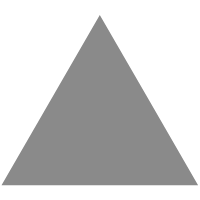
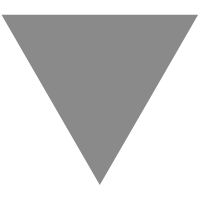
GitHub - yazeedobaid/openai-fsharp: OpenAI - F# - A library that allows you to i...
source link: https://github.com/yazeedobaid/openai-fsharp
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
OpenAI F# is a library that allows you to interact with Open AI API in an easy, fluent, and typed way!
Get Started
Add the library to your project using DotNet CLI:
dotnet add package OpenAI.Client
Or using Paket:
paket add OpenAI.Client
The library provide two styles to consume the APIs, either a methods calls through pipe operator as shown below:
open OpenAI
open OpenAI.Client
open OpenAI.Models
let client =
Config(
{ Endpoint = "https://api.openai.com/v1"
ApiKey = "your-api-key" },
HttpRequester()
)
let result = client |> models |> list
Or using a computation expression builder object as shown below:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
models
list
}
You can check OpenAI API reference at API reference.
Usage
Models
Resource
List and describe the various models available in the API. You can refer to the Models documentation to understand what models are available and the differences between them.
list
Lists the currently available models, and provides basic information about each one such as the owner and availability.
let result = client |> models |> list
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
models
list
}
retrieve
Retrieves a model instance, providing basic information about the model such as the owner and permissioning.
let result = client |> models |> retrieve "text-davinci-003"
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
models
retrieve "text-davinci-003"
}
delete
Delete a fine-tuned model.
let result = client |> models |> delete "curie:ft-acmeco-2021-03-03-21-44-20"
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
models
delete "curie:ft-acmeco-2021-03-03-21-44-20"
}
Completions
Resource
Given a prompt, the model will return one or more predicted completions, and can also return the probabilities of alternative tokens at each position.
create
Creates a completion for the provided prompt and parameters.
let result =
client
|> completions
|> Completions.create
{ Model = "text-davinci-003"
Prompt = "What is the meaning of living?"
Temperature = 0.5
Stop = "." }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
completions
create
{ Model = "text-davinci-003"
Prompt = "What is the meaning of living?"
Temperature = 0.5
Stop = "." }
}
Edits
Resource
Given a prompt and an instruction, the model will return an edited version of the prompt.
create
Creates a new edit for the provided input, instruction, and parameters.
let result =
client
|> edits
|> Edits.create
{ Model = "text-davinci-edit-001"
Input = "What day of the wek is it?"
Instruction = "Fix the spelling mistakes" }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
edits
create
{ Model = "text-davinci-edit-001"
Input = "What day of the wek is it?"
Instruction = "Fix the spelling mistakes" }
}
Images
Resource
Given a prompt and/or an input image, the model will generate a new image.
create
Creates an image given a prompt.
let result =
client
|> images
|> create
{ Prompt = "A cute baby sea otter"
N = 2
Size = "1024x1024"
responseFormat = "url" }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
images
create
{ Prompt = "A cute baby sea otter"
N = 2
Size = "1024x1024"
responseFormat = "url" }
}
edit
Creates an edited or extended image given an original image and a prompt.
let result =
client
|> images
|> edit
{ Image = "sample.png"
Mask = "sample.png"
Prompt = "A cute baby sea otter wearing a beret"
N = 2
Size = "1024x1024"
responseFormat = "url" }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
images
edit
{ Image = "sample.png"
Mask = "sample.png"
Prompt = "A cute baby sea otter wearing a beret"
N = 2
Size = "1024x1024"
responseFormat = "url" }
}
variation
Creates a variation of a given image.
let result =
client
|> images
|> variation
{ Image = "sample.png"
N = 2
Size = "1024x1024"
responseFormat = "url" }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
images
variation
{ Image = "sample.png"
N = 2
Size = "1024x1024"
responseFormat = "url" }
}
Embeddings
Resource
Get a vector representation of a given input that can be easily consumed by machine learning models and algorithms.
create
Creates an embedding vector representing the input text.
let result =
client
|> embeddings
|> create
{ Model = "text-embedding-ada-002"
Input = "The food was delicious and the waiter..." }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
embeddings
create
{ Model = "text-embedding-ada-002"
Input = "The food was delicious and the waiter..." }
}
Files
Resource
Files are used to upload documents that can be used with features like Fine-tuning.
list
Returns a list of files that belong to the user's organization.
let result = client |> files |> list
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
files
list
}
upload
Upload a file that contains document(s) to be used across various endpoints/features.
let result =
client
|> files
|> upload
{ File = @"sample-json.txt"
Purpose = "fine-tune" }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
files
upload
{ File = @"sample-json.txt"
Purpose = "fine-tune" }
}
delete
Delete a file.
let result =
client
|> files
|> delete "file-qtUwySute1Zf2yT6mWIGTCwq"
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
files
delete "file-qtUwySute1Zf2yT6mWIGTCwq"
}
retrieve
Returns information about a specific file.
let result = client |> files |> retrieve "file-Lpe0n5tOHtoG6OVVbk5d4iXA"
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
files
retrieve "file-Lpe0n5tOHtoG6OVVbk5d4iXA"
}
download
Returns the contents of the specified file.
let result = client |> files |> download "file-Lpe0n5tOHtoG6OVVbk5d4iXA"
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
files
download "file-Lpe0n5tOHtoG6OVVbk5d4iXA"
}
FineTunes
Resource
Manage fine-tuning jobs to tailor a model to your specific training data.
create
Creates a job that fine-tunes a specified model from a given dataset.
let result =
client
|> fineTunes
|> create { CreateRequest.Default with TrainingFile = "file-Lpe0n5tOHtoG6OVVbk5d4iXA" }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
fineTunes
create { CreateRequest.Default with TrainingFile = "file-Lpe0n5tOHtoG6OVVbk5d4iXA" }
}
list
List your organization's fine-tuning jobs.
let result = client |> fineTunes |> list
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
fineTunes
list
}
retrieve
Gets info about the fine-tune job.
let result = client |> fineTunes |> retrieve "ft-kNJeZjAhlmFLdCuIzcpkrmNI"
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
retrieve
retrieve "ft-kNJeZjAhlmFLdCuIzcpkrmNI"
}
cancel
Immediately cancel a fine-tune job.
let result = client |> fineTunes |> cancel "ft-kNJeZjAhlmFLdCuIzcpkrmNI"
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
fineTunes
cancel "ft-kNJeZjAhlmFLdCuIzcpkrmNI"
}
list events
Get fine-grained status updates for a fine-tune job.
let result = client |> fineTunes |> listEvents "ft-kNJeZjAhlmFLdCuIzcpkrmNI"
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
fineTunes
listEvents "ft-kNJeZjAhlmFLdCuIzcpkrmNI"
}
Moderations
Resource
Given a input text, outputs if the model classifies it as violating OpenAI's content policy.
create
Classifies if text violates OpenAI's Content Policy.
let result =
client
|> moderations
|> create
{ Model = "text-moderation-latest"
Input = "I want to kill them." }
Or using a computation expression builder:
let result =
openAI {
endPoint "https://api.openai.com/v1"
apiKey "your-api-key"
moderations
create
{ Model = "text-moderation-latest"
Input = "I want to kill them." }
}
Credits
This library was inspired from openai-php and FsHttp.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK