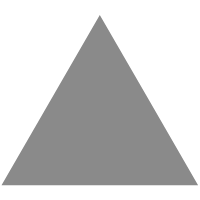
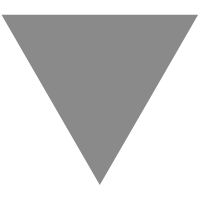
The 30-Day .NET Challenge - Day 30: XML vs JSON Serialization
source link: https://hackernoon.com/the-30-day-net-challenge-day-30-xml-vs-json-serialization
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The 30-Day .NET Challenge - Day 30: XML vs JSON Serialization
Sukhpinder Singh
@ssukhpinder
Programmer by heart | C# | Python | .Net Core...

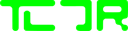
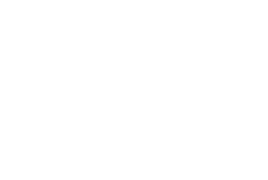
Too Long; Didn't Read
Serialization involves a process of converting an object into an easily stored format. The article demonstrates the problem with old XML Serialization and how JSON serialization improves both efficiency and effectiveness.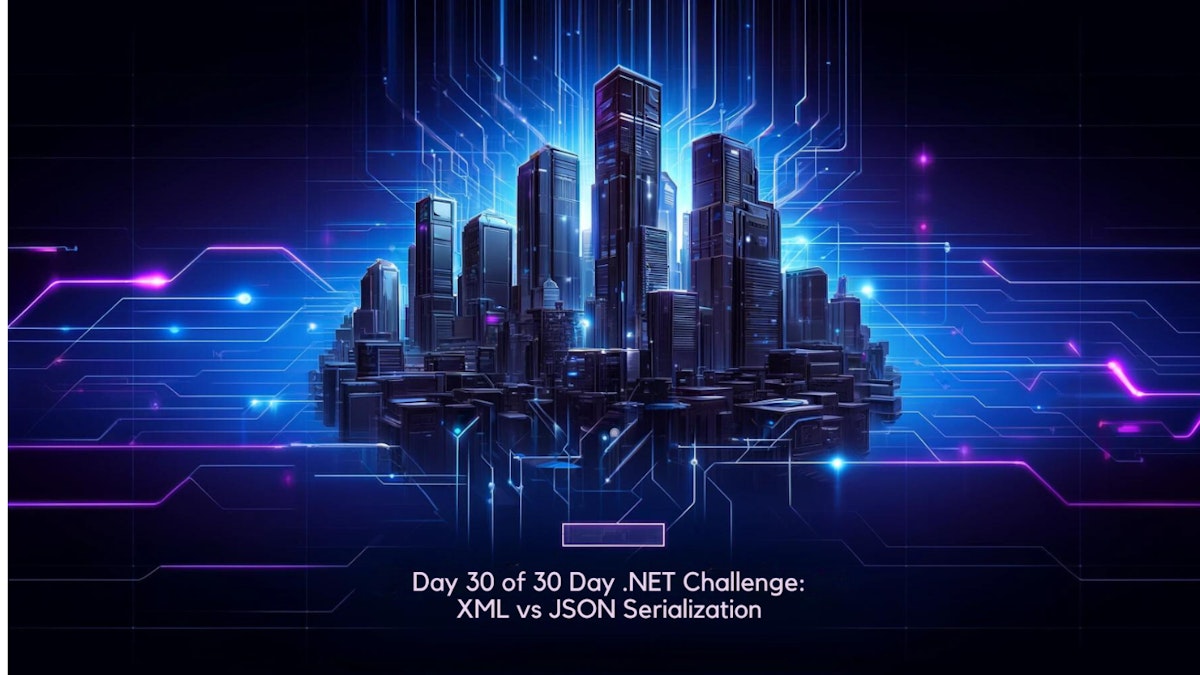
audio
element.Learn to enhance your code with JSON Serialization in C#. Discover a better approach on Day 30 of our 30-Day .NET Challenge.
Introduction
Serialization involves a process of converting an object into an easily stored format. The article demonstrates the problem with old XML Serialization and how JSON serialization improves both efficiency and effectiveness.
Learning Objectives
- Drawbacks of XML Serialization
- Advantages of JSON Serialization
Prerequisites for Developers
- Basic understanding of C# programming language.
Getting Started
Drawbacks of XML Serialization
Traditionally many developers have used XML Serialization as demonstrated in the following code snippet.
// Using XmlSerializer for data serialization
private string SerializeObjectToXml<T>(T obj)
{
var serializer = new XmlSerializer(typeof(T));
using (var writer = new StringWriter())
{
serializer.Serialize(writer, obj);
return writer.ToString();
}
}
Even though XML is human-readable and globally supported it is not an optimized and efficient choice of serialization in the C# programming language. The main reason is that it involves a lot of temporary objects which can impact the memory usage and the corresponding GC pressure.
Advantages of JSON Serialization
Please find below the refactored version of the previous code snippet using NewtonSoft.Json library
// Using Newtonsoft.Json for data serialization
private string SerializeObjectToJson<T>(T obj)
{
return JsonConvert.SerializeObject(obj);
}
The aforementioned library outperforms XmlSerializer in both speed and efficiency. In addition to that, the JSON files are smaller in size which makes reading and writing faster.
Complete Code
Create another class named EfficientSerialization and add the following code snippet
public static class EfficientSerialization
{
public static string XML<T>(T obj)
{
var serializer = new XmlSerializer(typeof(T));
using (var writer = new StringWriter())
{
serializer.Serialize(writer, obj);
return writer.ToString();
}
}
public static string JSON<T>(T obj)
{
return JsonConvert.SerializeObject(obj);
}
}
And create a model class as follows:
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
Execute from the main method as follows
#region Day 30: Efficient Serialization
static string ExecuteDay30()
{
Person person = new Person { Name = "John Doe", Age = 30 };
// XML Serialization
string xmlData = EfficientSerialization.XML(person);
Console.WriteLine("XML Serialization Output:");
Console.WriteLine(xmlData);
// JSON Serialization
string jsonData = EfficientSerialization.JSON(person);
Console.WriteLine("JSON Serialization Output:");
Console.WriteLine(jsonData);
return "Executed Day 30 successfully..!!";
}
#endregion
Console Output
XML Serialization Output:
<?xml version="1.0" encoding="utf-16"?>
<Person xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Name>John Doe</Name>
<Age>30</Age>
</Person>
JSON Serialization Output:
{"Name":"John Doe","Age":30}
Complete Code here: GitHub — ssukhpinder/30DayChallenge.Net
Thank you for being a part of the C# community! Before you leave:
Follow us: Youtube | X | LinkedIn | Dev.to Visit our other platforms: GitHub More content at C# Programming
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK