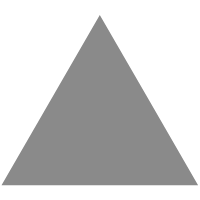
1
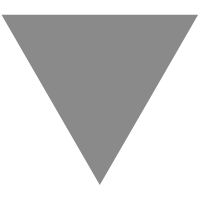
Django开发中,Form和ModelForm中的钩子函数的作用和具体用法详解
source link: https://blog.51cto.com/u_13188203/10512495
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Django开发中,Form和ModelForm中的钩子函数的作用和具体用法详解
精选 原创在 Django 的表单处理中,Form
和 ModelForm
类中的钩子函数提供了强大的机制,用于在数据保存到数据库之前或之后进行自定义操作。这些钩子函数允许开发者插入自定义逻辑,例如验证数据、修改数据或执行某些业务规则。
常见的钩子函数
以下是在 Django Form
和 ModelForm
中常用的几个钩子函数:
clean_<fieldname>()
:
- 这是一个字段级的钩子函数,用于对单个表单字段进行额外的清洗和验证。
- 它应该返回该字段的清洗后的值或者抛出
ValidationError
。
clean()
:
- 这是在所有字段的
clean_<fieldname>()
方法之后执行的方法。 - 用于对表单的多个字段进行跨字段的验证。
- 它可以访问
self.cleaned_data
字典来获取已经清洗过的数据并进行进一步的处理。
save(commit=True)
:
- 只在
ModelForm
中有这个方法。 - 用于保存表单到数据库。
commit
参数控制是否立即保存模型实例。如果commit=False
,它将返回一个模型实例,但不会保存到数据库。
以下是如何在 Django Form
和 ModelForm
中使用这些钩子函数的示例。
1. 使用 clean_<fieldname>()
from django import forms
from django.core.exceptions import ValidationError
class MyForm(forms.Form):
age = forms.IntegerField()
def clean_age(self):
age = self.cleaned_data['age']
if age < 18:
raise ValidationError("You must be at least 18 years old.")
return age
在这个示例中,clean_age()
方法确保提交的年龄至少为 18。
2. 使用 clean()
class SignupForm(forms.Form):
password = forms.CharField(widget=forms.PasswordInput)
confirm_password = forms.CharField(widget=forms.PasswordInput)
def clean(self):
cleaned_data = super().clean()
password = cleaned_data.get("password")
confirm_password = cleaned_data.get("confirm_password")
if password and confirm_password and password != confirm_password:
raise ValidationError("The two password fields must match.")
return cleaned_data
这里,clean()
方法用于检查两次输入的密码是否一致。
3. 使用 save()
from django.forms import ModelForm
from myapp.models import Book
class BookForm(ModelForm):
class Meta:
model = Book
fields = ['title', 'author']
def clean_title(self):
title = self.cleaned_data['title']
if 'Django' not in title:
raise ValidationError("Every book must have Django in the title.")
return title
def save(self, commit=True):
book = super().save(commit=False)
# 自定义保存逻辑
book.some_field = 'Some value'
if commit:
book.save()
return book
在这个例子中,save()
方法被重写以添加额外的逻辑在保存之前。
使用 Django 表单的钩子函数可以让你在数据处理流程中插入自定义的验证或处理逻辑,使得表单处理更加灵活和强大。通过这些钩子函数,你可以精确控制数据的验证和保存过程,确保数据的完整性和应用逻辑的正确性。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK