blog about the ES6 Arrow functions, please check it out!
Let's get back to where we are all talking about 😉
The Object to loop over
First we need an example object to loop over. So I put some of my experience in it 😉 (hahaha)! Keep the fun in it!
Object.keys(object)
The Object.keys() method will return an array of keys. If you put this in a variable and put it in a console.log() you will see an array of the keys.
Object.keys(experienceObject).map()
With the Object.keys.map method we are gonna loop over the Array that the Objec.keys has returned. The map method is one of the JavaScript ways to loop over an Array.
The map method is one of the JavaScript ways to loop over an Array.
Object.entries(object)
The Object.keys() method will return an array of keys. If you put this in a variable and put it in a console.log() you will see an array of the keys.
Be aware that this is ES2017! Thanks to people on Reddit who put it in the comments!``` var objectEntries = entries(experienceObject); console.log(‘objectEntries: ‘, objectEntries);```
Object.entries(experienceObject).forEach()
With the Object.keys.forEach method we are gonna loop over the Array of key-value pairs that the Object.entries has returned.
In this case we use the forEach method to loop over the Array. The forEach another simple method to loop over an Array instead of the for-loop.
For-in loop
The last example is the For-in loop to loop over the properties of the Object it.
The for-in loop is a whole lot simpler than the for-loop.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK