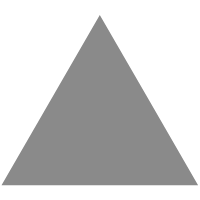
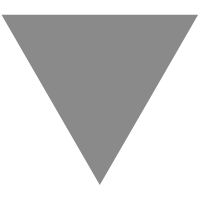
Single-threading is more memory-efficient
source link: https://coder-mike.com/blog/2022/05/27/single-threading-is-more-memory-efficient/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
TL;DR: Single-threading with super-loops or job queues may make more efficient use of a microcontroller’s memory over time, and Microvium’s closures make single-threading easier with callback-style async code.
Multi-threading
In my last post, I proposed the idea that we should think of the memory on a microcontroller not just as a space but as a space-time, with each memory allocation occupying a certain space for some duration of time:
I suggested that we should then measure the cost of an allocation in byte-seconds (the area of the above rectangles as bytes × seconds
), so long as we assumed that allocations were each small and occurred randomly over time. Randomness like this is a natural byproduct of a multi-threaded environment, where at any moment you may coincidentally have multiple tasks doing work simultaneously and each taking up memory. In this kind of situation, tasks must be careful to use as little memory as possible because at any moment some other tasks may start up and want to share the memory space for their own work.
This leaves a random chance that at any moment you could run out of memory if too many things happen at once. You can guard against this by just leaving large margins — lots of free memory — but this is not a very efficient use of the space. There is a better way: single threading.
Single threading
Firmware is sometimes structured in a so-called super-loop design, where the main
function has a single while(1)
loop that services all the tasks in turn (e.g. calling a function corresponding to each task in the firmware). This structure can have a significant advantage for memory efficiency. In this way of doing things, each task essentially has access to all the free memory while it has its turn, as long as it cleans up before the next task, as depicted in the following diagram. (And there may still be some statically-allocated memory and “long-lived” memory that is dynamic but used beyond the “turn” of a task).
Overall, this is a much more organized use of memory and potentially more space-efficient.
In the multi-threaded model, if two memory-heavy tasks require memory around the same time, neither has to wait for the other to be finished — or to put it another way, malloc
looks for available space but not available time for that space. On the other hand, in a super-loop model, those same tasks will each get a turn at different times. Each will have much more memory available to them during their turn while having much less impact on other tasks the rest of the time.
An animated diagram you may have seen before on my blog demonstrates the general philosophy here. A task remains idle until its turn, at which point it takes center stage and can use all the resources it likes, as long as it packs up and cleans up before the next task.
So, what counts as expensive in this new memory model?
It’s quite clear from the earlier diagram:
- Memory that is only used for one turn is clearly very cheap. Tasks won’t be interrupted during their turn, so they have full access to all the free memory without impacting the rest of the system.
- Statically-allocated memory is clearly the most expensive: it takes away from the available memory for all other tasks across all turns.
- Long-lived dynamic allocations — or just allocations that live beyond a single turn — are back to the stochastic model we had with multi-threading. Their cost is the amount of space × the number of turns they occupy the space for. Because these are a bit unpredictable, they also have a cost in that they add to the overall risk of running out of memory, so these kinds of allocations should be kept as small and short as possible.
Microvium is designed this way
Microvium is built from the ground up on this philosophy — keeping the idle memory usage as small as possible so that other operations get a turn to use that memory afterward, but not worrying as much about short spikes in memory that last only a single turn.
- The idle memory of a Microvium virtual machine is as low as 34 bytes1.
- Microvium uses a compacting garbage collector — one that consolidates and defragments all the living allocations into a single contiguous block — and releases any unused space back to the host firmware. The GC itself uses quite a bit of memory2 but it does so only for a very short time and only synchronously.
- The virtual call-stack and registers are deallocated when control returns from the VM back to the host firmware.
- Arrays grow their capacity geometrically (they double in size each time) but a GC cycle truncates unused space in arrays when it compacts.
See here for some more details.
Better than super-loop: a Job Queue
The trouble with a super-loop architecture is that it services every single task in each cycle. It’s inefficient and doesn’t scale well as the number of tasks grows3. There’s a better approach — one that JavaScript programmers will be well familiar with: the job queue.
A job queue architecture in firmware is still pretty simple. Your main loop is just something like this:
while (1) { if (thereIsAJobInTheQueue) doNextJob(); else goToSleep(); }
When I write bare-metal firmware, often the first thing I do is to bring in a simple job queue like this. If you’re using an RTOS, you might implement it using RTOS queues, but I’ve personally found that the job-queue style of architecture often obviates the need for an RTOS at all.
As JavaScript programmers may also be familiar with, working in a cooperative single-threaded environment has other benefits. You don’t need to think about locking, mutexes, race conditions, and deadlocks. There is less unpredictable behavior and fewer heisenbugs. In a microcontroller environment especially, a single-threaded design also means you also save on the cost of having multiple dedicated call stacks being permanently allocated for different RTOS threads.
Advice for using job queues
JavaScript programmers have been working with a single-threaded job-queue-based environment for decades and are well familiar with the need to keep the jobs short. When running JS in a browser, long jobs means that the page becomes unresponsive, and the same is true in firmware: long jobs make the firmware unresponsive — unable to respond to I/O or service accumulated buffers, etc. In a firmware scenario, you may want to keep all jobs below 1ms or 10ms, depending on what kind of responsiveness you need4.
As a rule of thumb, to keep jobs short, they should almost never block or wait for I/O. For example, if a task needs to power-on an external modem chip, it should not block while the modem to boots up. It should probably schedule another job to handle the powered-on event later, allowing other jobs to run in the meantime.
But in a single-threaded environment, how do we implement long-running tasks without blocking the main thread? Do you need to create complicated state machines? JavaScript programmers will again recognize a solution…
Callback-based async
JavaScript programmers will be quite familiar the pattern of using continuation-passing-style (CPS) to implement long-running operations in a non-blocking way. The essence of CPS is that a long-running operation should accept a callback
argument to be called when the operation completes.
The recent addition of closures (nested functions) as a feature in Microvium makes this so much easier. Here is a toy example one might use for sending data to a server in a multi-step process that continues across 3 separate turns of the job queue:
function sendToServer(url, data) { modem.powerOn(powerOnCallback); function powerOnCallback() { modem.connectTo(url, connectedCallback); } function connectedCallback() { modem.send(data); } }
Here, the data
parameter is in scope for the inner connectedCallback
function (closure) to access, and the garbage collector will automatically free both the closure and the data when they aren’t needed anymore. A closure like this is much more memory-efficient than having to allocate a whole RTOS thread, and much less complicated than manually fiddling with state machines and memory ownership yourself.
Microvium also supports arrow functions, so you could write this same example more succinctly like this:
function sendToServer(url, data) { modem.powerOn( () => modem.connectTo(url, () => modem.send(data))); }
Each of these 3 stages — powerOn
, connectTo
and send
— happen in a separate job in the queue. Between each job, the VM is idle — it does not consume any stack space5 and the heap is in a compacted state6.
If you’re interested in more detail about the mechanics of how modem.powerOn
etc. might be implemented in a non-blocking way, take a look at this gist where I go through this example in more detail.
Conclusion
So, we’ve seen that multi-threading can be a little hazardous when it comes to dynamic memory management because memory usage is unpredictable, and this also leads to inefficiencies because you need to leave a wider margin of error to avoid randomly running out of memory.
We’ve also seen how single-threading can help to alleviate this problem by allowing each operation to consume resources while it has control, as long it cleans up before the next operation. The super-loop architecture is a simple way to achieve this but an event-driven job-queue architecture is more modular and efficient.
And lastly, we saw that the Microvium JavaScript engine for embedded devices is well suited to this kind of design, because its idle memory usage is particularly small and because it facilitates callback-style asynchronous programming. Writing code this way avoids the hassle and complexity of writing state machines in C, of manually keeping track of memory ownership across those states, and the pitfalls and overheads of multithreading.
Or 22 bytes on a 16-bit platform
In the worst case, it doubles the size of heap while it’s collecting
A super-loop also makes it more challenging to know when to put the device to sleep since the main loop doesn’t necessarily know when there aren’t any tasks that need servicing right now, without some extra work.
There will still be some tasks that need to be real-time and can’t afford to wait even a few ms in a job queue to be serviced. I’ve personally found that interrupts are sufficient for handling this kind of real-time behavior, but your needs may vary. Mixing a job queue with some real-time RTOS threads may be a way to get the best of both worlds — if you need it.
Closures are stored on the virtual heap.
It’s in a compacted state if you run a GC collection cycle after each event, which you would do if you cared a lot about idle memory usage.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK