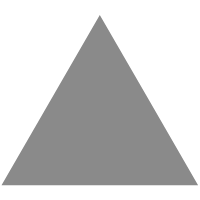
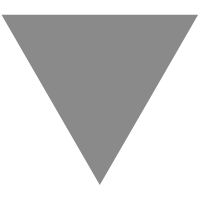
JAVA通过HttpClient发送HTTP请求
source link: https://blog.51cto.com/sourcebyte/5813961
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
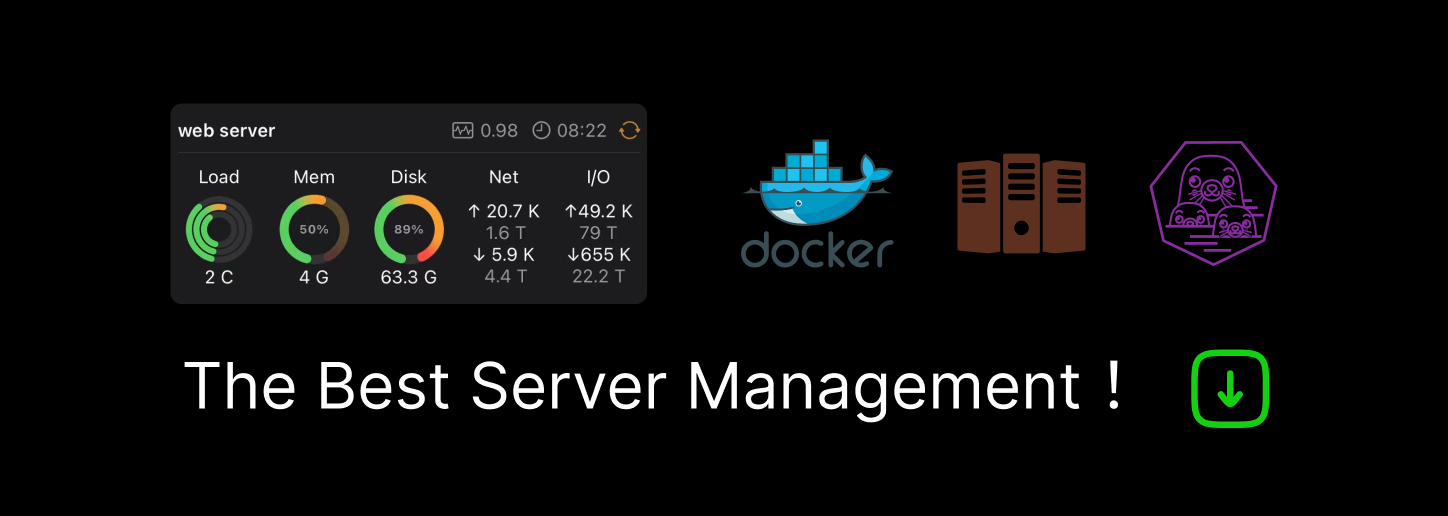
JAVA通过HttpClient发送HTTP请求_开源字节的技术博客_51CTO博客
第一步:引入Maven依赖
第二步:编写工具类
import java.io.File;
import java.io.IOException;
import java.security.KeyManagementException;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.apache.http.HttpEntity;
import org.apache.http.HttpStatus;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.config.Registry;
import org.apache.http.config.RegistryBuilder;
import org.apache.http.conn.socket.ConnectionSocketFactory;
import org.apache.http.conn.socket.PlainConnectionSocketFactory;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.conn.ssl.SSLContextBuilder;
import org.apache.http.conn.ssl.TrustSelfSignedStrategy;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.entity.mime.content.StringBody;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.DefaultHttpRequestRetryHandler;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import org.apache.http.util.EntityUtils;
/**
*
* @author zy
* @date 2021年11月05日 上午11:27:25
*
*/
public class HttpClientUtil {
// utf-8字符编码
public static final String CHARSET_UTF_8 = "utf-8";
// HTTP内容类型。
public static final String CONTENT_TYPE_TEXT_HTML = "text/xml";
// HTTP内容类型。相当于form表单的形式,提交数据
public static final String CONTENT_TYPE_FORM_URL = "application/x-www-form-urlencoded";
// HTTP内容类型。相当于form表单的形式,提交数据
public static final String CONTENT_TYPE_JSON_URL = "application/json;charset=utf-8";
// 连接管理器
private static PoolingHttpClientConnectionManager pool;
// 请求配置
private static RequestConfig requestConfig;
static {
try {
SSLContextBuilder builder = new SSLContextBuilder();
builder.loadTrustMaterial(null, new TrustSelfSignedStrategy());
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(
builder.build());
// 配置同时支持 HTTP 和 HTPPS
Registry<ConnectionSocketFactory> socketFactoryRegistry = RegistryBuilder.<ConnectionSocketFactory> create().register(
"http", PlainConnectionSocketFactory.getSocketFactory()).register(
"https", sslsf).build();
// 初始化连接管理器
pool = new PoolingHttpClientConnectionManager(
socketFactoryRegistry);
// 将最大连接数增加到200
pool.setMaxTotal(200);
// 设置最大路由
pool.setDefaultMaxPerRoute(2);
// 根据默认超时限制初始化requestConfig
int socketTimeout = 10000;
int connectTimeout = 10000;
int connectionRequestTimeout = 10000;
requestConfig = RequestConfig.custom().setConnectionRequestTimeout(
connectionRequestTimeout).setSocketTimeout(socketTimeout).setConnectTimeout(
connectTimeout).build();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (KeyStoreException e) {
e.printStackTrace();
} catch (KeyManagementException e) {
e.printStackTrace();
}
// 设置请求超时时间
requestConfig = RequestConfig.custom().setSocketTimeout(50000).setConnectTimeout(50000)
.setConnectionRequestTimeout(50000).build();
}
public static CloseableHttpClient getHttpClient() {
CloseableHttpClient httpClient = HttpClients.custom()
// 设置连接池管理
.setConnectionManager(pool)
// 设置请求配置
.setDefaultRequestConfig(requestConfig)
// 设置重试次数
.setRetryHandler(new DefaultHttpRequestRetryHandler(0, false))
.build();
return httpClient;
}
/**
* 发送Post请求
*
* @param httpPost
* @return
*/
private static String sendHttpPost(HttpPost httpPost) {
CloseableHttpClient httpClient = null;
CloseableHttpResponse response = null;
// 响应内容
String responseContent = null;
try {
// 创建默认的httpClient实例.
httpClient = getHttpClient();
// 配置请求信息
httpPost.setConfig(requestConfig);
// 执行请求
response = httpClient.execute(httpPost);
// 得到响应实例
HttpEntity entity = response.getEntity();
// 可以获得响应头
// Header[] headers = response.getHeaders(HttpHeaders.CONTENT_TYPE);
// for (Header header : headers) {
// System.out.println(header.getName());
// }
// 得到响应类型
// System.out.println(ContentType.getOrDefault(response.getEntity()).getMimeType());
// 判断响应状态
if (response.getStatusLine().getStatusCode() >= 300) {
throw new Exception(
"HTTP Request is not success, Response code is " + response.getStatusLine().getStatusCode());
}
if (HttpStatus.SC_OK == response.getStatusLine().getStatusCode()) {
responseContent = EntityUtils.toString(entity, CHARSET_UTF_8);
EntityUtils.consume(entity);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
// 释放资源
if (response != null) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return responseContent;
}
/**
* 发送Get请求
*
* @param httpGet
* @return
*/
private static String sendHttpGet(HttpGet httpGet) {
CloseableHttpClient httpClient = null;
CloseableHttpResponse response = null;
// 响应内容
String responseContent = null;
try {
// 创建默认的httpClient实例.
httpClient = getHttpClient();
// 配置请求信息
httpGet.setConfig(requestConfig);
// 执行请求
response = httpClient.execute(httpGet);
// 得到响应实例
HttpEntity entity = response.getEntity();
// 可以获得响应头
// Header[] headers = response.getHeaders(HttpHeaders.CONTENT_TYPE);
// for (Header header : headers) {
// System.out.println(header.getName());
// }
// 得到响应类型
// System.out.println(ContentType.getOrDefault(response.getEntity()).getMimeType());
// 判断响应状态
if (response.getStatusLine().getStatusCode() >= 300) {
throw new Exception(
"HTTP Request is not success, Response code is " + response.getStatusLine().getStatusCode());
}
if (HttpStatus.SC_OK == response.getStatusLine().getStatusCode()) {
responseContent = EntityUtils.toString(entity, CHARSET_UTF_8);
EntityUtils.consume(entity);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
// 释放资源
if (response != null) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return responseContent;
}
/**
* 发送 post请求
*
* @param httpUrl
* 地址
*/
public static String sendHttpPost(String httpUrl) {
// 创建httpPost
HttpPost httpPost = new HttpPost(httpUrl);
return sendHttpPost(httpPost);
}
/**
* 发送 get请求
*
* @param httpUrl
*/
public static String sendHttpGet(String httpUrl) {
// 创建get请求
HttpGet httpGet = new HttpGet(httpUrl);
return sendHttpGet(httpGet);
}
/**
* 发送 post请求(带文件)
*
* @param httpUrl
* 地址
* @param maps
* 参数
* @param fileLists
* 附件
*/
public static String sendHttpPost(String httpUrl, Map<String, String> maps, List<File> fileLists) {
HttpPost httpPost = new HttpPost(httpUrl);// 创建httpPost
MultipartEntityBuilder meBuilder = MultipartEntityBuilder.create();
if (maps != null) {
for (String key : maps.keySet()) {
meBuilder.addPart(key, new StringBody(maps.get(key), ContentType.TEXT_PLAIN));
}
}
if (fileLists != null) {
for (File file : fileLists) {
FileBody fileBody = new FileBody(file);
meBuilder.addPart("files", fileBody);
}
}
HttpEntity reqEntity = meBuilder.build();
httpPost.setEntity(reqEntity);
return sendHttpPost(httpPost);
}
/**
* 发送 post请求
*
* @param httpUrl
* 地址
* @param params
* 参数(格式:key1=value1&key2=value2)
*
*/
public static String sendHttpPost(String httpUrl, String params) {
HttpPost httpPost = new HttpPost(httpUrl);// 创建httpPost
try {
// 设置参数
if (params != null && params.trim().length() > 0) {
StringEntity stringEntity = new StringEntity(params, "UTF-8");
stringEntity.setContentType(CONTENT_TYPE_FORM_URL);
httpPost.setEntity(stringEntity);
}
} catch (Exception e) {
e.printStackTrace();
}
return sendHttpPost(httpPost);
}
/**
* 发送 post请求
*
* @param maps
* 参数
*/
public static String sendHttpPost(String httpUrl, Map<String, String> maps) {
String parem = convertStringParamter(maps);
return sendHttpPost(httpUrl, parem);
}
/**
* 发送 post请求 发送json数据
*
* @param httpUrl
* 地址
* @param paramsJson
* 参数(格式 json)
*
*/
public static String sendHttpPostJson(String httpUrl, String paramsJson) {
HttpPost httpPost = new HttpPost(httpUrl);// 创建httpPost
try {
// 设置参数
if (paramsJson != null && paramsJson.trim().length() > 0) {
StringEntity stringEntity = new StringEntity(paramsJson, "UTF-8");
stringEntity.setContentType(CONTENT_TYPE_JSON_URL);
httpPost.setEntity(stringEntity);
}
} catch (Exception e) {
e.printStackTrace();
}
return sendHttpPost(httpPost);
}
/**
* 发送 post请求 发送xml数据
*
* @param httpUrl 地址
* @param paramsXml 参数(格式 Xml)
*
*/
public static String sendHttpPostXml(String httpUrl, String paramsXml) {
HttpPost httpPost = new HttpPost(httpUrl);// 创建httpPost
try {
// 设置参数
if (paramsXml != null && paramsXml.trim().length() > 0) {
StringEntity stringEntity = new StringEntity(paramsXml, "UTF-8");
stringEntity.setContentType(CONTENT_TYPE_TEXT_HTML);
httpPost.setEntity(stringEntity);
}
} catch (Exception e) {
e.printStackTrace();
}
return sendHttpPost(httpPost);
}
/**
* 将map集合的键值对转化成:key1=value1&key2=value2 的形式
*
* @param parameterMap
* 需要转化的键值对集合
* @return 字符串
*/
public static String convertStringParamter(Map parameterMap) {
StringBuffer parameterBuffer = new StringBuffer();
if (parameterMap != null) {
Iterator iterator = parameterMap.keySet().iterator();
String key = null;
String value = null;
while (iterator.hasNext()) {
key = (String) iterator.next();
if (parameterMap.get(key) != null) {
value = (String) parameterMap.get(key);
} else {
value = "";
}
parameterBuffer.append(key).append("=").append(value);
if (iterator.hasNext()) {
parameterBuffer.append("&");
}
}
}
return parameterBuffer.toString();
}
public static void main(String[] args) throws Exception {
System.out.println(sendHttpGet("http://www.baidu.com"));
}
}
Recommend
-
37
背景 最近遇到一个下载的需求,由于 url 参数太长(常用的下载方法 a 标签或者 location.href 的方法都是 get 请求,get 请求参数长度有限制),无法下载,考虑了好几种方案,最终还是觉得通过 ajax 的 POST 方法进行下载,比较...
-
34
谁说 HTTP GET 就不能通过 Body 来发送数据呢? 2017-08-19 — Yanbin 当我们被问及 HTTP 的 GET 与 POST 两种请求方式的区别的时候,很多答案是说 GET 的数据须通过 URL 以 Query Parameter 来传送,而 POST 可以通过请求体来发送数据,所以...
-
9
使用Spring WebClient发送HTTP请求Spring 5有一个响应式 Web 框架:Spring WebFlux。这旨在与现有的 Spring Web MVC API 共存,但增加对非阻塞设计的支持。使用 WebFlux,您可以构建异步 Web 应用程序,使用反应式流和函数式 API 来更好地支持并发和扩展。
-
7
dart:html包为dart提供了构建浏览器客户端的一些必须的组件,之前我们提到了HTML和DOM的操作,除了这些之外,我们在浏览器端另一个常用的操作就是使用XMLHttpRequest去做异步HTTP资源的请求,也就是AJAX请求。 dart同样提供了类似JS中XMLHttpRequest的封...
-
8
1.荒腔走板 前几天有个童鞋在群里面问:怎么使用HttpClient发送文件? 之前我写了一个ABP上传文件,主要体现的是服务端,上传文件的动作是由前端小姐姐完成的, 我还真没有用HttpClient编程方式发送过文件。 不过想来,Web协议都是一样...
-
7
通过向 DNS 服务发送 SRV 查询请求获取 kubernetes 集群内所有 Service 信息...
-
4
go代码示例:发送http网络请求 评分: 4.5 作者: Ryan Lu 类别: golang
-
5
在用户离开页面时可靠地发送 HTTP 请求 HTTP在某些情况下,当用户执行诸如导航到不同页面或提交表单之类的操作时,我需要发送带有一些数据的请求...
-
8
curl Curl 设置发送 HTTP 请求头 Header Curl 是用于在本地计算机与远程服务器之间传输数据的命令行工具 ...
-
10
Dart 通过 Dio 发送 HTTP 请求 dart pub add dio Flutterflutter pub add dio import 'package:dio/dio.dart'; final dio =...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK