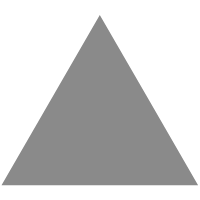
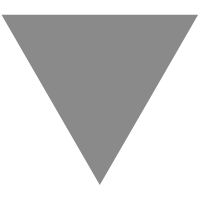
SwiftUI Cookbook [FREE]
source link: https://www.kodeco.com/books/swiftui-cookbook
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The SwiftUI Cookbook is a reference for common patterns for creating user interfaces in SwiftUI that you can quickly refer to while coding.
Getting Started with SwiftUI
Welcome to the Getting Started with SwiftUI section! This is where your journey to becoming a SwiftUI wizard begins.
We will start with the basics, exploring how to Create & Preview a SwiftUI View. Then, we delve into the foundational concept of SwiftUI Views & View Hierarchies, where you’ll understand the structure of SwiftUI apps and how views are layered.
We will then move on to Use Xcode Previews with SwiftUI, a fantastic feature that allows you to see your code changes in real-time.
From there, we’ll build your toolset by teaching you how to add various components to your SwiftUI views. Chapters like Add a Text View in SwiftUI, Add an Image View in SwiftUI and Add a Button in SwiftUI will guide you through adding and working with these elemental views.
We will also discuss more complex views like Add a NavigationStack in SwiftUI, Add a List View in SwiftUI and Add a Form in SwiftUI, which will open up a vast array of possibilities for your app interfaces.
By the end of this section, you will have a solid foundation in SwiftUI and be ready to explore more advanced topics. So, let’s jump right in!
Views & Modifiers in SwiftUI
Welcome to the Views & Modifiers in SwiftUI section! Here, we’re going to discover the wonderful world of creating and customizing views - the building blocks of any SwiftUI application.
We begin with the fundamentals in Create a View in SwiftUI, where you’ll learn how to start making your own interactive interfaces. From there, we’ll dive right into view customization, starting with how to Customize View Background & Border and Add Shadows to Views in SwiftUI for that perfect aesthetic.
Further customizations are covered in the chapters Clip Views in SwiftUI, where we explore cropping, and Adjust View Opacity in SwiftUI, delving into transparency effects. If you’re curious about how to create more unique shapes, Create a Circular View in SwiftUI and Add a Custom Shape to a View in SwiftUI are exactly what you need.
We also have a chapter on creating a grid-like interface with Build a Grid of Views in SwiftUI, a commonly used layout in various apps.
The chapter on Create a Custom View Modifier in SwiftUI will provide you with the tools to create reusable and adaptable components to maintain a consistent and manageable codebase.
By the end of this section, you’ll be well-versed in crafting beautiful and custom interfaces with SwiftUI views and modifiers. Let’s get started!
Managing User Interface State in SwiftUI
Welcome to the Managing User Interface State in SwiftUI section! In this part of the SwiftUI Cookbook, we’ll delve into the dynamic world of state management and how it enables us to build interactive, responsive, and data-driven applications.
Firstly, you’ll be introduced to the fundamental concepts of State & Binding in SwiftUI, the building blocks of SwiftUI’s reactive design paradigm. We then take a step further to explore ObservableObject & ObservedObject and how they help keep our views in sync with our data.
But what about sharing state across multiple views? This is where Environment Objects step in. You’ll learn how to effectively use them to share data in an app, followed by understanding the role of StateObject to manage observable objects.
Next, we’ll delve into how Combine With SwiftUI can be used for more complex state management scenarios. For persistent state, we’ll discuss AppStorage & SceneStorage, enabling you to store user settings and per-scene state respectively.
In the chapter Creating & Accessing Environment Values in SwiftUI, we explore how to influence SwiftUI’s environment and share values effectively.
To round off the section, we’ll discuss Best Practices for State Management in SwiftUI that’ll help you avoid common pitfalls and write more maintainable SwiftUI apps.
By the end of this section, you’ll have a firm grasp on managing user interface state, setting you up to build robust and reactive SwiftUI apps. Let’s dive in!
Text & Fonts in SwiftUI
Welcome to the Text & Fonts in SwiftUI section! Text is a fundamental part of any application and in this section, we’ll focus on how to work with text views, styles, custom fonts, and text fields in SwiftUI.
We’ll start off by creating a basic Text View in SwiftUI. Once you’re comfortable with creating simple text views, we’ll jump into Styling Text in SwiftUI to make your text pop out and match your app’s theme. To handle larger blocks of text, we’ll dive into Creating a Multiline Text View in SwiftUI.
You’ll also learn about Applying Dynamic Type Text Styles in SwiftUI which allows your app to respond to user’s font size preferences. We’ll also look at Formatting Text in SwiftUI to display numbers, dates, or custom formats.
When it comes to unique styles, using custom fonts can make your app stand out. In Using Custom Fonts in SwiftUI, you’ll learn how to import and use your own font files.
In the latter part of the section, you’ll find out how to Create a Text Field in SwiftUI and ensure the privacy of text input with Creating a Secure Field in SwiftUI. We’ll wrap up the section by guiding you on how to Adjust the Text Field Keyboard Type in SwiftUI for different types of input.
By the end of this section, you’ll have a thorough understanding of how to handle text in SwiftUI in a way that is both user-friendly and design-conscious. Let’s get started!
Images & Icons in SwiftUI
Welcome to the Images & Icons in SwiftUI section! Images are one of the most impactful ways to add life to your applications. In this section, we’ll explore a wide range of techniques for handling images and icons, making your SwiftUI applications visually appealing.
Start with the basics by learning how to Load an Image in SwiftUI, before moving on to handling different aspect ratios with Show Images with Different Aspect Ratios in SwiftUI. After that, you’ll learn how to manipulate images with chapters like Crop an Image in SwiftUI, Apply a Filter to an Image in SwiftUI, and Blend Two Images Together in SwiftUI.
Next, you’ll explore SF Symbols, a library of thousands of symbols you can use in your app, by Adding an Icon from SF Symbols in SwiftUI. Further, you’ll discover how to get creative with your images by Creating a Custom Shape for an Image in SwiftUI and Adding a Shadow to an Image in SwiftUI.
As you move deeper, you’ll encounter some unique image-related features, like Using Animated Images in SwiftUI, a fun way to bring dynamism to your app, and Using the SwiftUI PhotosPicker to let users pick photos from their library.
We’ll round off the section by delving into creating visually consistent designs with Create Consistent SwiftUI Designs. By the end of this section, you’ll be well-equipped to work with images and icons in SwiftUI, giving your apps a strong visual identity. Let’s dive in!
Buttons in SwiftUI
Welcome to the Buttons in SwiftUI section. Buttons are a fundamental part of almost every application, used to perform actions or trigger changes. In this section, you will learn how to create and customize buttons, making your SwiftUI applications interactive and intuitive.
Firstly, you will Create a Button in SwiftUI and quickly move onto how to Customize the Appearance of a Button in SwiftUI, making your buttons visually appealing and in sync with your app’s aesthetic. Then, you’ll explore adding visual elements to buttons by Adding an Image to a Button in SwiftUI and Adding an Icon to a Button in SwiftUI.
Next up, you’ll learn how to add functionality to your buttons by Adding an Action to a Button in SwiftUI, an essential part of any interactive application. You’ll also discover how to Create a Toggle Button in SwiftUI for situations where you need a binary on-off type of interaction.
Exploring group dynamics, you will learn to Create a Group of Buttons in SwiftUI, which is handy when you want to present a set of related actions. You’ll also gain insight into how to Disable a Button in SwiftUI, useful for controlling user interaction based on certain conditions.
As you move further, you’ll tackle Creating a Full-Screen Button in SwiftUI, an impactful design choice for certain use cases. The section culminates with Advanced Button Styling in SwiftUI, where you’ll explore more complex and intricate styling options for your buttons.
By the end of this section, you’ll have a solid understanding of how to create and manipulate buttons in SwiftUI, adding a vital interactive element to your apps. Let’s get started!
Lists & Navigation in SwiftUI
Welcome to the Lists & Navigation in SwiftUI section. Navigational interfaces and lists form the core of most mobile applications, and SwiftUI makes it simple to implement them. This section will guide you through the process, ensuring you’re equipped with the knowledge to create rich, dynamic, and interactive list-based interfaces.
You’ll start with the basics of Creating a List in SwiftUI before diving deeper into how to Customize List Rows in SwiftUI. Navigational aspects are introduced in Adding Navigation to a List in SwiftUI, followed by further elaboration on how to Create a NavigationTitle in SwiftUI and Add a Button to a NavigationBar in SwiftUI.
Sectioning lists is a great way to organize content, and you’ll learn how to Implement Section Headers in a List in SwiftUI. Searching within lists is a critical feature for many apps, and the chapter on Creating a Search Bar in a List in SwiftUI will guide you through its implementation.
Next, you’ll discover how to enhance the user experience by Adding Swipe Actions to a List in SwiftUI. This will be followed by exploring how to Create a TabView with Lists in SwiftUI, combining tabs and lists for a more complex interface.
Finally, the section will end with a bang as you tackle Creating an Infinitely Scrolling List in SwiftUI, a common feature in apps displaying large volumes of data.
By the end of this section, you’ll be proficient in creating and managing lists and navigation within SwiftUI. Let’s jump in!
Forms & Controls in SwiftUI
Welcome to the Forms & Controls in SwiftUI section. Creating intuitive and easy-to-use forms is an essential skill in any developer’s toolkit. In this section, you’ll delve into the various controls and elements you can utilize in SwiftUI to create functional and user-friendly forms.
You’ll start by learning how to Create a Form With Sections in SwiftUI, providing a structured approach to form design. From there, you’ll move onto the various form controls available, each with a dedicated chapter: Creating a Checkbox in SwiftUI, Adding a Stepper in SwiftUI, Creating a Picker in SwiftUI, Creating a Date Picker in SwiftUI, and Creating a Slider in SwiftUI.
You’ll also get to grips with Creating a Segmented Control in SwiftUI, a common user interface element used to present a group of options. The chapter Adding a Progress View in SwiftUI will guide you on how to show the status of tasks within your form, while Creating a Color Picker in SwiftUI will help you explore more interactive and visual elements you can use within forms.
By the end of this section, you’ll have a broad understanding of how to construct and customize forms using SwiftUI’s built-in controls. Let’s get started!
Tab Views & Split Views in SwiftUI
Welcome to the Tab Views & Split Views in SwiftUI section. This part of the cookbook is dedicated to the exploration and mastery of SwiftUI’s tab and split views. These components are essential for creating robust navigation and user experiences in modern apps.
Begin your journey with Creating a Tab View in SwiftUI before diving into how to Customize Tab View Appearance in SwiftUI to better align with your app’s aesthetics. You’ll learn how to Add Custom Icons to Tab View Items in SwiftUI, enriching the visual feedback for your users.
Next up, explore the world of split views. Start by Creating a Split View in SwiftUI. Then, delve into customizing its look with Customize Split View Appearance in SwiftUI. In Adding a Detail View to Split View in SwiftUI, you’ll learn how to provide deeper context to your users in a well-structured layout.
Continue your learning journey with Presenting a Modal View from a Tab View in SwiftUI and Switching Tabs Programmatically in SwiftUI, providing dynamic interactions for your app’s users.
You’ll then understand how to Hide a Tab View in SwiftUI for different scenarios. Conclude with Adding a Tab View to a Navigation View in SwiftUI to combine SwiftUI’s powerful navigation tools in a harmonious blend.
By the end of this section, you’ll have a solid grasp of tab views and split views in SwiftUI, allowing you to create intuitive and engaging navigation experiences in your apps. Let’s dive in!
Progress Indicators in SwiftUI
Welcome to the Progress Indicators in SwiftUI section. Progress indicators are an essential part of the user interface when it comes to providing feedback to users about ongoing tasks or loading content. In this section, you’ll explore various ways to create and customize progress indicators using SwiftUI.
Kick things off by learning how to Animate a Progress Bar in SwiftUI and how to Create a Spinning Activity Indicator in SwiftUI, two common types of indicators that provide clear visual feedback to users.
Customization can make progress indicators fit seamlessly into your app’s design. Discover how to Customize the Style of Progress Indicators in SwiftUI and create different styles of progress bars, such as Creating a Circular Progress Bar in SwiftUI.
You’ll also learn how to work with both determinate and indeterminate progress indicators in SwiftUI, which are important for showing definite and indefinite progress respectively.
Adding progress views to your navigation bar can be a great way to provide non-intrusive progress updates. Learn how to do this in the chapter on Adding a Progress View to a Navigation Bar in SwiftUI.
Finally, flex your creativity muscles and create your own progress views with the chapters on Making a Custom Segmented Progress Bar in SwiftUI and Creating a Custom Progress View in SwiftUI.
By the end of this section, you’ll have the skills to effectively use progress indicators in SwiftUI, enhancing the overall user experience of your app. Let’s show some progress!
Frames & Layouts in SwiftUI
Welcome to the Frames & Layouts in SwiftUI section. This is where you’ll learn to expertly arrange and align your views, creating a cohesive and visually appealing user interface in your SwiftUI applications.
Kick things off by Understanding Frames & Alignment in SwiftUI, the foundation of your view layout. You’ll then dive into the mechanics of view fitting with Exploring ViewThatFits in SwiftUI.
Learn about creating grid layouts, a common UI pattern, with Using LazyVGrid & LazyHGrid for Grid Layouts in SwiftUI. For creating scrollable content, the chapter on Using ScrollView in SwiftUI has got you covered.
Stack views are fundamental in SwiftUI. Understand how to arrange your views vertically and horizontally with Understanding ZStack & VStack in SwiftUI and Understanding HStack & Spacer in SwiftUI.
Unlock the power of dynamic layouts with Understanding GeometryReader in SwiftUI. This is followed by an exploration of Adding Padding & Spacing in SwiftUI to perfectly space your views.
Up next, dive deeper into alignment with Using Alignment Guides in SwiftUI. Finally, master the art of laying out your views within the safe areas of the device screen with Mastering Safe Areas in SwiftUI.
By the end of this section, you’ll have a deep understanding of how SwiftUI handles view layouts and frames, giving you the ability to build polished and adaptive UIs. Let’s get arranging!
Animations & Transitions in SwiftUI
Animating your SwiftUI app adds a layer of polish that keeps users engaged and # Animations & Transitions in SwiftUI
Welcome to the Animations & Transitions in SwiftUI section. Animations are a powerful tool to make your app stand out, providing visual feedback and making your app feel more interactive and engaging. In this part of the guide, you’ll learn to incorporate animations and transitions into your SwiftUI views.
Begin your journey into SwiftUI animations by learning how to Declare an Animation in SwiftUI. Once you’ve got the basics down, you’ll explore how to animate various aspects of your views such as Animating a View’s Opacity, Position, Rotation and Size in SwiftUI.
Next, dive into more complex and nuanced animation types by learning how to Create a Spring Animation, Create a Delayed Animation and Create a Repeating Animation in SwiftUI.
You’ll also discover how to create intricate animations by learning how to Chain Multiple Animations in SwiftUI and how to Animate Binding Values in SwiftUI.
Finally, make your view presentations and dismissals more dynamic by understanding how to Animate View Transitions in SwiftUI.
By the end of this section, you’ll be able to create captivating and interactive animations to enrich the user experience of your SwiftUI app. Let’s animate!
Gestures & Interactions in SwiftUI
Welcome to the Gestures & Interactions in SwiftUI section. Interactions breathe life into your app, allowing users to engage with the interface in meaningful and intuitive ways. This part of the guide is dedicated to helping you understand and implement various gesture interactions within your SwiftUI applications.
Start your journey with some of the most common gestures in mobile applications: Detecting Taps in SwiftUI and Detecting Long Press Gestures in SwiftUI. Then, add more complex gestures, such as Implementing Dragging in SwiftUI and Implementing Swipe to Delete in SwiftUI.
You’ll then explore how to use gestures to manipulate the views themselves. Learn how to animate and interact with your views by Rotating Views with Gestures in SwiftUI and Implementing Pinch to Zoom in SwiftUI.
Managing gestures can get complex as your app grows, so understanding the concepts of Using Gesture Priority in SwiftUI, Sequencing Gestures in SwiftUI, Exclusive Gestures in SwiftUI, and Simultaneous Gestures in SwiftUI will allow you to build more responsive and intuitive user experiences.
By the end of this section, you’ll be well-equipped to create a SwiftUI application that responds fluidly to a variety of touch inputs. Let’s start interacting!
Text Input in SwiftUI
Welcome to the Text Input in SwiftUI section. Here, you’ll master the art of handling user text inputs in your SwiftUI applications, a fundamental aspect of creating interactive and responsive applications.
You’ll start off by learning how to Create a Text Field in SwiftUI, the most basic form of text input. Once you’re comfortable with that, you’ll venture into Creating a Text Editor in SwiftUI to accept multi-line text input from users.
To facilitate long text entries, you’ll explore how to Create A Scrollable Text Field in SwiftUI. You’ll also learn to accommodate optional input with the Create a Text Field with an Optional in SwiftUI chapter.
Next, make your input fields smarter and cleaner by learning to Format Text Input in a Text Field in SwiftUI. From here, delve into the aesthetics of text input fields by learning how to Style a Text Field and Style a Text Editor in SwiftUI.
For sensitive information, such as passwords, you’ll find the chapter on how to Hide User Input Using a SecureField in SwiftUI particularly useful.
Adding advanced features to your text editors can dramatically enhance user experience. Learn to Add Find and Replace to a TextEditor in SwiftUI for a more efficient text manipulation.
Finally, you’ll learn how to improve user experience by understanding how to Dismiss Keyboard on Scroll in SwiftUI, a common functionality in many apps.
By the end of this section, you’ll have all the skills necessary to create and customize dynamic text input fields in SwiftUI. Ready to start? Let’s go!
Creating Modal Views in SwiftUI
Welcome to the Creating Modal Views in SwiftUI section. Modals play an integral role in user interfaces, serving as contextual screens for alerts, options, or displaying additional information. This part of the guide aims to help you understand and implement a wide variety of modals within your SwiftUI applications.
You’ll start with the basics on how to Create a Modal View in SwiftUI and Dismiss a Modal View in SwiftUI. You’ll then explore how to Pass Data to a Modal View in SwiftUI, making your modals more dynamic and interactive.
The journey continues with understanding how to create different types of modals, like a Full Screen Modal View or a Popover in SwiftUI. You’ll also learn to tweak your modal’s appearance by Configuring the Modal View Height, Adding a List to a Modal, Customizing the Corner Radius of a Modal, and Setting a Custom Background for a Modal in SwiftUI.
Interaction is key, so you’ll discover how to Control Interaction with the View Behind a Modal in SwiftUI, allowing you to decide when and how users can interact with the underlying view.
Lastly, alerts and dialogues are vital for communicating with users. You’ll learn how to Create an Alert in SwiftUI, Handle Errors with an Alert, and Present a Confirmation Dialog in SwiftUI.
By the end of this section, you’ll be well-equipped to create and customize a variety of modal views in SwiftUI, enriching your app’s user experience. Let’s dive in!
Adding Audio & Video in SwiftUI
Welcome to the Adding Audio & Video in SwiftUI section. Here, you’ll learn how to enhance your SwiftUI applications with immersive multimedia experiences. Whether you’re creating a music player, a movie streaming app, or adding aural feedback to your interfaces, this part of the guide has got you covered.
You’ll kick off the section by creating the heart of any media application, with chapters on how to Create an Audio Player in SwiftUI and Create a Video Player in SwiftUI. Once you’ve mastered the basics, you’ll explore Customizing Audio & Video Playback in SwiftUI for a more personalized user experience.
Keeping your media accessible even when the app is not in focus is crucial. Learn the ins and outs of Playing Audio & Video in the Background in SwiftUI to ensure a seamless experience for your users.
Then, step into the realm of content creation by Recording Audio & Video in SwiftUI. Complement this with techniques for Adding Sound Effects in SwiftUI to make your app more engaging.
Next, embrace the age of streaming with the chapter on Implementing Video Streaming in SwiftUI. Then, bring your media app to life with Creating Animated Visualizations for Audio & Video in SwiftUI.
Accessibility is key, so you’ll also learn to Add Captions & Subtitles to Videos in SwiftUI. And, of course, things don’t always go as planned, so you’ll discover how to handle setbacks gracefully in Handling Errors & Exceptions While Playing Audio & Video in SwiftUI.
By the end of this section, you’ll be well-equipped to create engaging and dynamic multimedia experiences in your SwiftUI apps. Let’s dive in!
Building for Multiple Platforms with SwiftUI
Welcome to the Building for Multiple Platforms with SwiftUI section. SwiftUI’s power lies in its ability to seamlessly work across all Apple platforms. This part of the guide aims to give you the confidence and know-how to create SwiftUI apps that are truly multiplatform.
You’ll kick things off by exploring how to create applications for different Apple platforms, starting with Creating a macOS App with SwiftUI, then moving on to Creating a watchOS App with SwiftUI, Creating an iOS & iPadOS App with SwiftUI, and finally, Creating a tvOS App with SwiftUI.
From there, you’ll delve into building components that are universal across platforms with Creating Multiplatform Components in SwiftUI. You’ll also see how to Write Platform-Specific Code Using Conditional Compilation and Import Platform-Specific Frameworks Using Conditional Compilation.
Adjusting your app to suit different screen sizes is another crucial aspect of multiplatform development. You’ll master this in Adapting SwiftUI Layouts for Various Screen Sizes.
Next, learn to test your application on various devices and platforms in Testing Your SwiftUI App on Different Devices & Platforms.
Finally, you’ll discover how to extend the capabilities of your app by learning how to Use App Extensions in SwiftUI.
By the end of this section, you’ll be able to develop versatile apps that adapt and thrive on any Apple platform. Let’s get started!
Accessibility in SwiftUI
Welcome to the Accessibility in SwiftUI section. Inclusive design ensures that your apps are usable by as many people as possible. This part of the guide is all about making your SwiftUI applications accessible and user-friendly for all, including users with disabilities.
The journey begins with understanding how to Respond to Dynamic Type in SwiftUI for Accessibility, making sure your text sizes adapt to user preferences. You’ll also learn to enhance user experience with voice guidance by adding VoiceOver to SwiftUI Views.
Making your visual content accessible is equally important. Discover how to Make SwiftUI Images Accessible with Descriptions and how to Describe SwiftUI View Values for Accessibility for the optimal user experience.
Next, you’ll explore the methods to Add Custom Accessibility Content in SwiftUI Views and make your app more friendly to visually impaired users with Implementing Dark Mode Accessibility in SwiftUI.
Continue your learning by adding interactivity with Custom Accessibility Actions to SwiftUI Views. You’ll also get to grips with controlling how accessibility features interact with your views in Control Activation Points for Accessibility in SwiftUI.
VoiceOver is a powerful tool for accessibility, and you’ll learn how to tailor it to your needs in Tailor VoiceOver Speech Properties in SwiftUI. Finally, you’ll discover how to Navigate with Accessibility Rotors in SwiftUI, helping users to interact with your app more effectively using VoiceOver.
By the end of this section, you’ll be well-equipped to build SwiftUI applications that are truly inclusive, making the world of technology more accessible to everyone. Let’s dive in!
Localization & Internationalization in SwiftUI
Welcome to the Localization & Internationalization in SwiftUI section! In today’s global market, it’s vital to design applications that can adapt to different locales and languages. This part of the guide is dedicated to helping you make your SwiftUI applications accessible and user-friendly for a worldwide audience.
Your journey begins with understanding how to Create a Localized String in SwiftUI and Display the User’s Language in SwiftUI. You’ll then build upon these basics by mastering how to Interpolate Strings in SwiftUI Localization.
Next, you’ll learn to handle common internationalization tasks, such as localizing dates and numbers in Localize a Date in SwiftUI and Localize Numbers in SwiftUI. Additionally, you’ll see how to Test Your Localized SwiftUI App to ensure a seamless user experience across all languages and regions.
Localization doesn’t stop with text. You’ll also explore how to Localize Images in SwiftUI and accommodate right-to-left languages in Use RTL (Right to Left) Languages in SwiftUI.
Accessibility is a critical aspect of any app. In Localize Accessibility Labels & Hints in SwiftUI, you’ll learn to make your app more accessible for non-English speakers or users with disabilities.
Finally, you’ll learn how to Support Dynamic Type for Multilingual Text in SwiftUI to ensure your app’s typography responds appropriately to user preferences across languages.
By the end of this section, you’ll be well-equipped to build SwiftUI applications that are ready for global audiences. Let’s get started on this important journey to global inclusivity!
Deploying & Debugging SwiftUI Apps
Welcome to the Deploying & Debugging SwiftUI Apps section. It’s one thing to code an app, but getting it ready for deployment and effectively debugging it is another essential skill set. This section is here to guide you through that process and help you master the practical aspects of SwiftUI development.
The journey begins with Building & Running SwiftUI Apps in Xcode, laying the foundation for how you’ll test your app during development. Then, you’ll move on to Configuring the Deployment Target for SwiftUI Apps, to ensure your app works on the desired range of devices and operating systems.
You’ll also learn to give your app a professional look by Configuring App Icons & Launch Screens in SwiftUI and how to effectively Manage App Permissions for access to device features and user data.
Next, the focus will shift to debugging. You’ll discover how to make the most of Xcode’s debugging tools in Debugging SwiftUI Code with Xcode’s Debugger and Using the Console to Debug SwiftUI Apps.
One of the most powerful features of SwiftUI is the preview functionality. You’ll get to grips with this in Getting Started with SwiftUI Previews and Customizing SwiftUI Previews, to see your UI changes without the need for constant device or simulator testing.
Lastly, you’ll dive into identifying and fixing performance issues. Finding Memory Leaks Using Instruments will guide you in keeping your app’s memory usage efficient, and Understanding & Reducing Startup Time With Instruments will ensure your app launches quickly for the best user experience.
By the end of this section, you’ll have the know-how to deploy and debug your SwiftUI apps efficiently and effectively. Let’s get started!
Advanced Topics in SwiftUI
Welcome to the Advanced Topics in SwiftUI section. This part of the guide is dedicated to helping you delve deeper into the many advanced facets of SwiftUI, equipping you with the knowledge to handle complex tasks and create robust SwiftUI applications.
You’ll begin this section by learning how to Design a Seamless Onboarding Experience in SwiftUI, a crucial aspect of providing a welcoming user experience. Following this, we’ll explore how to Create & Customize Charts in SwiftUI With Swift Charts, enriching your applications with visually engaging data representations.
Our next stop is Create Complex UI With SwiftUI Shape & Path. This knowledge will arm you with the tools to create more complex, custom UI elements that make your applications stand out. From there, you’ll move onto integrating your SwiftUI applications with existing frameworks. Learn to Integrate SwiftUI With UIKit and Integrate Core Data With SwiftUI, broadening the scope of what your apps can do.
The section continues with a deep dive into modern, asynchronous programming techniques, specifically Async/Await in SwiftUI. You’ll also explore the power of Using Combine With SwiftUI to handle complex asynchronous events and data.
Later, you’ll be introduced to Unit Testing Strategies in SwiftUI, to ensure your code works as expected, and Testing SwiftUI Views With ViewInspector, to verify your UI looks and behaves as intended.
Lastly, you’ll tackle how to Implement MVVM Architecture in SwiftUI, a widely-accepted design pattern that can make your SwiftUI applications more maintainable and scalable.
By the end of this section, you’ll have amassed advanced skills that can dramatically elevate the quality and capabilities of your SwiftUI applications. Happy learning!
SwiftUI Best Practices & Tips
Welcome to the SwiftUI Best Practices & Tips section! In this valuable part of the guide, you’ll explore various best practices and tips for mastering SwiftUI, Apple’s innovative UI framework.
Kick off your journey by learning how to Improve SwiftUI Performance. This essential knowledge will ensure that the applications you create are as smooth and responsive as possible. Next, discover techniques to Reduce Complexity in SwiftUI Views, perfect for keeping larger applications manageable.
Master the art of clean, maintainable code by Separating View Logic with View Models. This approach will help you keep your code neatly structured and easier to understand. You’ll also uncover the benefits of Using Environment Variables in SwiftUI, enabling you to share data effectively across your SwiftUI views.
Explore the realm of dynamic and engaging UIs by Optimizing Animations in SwiftUI. This knowledge will ensure your animations always run smoothly, improving the overall user experience. Subsequently, grasp the intricacies of Maintaining State in SwiftUI to make sure your UI is consistently up-to-date and accurate.
Unearth powerful techniques for Debugging SwiftUI Views to solve those unexpected issues that inevitably arise during development. Boost your productivity further by learning how to Create Reusable SwiftUI Components and Use View Composition in SwiftUI, breaking down complex views into manageable, reusable pieces.
By the end of this section, you’ll have an arsenal of best practices and tips that will elevate your SwiftUI development skills. Dive in and let the journey begin!
Authors
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK