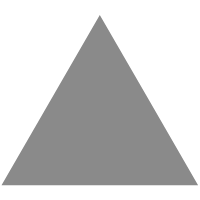
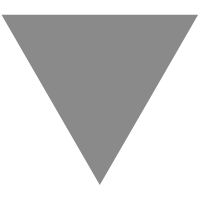
SAP Landscape Management Cloud (LaMa Cloud) REST API Sample
source link: https://blogs.sap.com/2023/06/21/sap-landscape-management-cloud-lama-cloud-rest-api-sample/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
SAP Landscape Management Cloud (LaMa Cloud) REST API Sample
Inbound REST API feature for LaMa Cloud was recently released and explained in the blog. Here I will show how to establish an API communication between LaMa Cloud and another application.
Sample is available at GitHub
API Configuration in LaMa Cloud
In order to use the API, you need to configure an API key. This is described in the LaMa Cloud Guide and the steps are summarized below:
- Go to Configuration > API Management
- Click Create and enter a name for the API Key
- Select the Scope (description)
- In this example we will not use X.509 certificate so it will be deselected

- Click Create and the generated JSON output should be saved as it contains important info that will not be displayed again

API Client Test Call
We will use postman to test the API call before we use it in our application. Below is the configuration of Postman used.
Authorization:
Type: OAuth2.0
Grant Type: Client Credentials
Access Token URL: Enter tokenurl from previous section
Client ID: Enter clientId from previous section
Client Secret: Enter clientSecret from previous section
Scope: blank
Client Authentication: Send as Basic Auth header
With the above click on Get Access Token and then use it.
GET URL:
Enter the “url” from the JSON generated in the previous section and append “/systems”
Clicking on Send should produce an output in JSON format such as below:

API Calls From A Sample Application (local machine)
Now that we have tested that we can make a GET call via Postman to retrieve Systems, we can now make the same call from a test application. For this I created a very simple Python web application. This will use the same OAuth2.0 authentication and using the token to then retrieve the Systems in JSON format. In the web app we will filter the JSON results and display only System names in a tabular form.
Sample code of this application can be found at GitHub and also shown below.
server.py
import os
import requests
from flask import Flask, jsonify, request, render_template
import json
from tabulate import tabulate
app = Flask(__name__)
port = int(os.environ.get('PORT', 3000))
# OAuth 2 client configuration
client_id = "Enter your clientId"
client_secret = "Enter your clientSecret"
token_url = 'Enter tokenUrl from LaMa Cloud API Config'
api_url = '<Enter url from LaMa Cloud API Config>/systems'
# Get access token using client credentials
def get_access_token():
payload = {
'grant_type': 'client_credentials',
'client_id': client_id,
'client_secret': client_secret
}
response = requests.post(token_url, data=payload)
access_token = response.json()['access_token']
return access_token
# Recursive function to extract "name" entries from JSON data
def extract_names(data):
names = []
if isinstance(data, dict):
if "group" in data:
del data["group"] # Exclude the "group" section
for key, value in data.items():
if key == "name":
names.append(value)
elif isinstance(value, (dict, list)):
names.extend(extract_names(value))
elif isinstance(data, list):
for item in data:
names.extend(extract_names(item))
return names
@app.route("/")
def hello_world():
return "Hello, World!"
@app.route('/api/v1/systems', methods=['GET'])
def get_data():
access_token = get_access_token()
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json'
}
response = requests.get(api_url, headers=headers)
data = response.json()
names = extract_names(data)
# Create a list of lists containing the names
table_data = [[name] for name in names]
# Generate the HTML table with borders
table_html = tabulate(table_data, headers=["SYSTEMS"], tablefmt="html")
# Render the template with the table
return render_template('table.html', table_html=table_html)
if __name__ == '__main__':
app.run(host='0.0.0.0', port=port)
templates/table.html:
<!DOCTYPE html>
<html>
<head>
<title>GET Call to LaMa Cloud</title>
<style>
table {
border-collapse: collapse;
}
th, td {
border: 1px solid black;
padding: 8px;
}
</style>
</head>
<body>
<h1>GET Call to LaMa Cloud</h1>
{{ table_html | safe }}
</body>
</html>
We also need to install some libraries that we are using in the Python script.
% pip install flask
% pip install tabulate
Now run the Python script as follows:
- Go to the directory where the above python script is located. Make sure that you have also created a sub directory called templates and in it you have the table.html file.
- On your system, issue the below command
% python server.py
- Open a browser on the same machine and go to URL “http://127.0.0.1:3000/api/v1/systems” and you should see a table such as below
- Alternatively you can also use the IP address of the machine and then it will work from a remote browser as well

This shows that from the web app, we were able to retrieve a token and then use this to make a GET call to /systems. This resulted in a JSON output from LaMa Cloud that we were then able to format to display as above.
API Calls From A Sample Application (in SAP BTP Cloud Foundry)
We will now run the same python web application in SAP BTP and using the Cloud Foundry runtime. You need the following prerequisites met:
- You have a trial or productive account for SAP BTP. A new trial account can be created via try out services for free.
- You have created a subaccount and a space on Cloud Foundry Environment.
- Install npm (refer to this site)
- Install the Cloud Foundry CLI by (refer to this guide)
- Install virtualenv
% pip install virtualenv
In the directory where the Python script resides, create the following additional files:
manifest.yml
---
applications:
- name: myapp
random-route: true
path: ./
memory: 128M
buildpack: python_buildpack
command: python server.py
requirements.txt
Flask==2.0.1
flask-restful
flask
requests
tabulate
runtime.txt (adjust to reflect your version)
python-3.10.11
Execute below commands
% cf api https://api.cf.us10-001.hana.ondemand.com (change to your own region URL)
% cf login
% cf push
In the output of the “cf push” command note down the URL listed for routes.

Now you can test this by going to the URL: https://<URL from the cf push>/api/v1/systems. You should get the same output as in previous section.

As you can see, we successfully made the API call to LaMa Cloud from an SAP BTP hosted application.
You have now learnt the basics of how to make RESTful API calls to LaMa Cloud.
References
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK