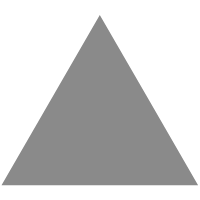
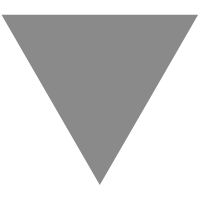
scalar type-casting
source link: https://externals.io/message/98749
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
scalar type-casting
Since PHP 7.0, I've started using scalar type-hints a lot, and it got me
thinking about scalar type-casting.
After poking through existing RFC's, it turns out, I was going to propose
precisely the thing that Anthony Ferrara proposed in 2012:
https://wiki.php.net/rfc/object_cast_to_types
In the light of scalar type-hints, I feel this RFC is now much more
relevant and useful than it was then.
One thing in this RFC jumps out at me though:
"when an internal function accepts a typed parameter by reference, if the
magic cast method is defined on the passed in object, an error is raised as
the cast cannot be performed because of the reference."
I'm afraid this is inconsistent with the behavior of built-in scalar
type-casts.
For example:
function add_one(int &$value) { $value += 1; }
$one = "1";
add_one($one);
var_dump($one); // int(2)
That is, an implicit type-cast made by passing e.g. a string to an int
reference argument has the side-effect of overwriting the input variable.
This behavior may be "okay" in the case of scalars, which, for the most
part, can just ping-pong between actual types - like, if someone were to
subsequently append to what they thought was a string in the above example,
the string-turned-integer would just convert itself back to a string.
The situation would be very different with an object being passed as
argument and cast to integer - if the object was simply replaced with an
integer as a side-effect, clearly this would have much more serious
ramifications than with scalars which can probably be cast back and forth
between various scalar types.
I'm guessing, at the time when scalar type-hints were introduced, you
likely weighed the pros and cons while designing this behavior and decided
it's "good enough", since it's damn near impossible to define another
rational behavior that is side-effect free and would also do something
meaningful with references (?)
It seems that references are once again the culprit that inspired "weird"
design-decisions such as side-effects.
I would call again for the deprecation/removal of references, but I know
that's a major language BC break and very unlikely to bear fruit, so I
won't suggest that.
Instead, I would like you to consider another, much smaller BC break, much
less likely to affect most code: rather than type-casting values when
passed by reference, instead type-check them, and error on mismatch.
That is, in the example above, add_one($one) would trigger an error,
because the variable you passed by reference isn't of the correct type.
I would need to refactor that code slightly, and introduce an intermediary
variable that is actually an integer, then call the function - or in other
words, I would need to write code that expresses what really happens, the
fact that the function operates on an integer variable in the calling scope:
$one = "1";
$one_int = (int) $one;
add_one($one_int);
This is much safer and much more transparent than the potentially very
surprising side-effect of having your local variable overwritten with a
different type.
The problem I'm describing is pretty serious for the one type-cast that
exists at present: __toString()
Example:
class Foo { public function __toString() { return "foo"; } }
function append_to(string &$str) { $str .= "_bar"; }
$foo = new Foo();
append_to($foo);
var_dump($foo); // string(7) "foo_bar"
In this example, the caller's instance of Foo gets wiped out and replaced
by a string - the "ping pong type-casting" that saved us in the previous
example won't save us this time.
While the side-effects for scalars being replaced by scalars may be "okay"
under most circumstances, I think this kind of side-effect is pretty
unnatural and surprising for any non-scalar type.
Most of the time, arguments are not by-reference, so I think changing this
this will likely have a pretty minimal impact on real world code - and the
work around (as in the previous example) is pretty easy to implement, and
could likely be fully automated by e.g. PHP Storm, CodeSniffer's cbf tool,
etc.
With this change, what Anthony proposed in 2012 becomes feasible, I think?
(And perhaps it comes feasible to (later) think about completing the
type-casting feature with support for casting between class/interface
types, but that's another subject...)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK