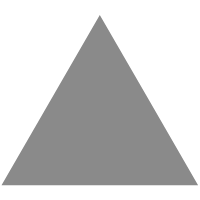
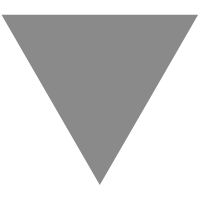
Paging and virtual lists
source link: https://devm.io/javascript/javascript-paging-lists
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
26. Aug 2022
In the previous issue of this column, we dealt with the topics of sorting and filtering lists. We asked ourselves if we prefer performing these operations on the client or server side. Our experiments involved displaying a list of 10,000 records—filtering, and sorting them according to a certain criterion. In this instance, the client side variant cut a better overall figure. But this doesn’t necessarily mean that these operations are generally better off on the client. This time, we'll revisit this topic and look at other options that can improve list display performance.
Pagination
Let's be honest: Who’d voluntarily work with a list of 10,000 entries? The browser scrollbar gets so small you can hardly see it and you live in constant fear that the browser will stop working because of memory consumption and the scroll event’s constant rendering. What’s more, 10,000 data sets move in a dimension where it’s already getting really exhausting for normal people like me. For this reason, you should ask yourself if you really want to throw this amount of data at your users. The following argument is often used: If I display everything, I can easily search for it with CTRL + F. Yes, that’s true, of course. However, this argument is very easy to refute. Your browser lets you respond to all sorts of events. This includes keyboard events and the key combination CTRL + F (or CMD + F on a Mac). The following code snippet makes sure that the search shortcut—CTRL + F—is intercepted and the standard routine of opening the search window is prevented. Instead, you focus on the element with the ID search, which you can use to search in your application:
document.addEventListener('keydown', (event) => {
if (event.code === 'KeyF' && (event.metaKey || event.ctrlKey)) {
event.preventDefault();
document.querySelector('#search').focus();
}
});
But please be careful with these actions. Not all users are happy when developers patronize them. Furthermore, there’s the risk of introducing an error in your routine and then searching won’t be possible at all. So, if you implement these features, you have to be 100% sure your users want the feature and that it works flawlessly. By the way, it’s similar with the right-click. You should think carefully about whether to intervene.
But now back to our actual question: Pagination in applications. Here you can act on the client or server side. On the server side, the advantage is that you can outsource the work to the database and feedback is relatively fast. On the client side, the communication overhead is eliminated.
Test 1: Server side pagination
With server-side pagination, the server delivers only a certain portion of the result set. In most cases, the users can configure the number of results per display page. In our example, the application is a simple address list with 10,000 records altogether. The backend is a Node.js application based on Express.js with Sequelize as database abstraction and a MySQL database for data storage. The frontend is a React application that sends a GET request to the backend, passing the page to be displayed as a query parameter. The server-side implementation looks like this:
app.get('/addresses', async (request, response) => {
const options = { order: [['id', 'ASC']] };
if (request.params.page) {
options.limit = 30;
options.offset = (request.body.page - 1) * 30;
}
const addresses = await Address.findAll(options);
response.json(addresses);
});
With the limit and offset options, you tell the database exactly how many and which records you’re interested in. If you make a query directly...
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK