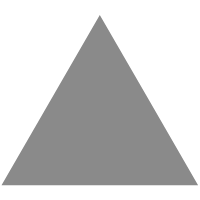
6
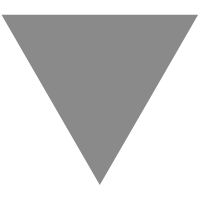
laravel 安装jwt
source link: https://blog.51cto.com/u_4483409/5715552
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
1.安装 jwt-auth
composer require tymon/jwt-auth:dev-develop

参考文档:Installation · tymondesigns/jwt-auth Wiki · GitHub
2.在 config/app.php 的 providers 配置项中注册服务提供者
'providers' => [
、、、
Tymon\JWTAuth\Providers\LaravelServiceProvider::class,
],
'aliases' => [
、、、
'JWTAuth' => Tymon\JWTAuth\Facades\JWTAuth::class,
'JWTFactory' => Tymon\JWTAuth\Facades\JWTFactory::class,
],
、、、
Tymon\JWTAuth\Providers\LaravelServiceProvider::class,
],
'aliases' => [
、、、
'JWTAuth' => Tymon\JWTAuth\Facades\JWTAuth::class,
'JWTFactory' => Tymon\JWTAuth\Facades\JWTFactory::class,
],
3.生成配置文件
php artisan vendor:publish --provider="Tymon\JWTAuth\Providers\LaravelServiceProvider"
4.生成密钥
php artisan jwt:secret
此命令会在你的 .env
文件中新增一行 JWT_SECRET=secret
此命令会在 config
目录下生成 jwt.php
4.创建模型(若已存在用户模型,则不生成,修改对应的继承关系即可)
php artisan make:model Model/User

<?php
namespace App\Model;
use Tymon\JWTAuth\Contracts\JWTSubject;
use Illuminate\Foundation\Auth\User as Authenticatable;
/**
* 说明:
* 1.账号名可不用name,但密码必须要用password字段
*/
class User extends Authenticatable implements JWTSubject
{
protected $fillable = ['name', 'password'];
protected $hidden = ['password', 'remember_token'];
/**
* 新增方法
*/
public function getJWTIdentifier()
{
return $this->getKey();
}
/**
* 新增方法
*/
public function getJWTCustomClaims()
{
return [];
}
}
namespace App\Model;
use Tymon\JWTAuth\Contracts\JWTSubject;
use Illuminate\Foundation\Auth\User as Authenticatable;
/**
* 说明:
* 1.账号名可不用name,但密码必须要用password字段
*/
class User extends Authenticatable implements JWTSubject
{
protected $fillable = ['name', 'password'];
protected $hidden = ['password', 'remember_token'];
/**
* 新增方法
*/
public function getJWTIdentifier()
{
return $this->getKey();
}
/**
* 新增方法
*/
public function getJWTCustomClaims()
{
return [];
}
}
5.修改配置文件 auth.php
'guards' => [
'api' => [
'driver' => 'jwt',
'provider' => 'users', // 对应下方的providers=>users数组
'hash' => false,
],
],
'providers' => [
'users' => [
'driver' => 'eloquent',
'model' => App\Models\User::class // 对应上一步的模型
],
'api' => [
'driver' => 'jwt',
'provider' => 'users', // 对应下方的providers=>users数组
'hash' => false,
],
],
'providers' => [
'users' => [
'driver' => 'eloquent',
'model' => App\Models\User::class // 对应上一步的模型
],
6.实现login 注册返回 token
php artisan make:controller Api/UserController // 创建控制器
<?php
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use App\Models\Member;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
use Tymon\JWTAuth\Facades\JWTAuth;
use Tymon\JWTAuth\Facades\JWTFactory;
class UserController extends Controller
{
public function __construct()
{
$this->middleware('auth:api', ['except' => ['register', 'login']]);
}
/**
* 用户注册
*/
public function register(Request $request)
{
// jwt token
$credentials = [
'name' => $request->name,
'password' => Hash::make($request->password)
];
$user = User::create($credentials);
if($user){
$token = JWTAuth::fromUser($user);
// JWTFactory::setTTL(80);// 设置TOKEN缓存时间
return $this->responseWithToken($token);
}
}
/**
* 用户login
*/
public function login(Request $request)
{
// todo 用户login逻辑
// jwt token
$credentials = $request->only('name', 'password');
// $credentials['status'] = 1; // 在login的可加
if (!$token = JWTAuth::attempt($credentials)) {
return response()->json(['result'=>'failed']);
}
// JWTFactory::setTTL(1);// 设置TOKEN缓存时间
return $this->responseWithToken($token);
}
/**
* 刷新token
*/
public function refresh()
{
return $this->responseWithToken(JWTAuth::refresh());
}
/**
* 退出
*/
public function logout(Request $request)
{
JWTAuth::invalidate(JWTAuth::getToken()); // 即把当前token加入黑名单
// auth()->logout();
}
/**
* 获取用户信息
*/
public function userInfo(Request $request)
{
$user = [];
try {
$token = JWTAuth::getToken();
if($token){
JWTAuth::setToken($token);
$user = JWTAuth::toUser();
}
} catch (\Exception $exception) {
dd($exception->getMessage());
}
return response()->json(['result' => $user]);
}
/**
* 响应
*/
private function responseWithToken(string $token)
{
$response = [
'access_token' => $token,
'token_type' => 'Bearer',
'expires_in' => JWTFactory::getTTL() * 60
];
return response()->json($response);
}
}
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use App\Models\Member;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
use Tymon\JWTAuth\Facades\JWTAuth;
use Tymon\JWTAuth\Facades\JWTFactory;
class UserController extends Controller
{
public function __construct()
{
$this->middleware('auth:api', ['except' => ['register', 'login']]);
}
/**
* 用户注册
*/
public function register(Request $request)
{
// jwt token
$credentials = [
'name' => $request->name,
'password' => Hash::make($request->password)
];
$user = User::create($credentials);
if($user){
$token = JWTAuth::fromUser($user);
// JWTFactory::setTTL(80);// 设置TOKEN缓存时间
return $this->responseWithToken($token);
}
}
/**
* 用户login
*/
public function login(Request $request)
{
// todo 用户login逻辑
// jwt token
$credentials = $request->only('name', 'password');
// $credentials['status'] = 1; // 在login的可加
if (!$token = JWTAuth::attempt($credentials)) {
return response()->json(['result'=>'failed']);
}
// JWTFactory::setTTL(1);// 设置TOKEN缓存时间
return $this->responseWithToken($token);
}
/**
* 刷新token
*/
public function refresh()
{
return $this->responseWithToken(JWTAuth::refresh());
}
/**
* 退出
*/
public function logout(Request $request)
{
JWTAuth::invalidate(JWTAuth::getToken()); // 即把当前token加入黑名单
// auth()->logout();
}
/**
* 获取用户信息
*/
public function userInfo(Request $request)
{
$user = [];
try {
$token = JWTAuth::getToken();
if($token){
JWTAuth::setToken($token);
$user = JWTAuth::toUser();
}
} catch (\Exception $exception) {
dd($exception->getMessage());
}
return response()->json(['result' => $user]);
}
/**
* 响应
*/
private function responseWithToken(string $token)
{
$response = [
'access_token' => $token,
'token_type' => 'Bearer',
'expires_in' => JWTFactory::getTTL() * 60
];
return response()->json($response);
}
}
7.创建中间件解决跨域问题(无需跨域可以省去此步奏)
php artisan make:middleware Cors

编辑 app/Http/Middleware/Cors.php
<?php
namespace App\Http\Middleware;
use Closure;
class Cors
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
//解决跨域问题
header('Access-Control-Allow-Origin: *');
$headers = [
'Access-Control-Allow-Methods'=> 'POST, GET, OPTIONS, PUT, DELETE',
'Access-Control-Allow-Headers'=> 'Content-Type, X-Auth-Token, Origin'
];
if($request->getMethod() == "OPTIONS") {
return Response::make('OK', 200, $headers);
}
$response = $next($request);
foreach($headers as $key => $value)
$response->header($key, $value);
return $response;
}
}
namespace App\Http\Middleware;
use Closure;
class Cors
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
//解决跨域问题
header('Access-Control-Allow-Origin: *');
$headers = [
'Access-Control-Allow-Methods'=> 'POST, GET, OPTIONS, PUT, DELETE',
'Access-Control-Allow-Headers'=> 'Content-Type, X-Auth-Token, Origin'
];
if($request->getMethod() == "OPTIONS") {
return Response::make('OK', 200, $headers);
}
$response = $next($request);
foreach($headers as $key => $value)
$response->header($key, $value);
return $response;
}
}
注册中间件 ----编辑 app/Http/Kernel.php
/**
* The application's route middleware.
*
* These middleware may be assigned to groups or used individually.
*
* @var array
*/
protected $routeMiddleware = [
...
'cors'=> \App\Http\Middleware\CORS::class,
];
* The application's route middleware.
*
* These middleware may be assigned to groups or used individually.
*
* @var array
*/
protected $routeMiddleware = [
...
'cors'=> \App\Http\Middleware\CORS::class,
];
8.创建中间件验证token
php artisan make:middleware User/authJWT
编辑 app/Http/Middleware/authJWT.php
<?php
namespace App\Http\Middleware\User;
use Closure;
use Tymon\JWTAuth\Facades\JWTAuth;
use Tymon\JWTAuth\Exceptions\JWTException;
use Tymon\JWTAuth\Exceptions\TokenExpiredException;
use Tymon\JWTAuth\Exceptions\TokenInvalidException;
class authJWT
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
try {
/**
*TODO 获取用户信息的方法可封装起来
*对应的放回参数可根据个人习惯进行自定义
*/
$token = JWTAuth::getToken();
if ($token) JWTAuth::setToken($token);
if (!$user = JWTAuth::toUser()){
return response()->json([
'errcode' => 400004,
'errmsg' => '无此用户'
], 404);
}
} catch (TokenExpiredException $e) {
return response()->json([
'errcode' => 400001,
'errmsg' => 'token 过期'
], $e->getStatusCode());
} catch (TokenInvalidException $e) {
return response()->json([
'errcode' => 400003,
'errmsg' => 'token 失效'
], $e->getStatusCode());
} catch (JWTException $e) {
return response()->json([
'errcode' => 400002,
'errmsg' => 'token 参数错误'
], $e->getStatusCode());
}
return $next($request);
}
}
namespace App\Http\Middleware\User;
use Closure;
use Tymon\JWTAuth\Facades\JWTAuth;
use Tymon\JWTAuth\Exceptions\JWTException;
use Tymon\JWTAuth\Exceptions\TokenExpiredException;
use Tymon\JWTAuth\Exceptions\TokenInvalidException;
class authJWT
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
try {
/**
*TODO 获取用户信息的方法可封装起来
*对应的放回参数可根据个人习惯进行自定义
*/
$token = JWTAuth::getToken();
if ($token) JWTAuth::setToken($token);
if (!$user = JWTAuth::toUser()){
return response()->json([
'errcode' => 400004,
'errmsg' => '无此用户'
], 404);
}
} catch (TokenExpiredException $e) {
return response()->json([
'errcode' => 400001,
'errmsg' => 'token 过期'
], $e->getStatusCode());
} catch (TokenInvalidException $e) {
return response()->json([
'errcode' => 400003,
'errmsg' => 'token 失效'
], $e->getStatusCode());
} catch (JWTException $e) {
return response()->json([
'errcode' => 400002,
'errmsg' => 'token 参数错误'
], $e->getStatusCode());
}
return $next($request);
}
}
注册该中间件----编辑 app/Http/Kernel.php
/**
* The application's route middleware.
*
* These middleware may be assigned to groups or used individually.
*
* @var array
*/
protected $routeMiddleware = [
...
'authJWT'=> \App\Http\Middleware\User\authJWT::class, // 不能使用'jwt.auth',因为安装包已经使用了,若验证失败默认是返回401页面而不是json参数
];
* The application's route middleware.
*
* These middleware may be assigned to groups or used individually.
*
* @var array
*/
protected $routeMiddleware = [
...
'authJWT'=> \App\Http\Middleware\User\authJWT::class, // 不能使用'jwt.auth',因为安装包已经使用了,若验证失败默认是返回401页面而不是json参数
];
routes\api.php
<?php
use Illuminate\Http\Request;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| 若需要添加跨域的中间件可设成['middleware' => ['api','cors'],'prefix' => 'admin-user']
| 我的这些中间件在 app/Providers/RouteServiceProvider.php 中已设置,
| 所以在当前文件不需要添加
|
*/
Route::group(['prefix' => 'admin'], function () {
// 当前为不要验证TOKEN的路由
Route::any('login', 'Api\UserController@login');
Route::any('register', 'Api\UserController@register');
// 需要验证token的路由可在下方添加
Route::group(['middleware' => 'authJWT'], function () {
Route::any('user-info', 'Api\UserController@userInfo');
});
});
use Illuminate\Http\Request;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| 若需要添加跨域的中间件可设成['middleware' => ['api','cors'],'prefix' => 'admin-user']
| 我的这些中间件在 app/Providers/RouteServiceProvider.php 中已设置,
| 所以在当前文件不需要添加
|
*/
Route::group(['prefix' => 'admin'], function () {
// 当前为不要验证TOKEN的路由
Route::any('login', 'Api\UserController@login');
Route::any('register', 'Api\UserController@register');
// 需要验证token的路由可在下方添加
Route::group(['middleware' => 'authJWT'], function () {
Route::any('user-info', 'Api\UserController@userInfo');
});
});

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK