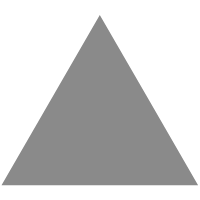
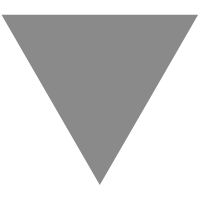
How to Retrieve the IDs, Titles, and URLs of the Core WordPress/WooCommerce Page...
source link: https://webdesign.tutsplus.com/how-to-retrieve-the-ids-titles-urls-of-the-core-wordpresswoocommerce-pages--cms-108631a
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
As WordPress developers, many times we want to grab different info (ID, title, URL) for standard WordPress/WooCommerce pages like the Blog and My Account pages.
Surprisingly, all this useful stuff is stored in the *_options
table, and we can access it using the get_option()
function. There’s also the wp_load_alloptions()
that we can use to print them all on the page.
Let’s see it in action!
Get Static WordPress Pages
Imagine that, on our site, we’ve registered static pages as front and blog pages.

WordPress stores the ID of these pages as values of the page_on_front
and page_for_posts
options.
From there we can access the titles and URLs using the built-in get_the_title()
and get_permalink()
functions.
It’s worth noting that another way of immediately getting the blog archive page URL is by using the get_post_type_archive_link()
function and passing the post
keyword as a parameter. For example, if we want to grab the archive page URL for the member
custom post type, we’ll pass the member
parameter to this function.
Static Front Page
/* GET URL */ |
|
2 |
function get_front_page_url() { |
3 |
return get_permalink( get_option( 'page_on_front' ) ); |
4 |
} |
5 |
|
6 |
/* GET TITLE */ |
7 |
function get_front_page_title() { |
8 |
return get_the_title( get_option( 'page_on_front' ) ); |
9 |
} |
Static Blog Page
/* GET URL */ |
|
2 |
// Method 1 |
3 |
function get_blog_page_url() { |
4 |
return get_permalink( get_option( 'page_for_posts' ) ); |
5 |
} |
6 |
|
7 |
// Method 2 - call get_blog_page_url2( 'post' ) |
8 |
function get_blog_page_url2( $type ) { |
9 |
return get_post_type_archive_link( $type ); |
} |
|
/* GET TITLE */ |
|
function get_blog_page_title() { |
|
return get_the_title( get_option( 'page_for_posts' ) ); |
|
} |
Get Static WooCommerce Pages
Among others, WooCommerce also stores the IDs of its essential pages like My Account, Shop, Cart, and Checkout pages in the *_options
table.

As discussed above, we can then access their titles and URLs using WordPress core functions.
WooCommerce also provides some useful functions like the ones below that we can use to access directly the page parts we want:
My Account Page
/* GET URL */ |
|
2 |
// Method 1 |
3 |
function get_my_account_page_url() { |
4 |
return get_permalink( get_option( 'woocommerce_myaccount_page_id' ) ); |
5 |
} |
6 |
|
7 |
// Method 2 |
8 |
function get_my_account_page_url2() { |
9 |
return get_permalink( wc_get_page_id( 'myaccount' ) ); |
} |
|
// Method 3 |
|
function get_my_account_page_url3() { |
|
return wc_get_page_permalink( 'myaccount' ); |
|
} |
|
/* GET TITLE */ |
|
// Method 1 |
|
function get_my_account_page_title() { |
|
20 |
return get_the_title( get_option( 'woocommerce_myaccount_page_id' ) ); |
21 |
} |
22 |
|
23 |
// Method 2 |
24 |
function get_my_account_page_title2() { |
25 |
return get_the_title( wc_get_page_id( 'myaccount' ) ); |
26 |
} |
Shop Page
/* GET URL */ |
|
2 |
// Method 1 |
3 |
function get_shop_page_url() { |
4 |
return get_permalink( get_option( 'woocommerce_shop_page_id' ) ); |
5 |
} |
6 |
|
7 |
// Method 2 |
8 |
function get_shop_page_url2() { |
9 |
return get_permalink( wc_get_page_id( 'shop' ) ); |
} |
|
// Method 3 |
|
function get_shop_page_url3() { |
|
return wc_get_page_permalink( 'shop' ); |
|
} |
|
/* GET TITLE */ |
|
// Method 1 |
|
function get_shop_page_title() { |
|
20 |
return get_the_title( get_option( 'woocommerce_shop_page_id' ) ); |
21 |
} |
22 |
|
23 |
// Method 2 |
24 |
function get_shop_page_title2() { |
25 |
return get_the_title( wc_get_page_id( 'shop' ) ); |
26 |
} |
Cart Page
/* GET URL */ |
|
2 |
// Method 1 |
3 |
function get_cart_page_url() { |
4 |
return get_permalink( get_option( 'woocommerce_cart_page_id' ) ); |
5 |
} |
6 |
|
7 |
// Method 2 |
8 |
function get_cart_page_url2() { |
9 |
return get_permalink( wc_get_page_id( 'cart' ) ); |
} |
|
// Method 3 |
|
function get_cart_page_url3() { |
|
return wc_get_page_permalink( 'cart' ); |
|
} |
|
// Method 4 |
|
function get_cart_page_url4() { |
|
return wc_get_cart_url(); |
|
20 |
} |
21 |
|
22 |
/* GET TITLE */ |
23 |
// Method 1 |
24 |
function get_cart_page_title() { |
25 |
return get_the_title( get_option( 'woocommerce_cart_page_id' ) ); |
26 |
} |
27 |
|
28 |
// Method 2 |
29 |
function get_cart_page_title2() { |
30 |
return get_the_title( wc_get_page_id( 'cart' ) ); |
31 |
} |
Checkout Page
/* GET URL */ |
|
2 |
// Method 1 |
3 |
function get_checkout_page_url() { |
4 |
return get_permalink( get_option( 'woocommerce_checkout_page_id' ) ); |
5 |
} |
6 |
|
7 |
// Method 2 |
8 |
function get_checkout_page_url2() { |
9 |
return get_permalink( wc_get_page_id( 'checkout' ) ); |
} |
|
// Method 3 |
|
function get_checkout_page_url3() { |
|
return wc_get_page_permalink( 'checkout' ); |
|
} |
|
// Method 4 |
|
function get_checkout_page_url4() { |
|
return wc_get_checkout_url(); |
|
20 |
} |
21 |
|
22 |
/* GET TITLE */ |
23 |
// Method 1 |
24 |
function get_checkout_page_title() { |
25 |
return get_the_title( get_option( 'woocommerce_checkout_page_id' ) ); |
26 |
} |
27 |
|
28 |
// Method 2 |
29 |
function get_checkout_page_title2() { |
30 |
return get_the_title( wc_get_page_id( 'checkout' ) ); |
31 |
} |
Other WooCommerce Plugins
Not surprisingly, other WooCommerce plugins store info about their pages in the *_options
table. For example, consider the Yith WooCommerce Wishlist popular plugin that allows users to save their popular products on the Wishlist page.
As long as we install and activate it, in the database we’ll see a new entry for the Wishlist page.
/* GET URL */ |
|
2 |
// Method 1 |
3 |
function get_yith_wishlist_page_url() { |
4 |
return get_permalink( get_option( 'yith_wcwl_wishlist_page_id' ) ); |
5 |
} |
6 |
|
7 |
// Method 2 |
8 |
function get_yith_wishlist_page_url2() { |
9 |
return YITH_WCWL()->get_wishlist_url(); |
} |
|
/* GET TITLE */ |
|
function get_yith_wishlist_page_title() { |
|
return get_the_title( get_option( 'yith_wcwl_wishlist_page_id' ) ); |
|
} |
Conclusion
Done! Today, we learned different methods for accessing the IDs, titles, and URLs of the core WordPress and WooCommerce pages. I hope you’ll have this tutorial as a reference any time you want to access such a page. Last but not least, all the code covered here is included in this Gist.
Remember that the best way to learn and find what you want is by looking in the database and checking the native files of WordPress and WooCoomerce.
As always, thanks a lot for reading!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK