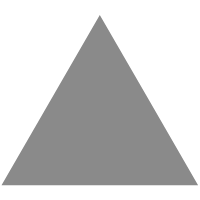
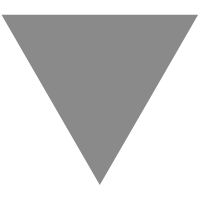
Run Selenium Headless – Chrome | Firefox (Python)
source link: https://www.shellhacks.com/run-selenium-headless-chrome-firefox-python/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Run Selenium Headless – Chrome | Firefox (Python)
Selenium is an open source tool for web browsers automation that is widely used in tandem with Python to test web applications.
As these tests are often automated and run on dedicated nodes, there is a sense to run Selenium in a headless mode, i.e. without opening a browser.
This note shows how to run headless Selenium in Python on the example of Chrome and Firefox browsers controlled by a ChromeDriver and GeckoDriver correspondingly.
Cool Tip: How to automate login to a website using Selenium in Python! Read More →
Run Headless Selenium in Python
Create a project’s working directory:
$ mkdir -p ~/projects/headless-selenium
Inside the project’s working directory create and activate a virtual environment:
$ cd ~/projects/headless-selenium $ python3 -m venv venv $ . venv/bin/activate
Install Selenium:
$ pip install selenium webdriver_manager $ pip show selenium | grep -i version Version: 4.4.3 $ pip show webdriver_manager | grep -i version Version: 3.8.3
Headless Chrome (ChromeDriver)
Download and unzip the latest stable version of a ChromeDriver (the executable that Selenium uses to interact with Chrome):
$ sudo unzip ~/Downloads/chromedriver_linux64.zip -d /usr/local/bin $ chromedriver --version ChromeDriver 104.0.5112.79
Create a run-headless-chrome.py
with the contents as follows:
# run-headless-chrome.py # by www.ShellHacks.com from selenium import webdriver from selenium.webdriver.chrome.service import Service from selenium.webdriver.chrome.options import Options chrome_options = Options() chrome_options.add_argument("--headless") driver = webdriver.Chrome(service=Service('/usr/local/bin/chromedriver'), options=chrome_options) driver.get('https://example.tld') print(driver.page_source.encode("utf-8")) driver.quit()
Execute the Python script:
$ python run-headless-chrome.py
This script should run Selenium headless (without opening Chrome) and print the source code of the https://example.tld webpage to the terminal.
Cool Tip: How to add random delays in Python to not get banned! Read More →
Headless Firefox (GeckoDriver)
Download and untar the latest stable version of a GeckoDriver (the executable that Selenium uses to interact with Firefox):
$ sudo tar -xvf ~/Downloads/geckodriver-*.tar.gz -C /usr/local/bin/ $ geckodriver --version geckodriver 0.31.0
Create a run-headless-firefox.py
with the contents as follows:
# run-headless-firefox.py # by www.ShellHacks.com from selenium import webdriver from selenium.webdriver.firefox.service import Service from selenium.webdriver.firefox.options import Options firefox_options = Options() firefox_options.add_argument("--headless") driver = webdriver.Chrome(service=Service('/usr/local/bin/geckodriver'), options=firefox_options) driver.get('https://base64-encode.online') print(driver.page_source.encode("utf-8")) driver.quit()
Execute the Python script:
$ python run-headless-firefox.py
This script should run Selenium headless (without opening Firefox) and print the source code of the https://example.tld webpage to the terminal.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK