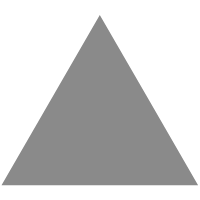
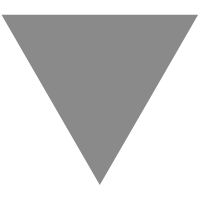
How to get and set Value in Form Fields in Javascript
source link: https://www.laravelcode.com/post/how-to-get-and-set-value-in-form-fields-in-javascript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
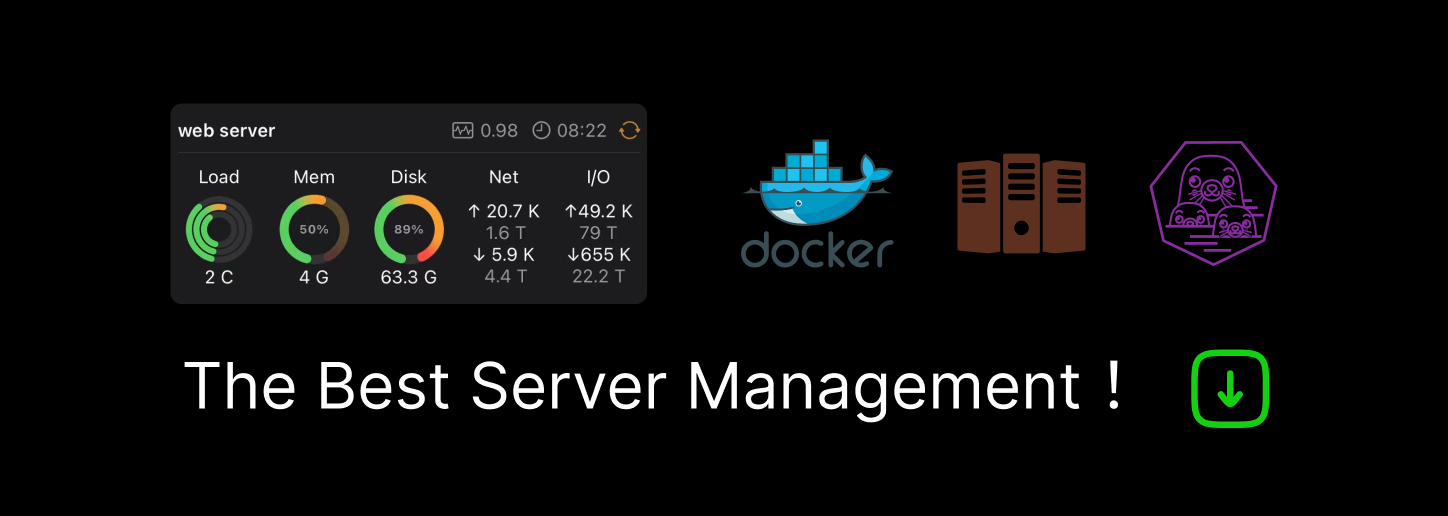
How to get and set Value in Form Fields in Javascript
In this article, we will discuss on setting and getting value from input and textarea. These elements contains value and not text. So first, let's starting with getting value from input field.
Let's get value from input field.
Example:
<input type="text" id="message" name="message" value="Hello World!">
<script type="text/javascript">
var data = document.getElementById('message').value;
console.log(data);
</script>
Same way we can also set value in the field. Here is example of textarea field.
Example:
<textarea id="message" name="message">Hello World!</textarea>
<script type="text/javascript">
var data = document.getElementById('message').value = 'Hello Earth!';
console.log(data);
// Hello Earth!
</script>
This will change text value in textarea field. Now let's look at how to get value from radio button. This is something different than textarea or input field. In the radio button, we have multiple input but we only need the checked input value.
Example:
<input type="radio" id="male" name="gender" value="male"><label for="male">Male</label>
<input type="radio" id="female" name="gender" value="female"><label for="female">Female</label>
<button onclick="checked()">Click</button>
<script type="text/javascript">
function checked() {
var data = document.querySelector("input[name=gender]:checked").value;
console.log(data);
}
</script>
In the checkbox field, value is already there, all we have to do is to check whether the checkbox is checked or unchecked. checked property will return true if element is checked. This is how we can do it.
Example:
<input type="checkbox" id="remember" name="remember" value="true"><label for="remember">Remember me?</label>
<button onclick="checked()">Click</button>
<script type="text/javascript">
function checked() {
if (document.querySelector("input[name=remember]").checked) {
var element = document.querySelector("input[name=remember]").value;
console.log(element);
} else {
console.log(false);
}
}
</script>
Conclusion
So far in this article, we have learned how to get and set value to different input and textarea.
I hope this will help you.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
-
19
Using JavaScript in PDF Form Fields
-
5
PHP: Do not Email Form Fields Left in White advertisements Currently I am making an online enquiry form with a set of fields that are non-mand...
-
5
Best practice for date-of-birth form fieldsWhat the evidence says vs. OS pattern libraries
-
12
You can't capture the nuance of my form fields June 27, 2021 on Drew DeVault's blog Check out this text box: Here are some of the nuances of using this tex...
-
6
Combine Form Fields, Merge Fields and Signature Boxes to Request Signatures The electronic signature best practices demo Text Control eSign shows how to use TX Text Control to integrate electronic signature processes into your a...
-
14
Publish non-typing fields in a form? advertisements This seems like a simple thing, and maybe I'm just not thinking straight today, but right now I...
-
10
PrestaShop: adding new fields to the customer form In this article, I will demonstrate how to edit, add, and mo...
-
3
Create Dynamic Form Fields in ReactA few days ago I needed to implement dynamic form fields for a project in React. I thought it would be good to make a quick tutorial on this, so here it is!Let’s say we are buildin...
-
9
Events and disabled form fieldsPosted 17 February 2017 I've been working on the web since I was a small child all the way through to the haggard old man I am to day. However, the web still continues to surprise me. Turns...
-
7
July 18, 2023Alpine.js and Form Fields
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK