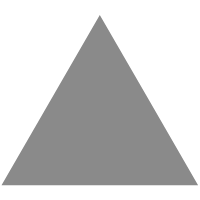
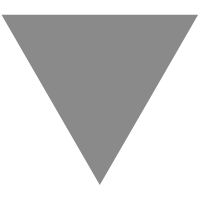
React报错之map() is not a function
source link: https://www.fly63.com/article/detial/11977
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
扫一扫分享
当我们对一个不是数组的值调用 map() 方法时,就会产生 "TypeError: map is not a function" 错误。为了解决该错误,请将你调用 map() 方法的值记录在 console.log 上,并确保只对有效的数组调用 map 。

这里有个示例来展示错误是如何发生的。
const App = () => {
const obj = {};
// :no_entry:️ Uncaught TypeError: map is not a function
return (
<div>
{obj.map(element => {
return <h2>{element}</h2>;
})}
</div>
);
};
export default App;
我们在一个对象上调用 Array.map() 方法,得到了错误反馈。
为了解决该错误,请 console.log 你调用 map 方法的值,确保它是一个有效的数组。
export default function App() {
const arr = ['one', 'two', 'three'];
return (
<div>
{arr.map((element, index) => {
return (
<div key={index}>
<h2>{element}</h2>
</div>
);
})}
</div>
);
}
Array.isArray
你可以通过使用 Array.isArray 方法,来有条件地检查值是否为数组。
const App = () => {
const obj = {};
return (
<div>
{Array.isArray(obj)
? obj.map(element => {
return <h2>{element}</h2>;
})
: null}
</div>
);
};
export default App;
如果值为数组,则返回对其调用 map 方法的结果,否则返回 null 。这种方式不会得到错误,即使值不是一个数组。
如果值是从远程服务中获取,请确保它是你期望的类型,将其记录到控制台,并确保你在调用 map 方法之前将其解析为一个原生JavaScript数组。
Array.from
如果有一个类数组对象,在调用 map 方法之前你尝试转换为数组,可以使用 Array.from() 方法。
const App = () => {
const set = new Set(['one', 'two', 'three']);
return (
<div>
{Array.from(set).map(element => {
return (
<div key={element}>
<h2>{element}</h2>
</div>
);
})}
</div>
);
};
export default App;
在调用 map 方法之前,我们将值转换为数组。这也适用于类数组的对象,比如调用 getElementsByClassName 方法返回的 NodeList 。
Object.keys
如果你尝试迭代遍历对象,使用 Object.keys() 方法获取对象的键组成的数组,在该数组上可以调用 map() 方法。
export default function App() {
const employee = {
id: 1,
name: 'Alice',
salary: 100,
};
return (
<div>
{/* :point_down:️ iterate object KEYS */}
{Object.keys(employee).map((key) => {
return (
<div key={key}>
<h2>
{key}: {employee[key]}
</h2>
<hr />
</div>
);
})}
<br />
<br />
<br />
{/* :point_down:️ iterate object VALUES */}
{Object.values(employee).map((value, index) => {
return (
<div key={index}>
<h2>{value}</h2>
<hr />
</div>
);
})}
</div>
);
}
我们使用 Object.keys 方法得到对象的键组成的数组。
const employee = {
id: 1,
name: 'Alice',
salary: 100,
};
// :point_down:️ ['id', 'name', 'salary']
console.log(Object.keys(employee));
// :point_down:️ [1, 'Alice', 100]
console.log(Object.values(employee));
我们只能在数组上调用 map() 方法,所以我们需要获得一个对象的键或者对象的值的数组。
原文链接:https://bobbyhadz.com/blog/react-map-is-not-a-function
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK