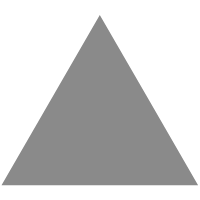
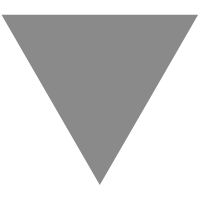
How To Add Three Photo Filters to Your Applications in Java
source link: https://dzone.com/articles/how-to-add-three-photo-filters-to-your-application
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How To Add Three Photo Filters to Your Applications in Java
In this article, learn how developers can implement robust image customization features with only a few lines of clean, simple code.
A unique image aesthetic makes a big difference in representing any personal or professional brand online. Career and hobby photographers, marketing executives, and casual social media patrons alike are in constant pursuit of easily distinguishable visual content, and this basic need to stand out from a crowd has, in turn, driven the democratization of photo editing and filtering services in the last decade or so. Nearly every social media platform you can think of (not to mention many e-commerce websites and various other casual sites where images are frequently uploaded) now incorporates some means for programmatically altering vanilla image files. These built-in services can vary greatly in complexity, ranging from simple brightness controls to gaussian blurs.
With this newfound ease of access to photo filtering, classic image filtering techniques have experienced a widespread resurgence in popularity. For example, the timeless look associated with black and white images can now be hastily applied to any image upload on the fly. Through simple manipulations of brightness and contrast, the illusion of embossment can be harnessed, allowing us to effortlessly emulate a vaunted, centuries-old printing technique. Even posterization – a classic, bold aesthetic once humbly associated with the natural color limitations of early printing machines – can be instantly generated within any grid of pixels.
Given the desirability of simplified image filtering (especially those with common-sense customization features), building these types of features into any application – especially those handling a large volume of image uploads – is an excellent idea for developers to consider. Of course, once we elect to go in that direction, an important question arises: how can we efficiently include these services in our applications, given the myriad lines of code associated with building even the simplest photo-filtering functionality?
Thankfully, that question is answered yet again by supply and demand forces brought about naturally in the ongoing digital industrial revolution. Developers can rely on readily available Image Filtering API services to circumvent large-scale programming operations, thereby implementing robust image customization features in only a few lines of clean, simple code.
API Descriptions
The purpose of this article is to demonstrate three free-to-use image filtering API solutions which can be implemented into your applications using complementary, ready-to-run Java code snippets. These snippets are supplied below in this article, directly following brief instructions to help you install the SDK. Before we reach that point, I’ll first highlight each solution, providing a more detailed look at its respective uses and API request parameters. Please note that each API will require a free-tier Cloudmersive API key to complete your call (provides a limit of 800 API calls per month with no commitments).
Grayscale (Black and White) Filter API
Early photography was initially limited to a grayscale color spectrum due to the natural constraints of primitive photo technology. The genesis of color photography opened new doors, certainly, but it never completely replaced the black-and-white photo aesthetic. Even now, in the digital age, grayscale photos continue to offer a certain degree of depth and expression which many feel the broader color spectrum can’t bring out.
The process of converting a color image to grayscale is straightforward. Color information is stored in the hundreds (or thousands) of pixels making up any digital image; grayscale conversion forces each pixel to ignore its color information and present varying degrees of brightness instead. Beyond its well-documented aesthetic effects, grayscale conversion offers practical benefits, too, by reducing the size of the image in question. Grayscale images are much easier to store, edit and subsequently process (especially in downstream operations such as Optical Character Recognition, for example).
The grayscale filter API below performs a simple black-and-white conversion, requiring only an image’s file path (formats like PNG and JPG are accepted) in its request parameters.
Embossment Filter API
Embossment is a physical printing process with roots dating as far back as the 15th century, and it’s still used to this day in that same context. While true embossment entails the inclusion of physically raised shapes on an otherwise flat surface (offering an enhanced visual and tactile experience), digital embossment merely emulates this effect by manipulating brightness and contrast in key areas around the subject of a photo.
An embossment photo filter can be used to quickly add depth to any image. The embossment filter API below performs a customizable digital embossment operation, requiring the following input request information:
- Radius: The radius of pixels of the embossment operation (larger values will produce a greater effect)
- Sigma: The variance of the embossment operation (higher values produce higher variance)
- Image file: The file path for the subject of the operation (supports common formats like PNG and JPG)
Posterization API
Given the ubiquity of high-quality smartphone cameras, it’s easy to take the prevalence of high-definition color photos for granted. The color detail we’re accustomed to seeing in everyday photos comes down to advancements in high-quality pixel storage. Slight variations in reds, blues, greens, and other elements on the color spectrum are mostly accounted for in a vast matrix of pixel coordinates. In comparison, during the bygone era of physical printing presses, the variety of colors used to form an image was typically far less varied, and digital posterization filters aim to emulate this old-school effect. It does so by reducing the volume of unique colors in an image, narrowing a distinct spectrum of hex values into a more homogenous group. The aesthetic effect is unmistakable, invoking a look one might have associated with political campaigns and movie theater posters in decades past.
The posterization API provided below requires a user to provide the following request information:
- Levels: The number of unique colors which should be retained in the output image
- Image File: The image file to perform the operation on (supports common formats like PNG and JPG)
API Demonstration
To structure your API call to any of the three services outlined above, your first step is to install the Java SDK. To do so with Maven, first, add a reference to the repository in pom.xml:
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
Then add one to the dependency in pom.xml:
<dependencies>
<dependency>
<groupId>com.github.Cloudmersive</groupId>
<artifactId>Cloudmersive.APIClient.Java</artifactId>
<version>v4.25</version>
</dependency>
</dependencies>
Alternatively, to install with Gradle, add it to your root build.gradle (at the end of repositories):
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Then add the dependency in build.gradle:
dependencies {
implementation 'com.github.Cloudmersive:Cloudmersive.APIClient.Java:v4.25'
}
To use the Grayscale Filter API, use the following code to structure your API call:
// Import classes:
//import com.cloudmersive.client.invoker.ApiClient;
//import com.cloudmersive.client.invoker.ApiException;
//import com.cloudmersive.client.invoker.Configuration;
//import com.cloudmersive.client.invoker.auth.*;
//import com.cloudmersive.client.FilterApi;
ApiClient defaultClient = Configuration.getDefaultApiClient();
// Configure API key authorization: Apikey
ApiKeyAuth Apikey = (ApiKeyAuth) defaultClient.getAuthentication("Apikey");
Apikey.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Apikey.setApiKeyPrefix("Token");
FilterApi apiInstance = new FilterApi();
File imageFile = new File("/path/to/inputfile"); // File | Image file to perform the operation on. Common file formats such as PNG, JPEG are supported.
try {
byte[] result = apiInstance.filterBlackAndWhite(imageFile);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling FilterApi#filterBlackAndWhite");
e.printStackTrace();
}
To use the Embossment Filter API, use the below code instead (remembering to configure your radius and sigma in their respective parameters):
// Import classes:
//import com.cloudmersive.client.invoker.ApiClient;
//import com.cloudmersive.client.invoker.ApiException;
//import com.cloudmersive.client.invoker.Configuration;
//import com.cloudmersive.client.invoker.auth.*;
//import com.cloudmersive.client.FilterApi;
ApiClient defaultClient = Configuration.getDefaultApiClient();
// Configure API key authorization: Apikey
ApiKeyAuth Apikey = (ApiKeyAuth) defaultClient.getAuthentication("Apikey");
Apikey.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Apikey.setApiKeyPrefix("Token");
FilterApi apiInstance = new FilterApi();
Integer radius = 56; // Integer | Radius in pixels of the emboss operation; a larger radius will produce a greater effect
Integer sigma = 56; // Integer | Sigma, or variance, of the emboss operation
File imageFile = new File("/path/to/inputfile"); // File | Image file to perform the operation on. Common file formats such as PNG, JPEG are supported.
try {
byte[] result = apiInstance.filterEmboss(radius, sigma, imageFile);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling FilterApi#filterEmboss");
e.printStackTrace();
}
Finally, to use the Posterization Filter API, use the below code (remember to define your posterization level with an integer as previously described):
// Import classes:
//import com.cloudmersive.client.invoker.ApiClient;
//import com.cloudmersive.client.invoker.ApiException;
//import com.cloudmersive.client.invoker.Configuration;
//import com.cloudmersive.client.invoker.auth.*;
//import com.cloudmersive.client.FilterApi;
ApiClient defaultClient = Configuration.getDefaultApiClient();
// Configure API key authorization: Apikey
ApiKeyAuth Apikey = (ApiKeyAuth) defaultClient.getAuthentication("Apikey");
Apikey.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Apikey.setApiKeyPrefix("Token");
FilterApi apiInstance = new FilterApi();
Integer levels = 56; // Integer | Number of unique colors to retain in the output image
File imageFile = new File("/path/to/inputfile"); // File | Image file to perform the operation on. Common file formats such as PNG, JPEG are supported.
try {
byte[] result = apiInstance.filterPosterize(levels, imageFile);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling FilterApi#filterPosterize");
e.printStackTrace();
}
When using the Embossment and Posterization APIs, I recommend experimenting with different radius, sigma, and level values to find the right balance.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK