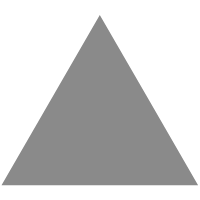
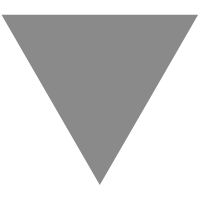
Remult, a Crud Framework for Fullstack Typescript
source link: https://www.infoq.com/news/2023/05/remult-crud-typescript/?itm_source=infoq&itm_medium=popular_widget&itm_campaign=popular_content_list&itm_content=
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
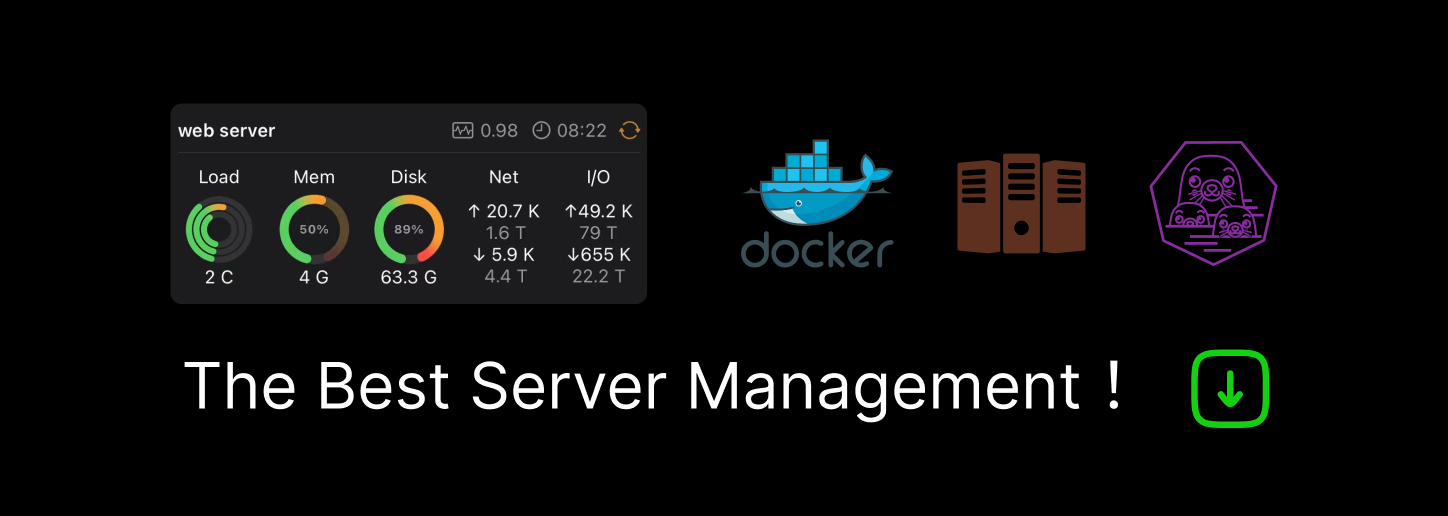
Remult, a Crud Framework for Fullstack Typescript
May 08, 2023 2 min read
Remult is a full-stack CRUD library that simplifies development by leveraging TypeScript models, providing a type-safe API client and query builder.
In software development, two data models must be managed and synchronized to ensure proper system functionality: server and client models. Server models specify the structure of the database and API, while client models define the data transmitted to and from the API.
However, maintaining separate sets of models and validators can result in redundancy, increased maintenance overhead, and the potential for errors when the models become out of sync.
Remult solves this problem by providing an integrated model that defines the database schema, exposes simple CRUD APIs, and supports client-side integration that enables developers to query the database, all while maintaining type safety easily.
Defining Entities
Remult utilizes decorators to transform basic JavaScript classes into Remult Entities. Developers can accomplish this easily by adding the Entity decorator to the class and applying the relevant field decorators to each property.
Using decorators, Remult simplifies the process of creating entities and their associated fields, making it more efficient and intuitive for developers.
import { Entity, Fields } from "remult"
@Entity("contacts", {
allowApiCrud: true
})
export class Contact {
@Fields.autoIncrement()
id = 0
@Fields.string()
name = ""
@Fields.string()
number = ""
}
Server Side Setup
To start using Remult, register it alongside the necessary entities with the chosen server.
Fortunately, Remult provides out-of-the-box integrations for several popular server frameworks, including Express, Fastify, Next.js, Nest, and Koa.
import express from "express";
import { remultExpress } from "remult/remult-express";
import Contact from "../shared/Contact.ts";
const app = express();
app.use(
remultExpress({
entities: [
Contact
]
})
);
Client Side Integration
After configuring the backend and entities, the next step is integrating Remult with the application's front end.
Fortunately, Remult's client integration is designed to be library agnostic, meaning it can operate using browser fetch capabilities or Axios.
To illustrate this functionality, consider the following example:
import { useEffect, useState } from "react"
import { remult } from "remult"
import { Contact } from "./shared/Contact"
const contactsRepo = remult.repo(Contact)
export default function App() {
const [contacts, setContacts] = useState<Contact[]>([])
useEffect(() => {
contactsRepo.find().then(setContact)
}, [])
return (
<div>
<h1>Contacts</h1>
<ul>
{contacts.map(contact => {
return (
<div key={contact.id}>
{contact.name} | {contact.phone}
</div>
)
})}
</ul>
</div>
)
}
This example demonstrates the ease and flexibility with which Remult can be incorporated into the front end of an application, allowing developers to seamlessly leverage the power and functionality of Remult across the entire stack.
Remult is open-source software available under the MIT license. Contributions are welcome via the Remult GitHub repository.
About the Author
Guy Nesher
Developer at Locusview focusing on web technologies and active speaker/meetup organizer.
Recommend
-
100
Ripple Fullstack On the server: index.js const ripple = require('rijs')({ dir: __dirname }) On the client: pages/index.html ...
-
67
README.md FullStack This is a platform I began building for a client. After he signed and I started working he decided to pivot and not pay me. Sometim...
-
35
-
10
Angular CRUD With ASP.NET Core And Entity Framework Core It feels great to be back with this, my 18th article on Angular with the recently updated Angular 7. With this article, let’s try to explore the Angular 7 features a...
-
20
Files Permalink Latest commit message Commit time
-
5
Aug 21st, 2019Potluck - Deploying Applications × Typescript × Live Coding with Twitch × Fullstack Architecture × More!👇 Download Show
-
6
In this article, we will do a small demo of the AspNetCore 6 Razor Pages CRUD operation.Razor Pages:Razor Page is a simplified web application model. On comparing with the 'MVC' template, razor pages won't have 'Controller', w...
-
8
Remult What is Remult? Remult is a full-stack CRUD framework that uses your TypeScript model types to provide: Secure REST API (highly configurable) Type-sa...
-
11
withtyped Note This project is in the experimental stage and has a lot of work to do. Don't use it in prod.
-
8
garph Screen.Recording.2023-02-27.at.19.32.45.mov Warning: We would love to hear your Feedback on our Discord
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK