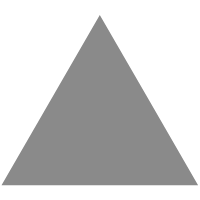
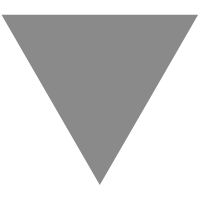
七个我在工作中经常使用的 JavaScript 技巧
source link: https://www.51cto.com/article/719561.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
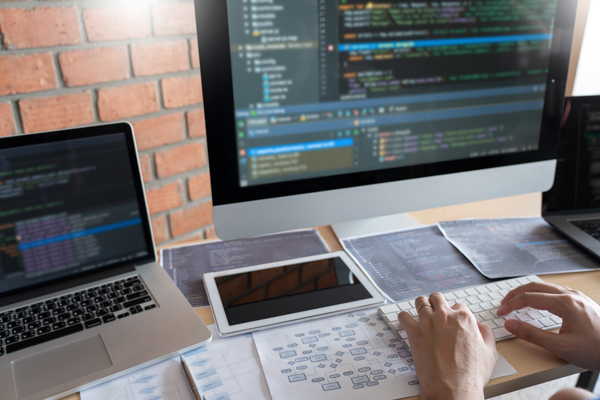
ES6 给我们编程带来了很多便利,以前用大量代码实现的功能现在变得非常简洁。
本文总结了我在工作中经常使用的 7 个 JavaScript 技巧,希望对你也有帮助。
1. 找出数组中的最大值或最小值
有时,我们需要找到数组中的最大值,你通常是怎么做的?
解决方案 1
我们可以先对数组进行排序,然后,数组的最后一项就是最大值。
const array = [ 1, 10, -19, 2, 7, 100 ]
array.sort((a, b) => a - b)
console.log('max value', array[ array.length - 1 ])
console.log('min value', array[ 0 ])
解决方案 2
还有其他解决方案吗?是的,我们可以使用“Math.max”轻松处理它。
const array = [ 1, 10, -19, 2, 7, 100 ]
console.log('max value', Math.max(...array))
console.log('min value', Math.min(...array))
2.计算数组的总和
如果有一个数字数组,得到它们总和的最快方法是什么?
const array = [ 1, 10, -19, 2, 7, 100 ]
const sum = array.reduce((sum, num) => sum + num)
3. 从数组中获取随机值
给你一个数组,现在你想从中获取一个随机值,你怎么做呢?
const array = [ 'fatfish', 'fish', 24, 'hello', 'world' ]
const getRandomValue = (array) => {
return array[ Math.floor(Math.random() * array.length) ]
}
console.log(getRandomValue())
console.log(getRandomValue())
console.log(getRandomValue())
4.随机打乱数组的值
我们在抽奖的时候,需要打乱抽奖顺序。
const prizes = [ '📱', '🍫', '🍵', '🍔' ]
prizes.sort(() => 0.5 - Math.random())
console.log(prizes)
prizes.sort(() => 0.5 - Math.random())
console.log(prizes)
5. 展平多层阵列
现在我们有了一个多维嵌套数组,如何将其铺成一维数组?
解决方案 1
const array = [ 1, [ 2, [ 3, [ 4, [ 5 ] ] ] ] ]
const flattenArray = (array) => {
return array.reduce((res, it) => {
return res.concat(Array.isArray(it) ? flattenArray(it) : it)
}, [])
}
console.log(flattenArray(array))
解决方案 2
事实上,我们有一个更简单的方法来解决它。关于flat,我们来看看MDN的解释:
flat() 方法创建一个新数组,其中所有子数组元素递归连接到指定深度。
const array = [ 1, [ 2, [ 3, [ 4, [ 5 ] ] ] ] ]
console.log(array.flat(Infinity))
6.检查数组是否包含值
过去,我们总是使用“indexOf”方法来检查数组是否包含值。如果“indexOf”返回的值大于-1,则表示有一个。
const array = [ 'fatfish', 'hello', 'world', 24 ]
console.log(array.indexOf('fatfish'))
console.log(array.indexOf('medium'))
但是,现在数据比较复杂,我们将无法通过 indexOf 方法直接确认数组中是否存在“fatfish”。幸运的是,ES6 中提供了 findIndex 方法。
const array = [
{
name: 'fatfish'
},
{
name: 'hello'
},
{
name: 'world'
},
]
const index = array.findIndex((it) => it.name === 'fatfish') // 0
7.使用“includes”方法进行判断
你一定见过这样的判断方法,虽然,可以达到条件判断的目的,但是,看起来很繁琐。
const value = 'fatfish'
if (value === 'fatfish' || value === 'medium' || value === 'fe') {
console.log('hello world')
}
我们可以使用includes方法让代码更简单甚至更可扩展。
const conditions = [ 'fatfish', 'medium', 'fe' ]
const value = 'fatfish'
if (conditions.includes(value)) {
console.log('hello world')
}
以上就是我今天跟你分享的7个我在工作中经常使用的ES6技巧,希望这些技巧也能够帮助到你
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK