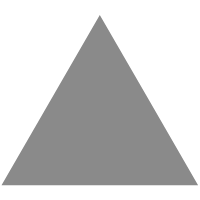
5
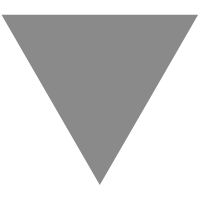
golang 日期、时间操作
source link: https://studygolang.com/articles/35671
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
golang 日期、时间操作
shensi · 1天之前 · 268 次点击 · 预计阅读时间 3 分钟 · 大约8小时之前 开始浏览一、整型、时间戳、日期时间格式类型转换
package main
import "fmt"
import "time"
func main() {
timeLayout := "2006-01-02 15:04:05" //转化所需模板
// 获取当前日期时间
now := time.Now()
fmt.Println(now) //2022-05-27 14:44:01.435578634 +0800 CST
fmt.Println(now.UnixMilli()) //获取当前时间毫秒 1653641009484
fmt.Println(now.UnixMicro()) //获取当前时间微秒 1653641009484528
fmt.Println(now.UnixNano()) //获取当前时间时纳秒 1632366278605122000
fmt.Println(now.Hour()) //获取当前小时 16
fmt.Println(now.Day()) //获取当前天 27
fmt.Println(now.Weekday()) //获取当前周 Friday
fmt.Println(now.ISOWeek()) //获取当前周2022 21
//格式化当前时间
fmt.Println(now.Format(timeLayout)) //2022-05-27 16:47:51
// 当前日期转时间戳
dn := now.Unix()
fmt.Println(dn) // 1653634563
// 时间戳转日期时间
ts := time.Unix(dn, 0)
fmt.Println(ts) // 2022-05-27 14:59:17 +0800 CST
// 指定时间戳转指定日期时间格式
var t1 int
t1 = 1653580799
t := time.Unix(int64(t1), 0).Format(timeLayout)
fmt.Println(t) // 2022-05-26 05:05:00
// 指定日期格式转时间戳
var cDate = "2022-06-06 09:10:00"
loc, _ := time.LoadLocation("Local") //获取时区
tmp, _ := time.ParseInLocation(timeLayout, cDate, loc)
timestamp := tmp.Unix() //转化为时间戳 类型是int64
fmt.Println(timestamp)
tm2, _ := time.Parse(timeLayout, cDate)
fmt.Println(tm2.Unix()) //1654506600 多8小时 默认时区非东八区
loc2, _ := time.LoadLocation("Asia/Shanghai")
fmt.Println(now.In(loc2).Format(timeLayout)) // 设置时区
fmt.Println(now.Format(timeLayout)) // 默认
// "2022-05-24T10:36:55Z" 转为当前时区日期时间
location, _ := time.LoadLocation("Asia/Shanghai")
t, _ := time.Parse(time.RFC3339, "2022-05-24T10:36:55Z")
fmt.Println(t.In(location)) // 2022-05-24 18:36:55 +0800 CST
fmt.Println("===")
}
二、日期、时间加减
// 通过时间戳加减时间
timeLayout := "2006-01-02 15:04:05" //转化所需模板
now := time.Now().Unix() // 当前时间戳
fmt.Println(time.Unix(now, 0).Format(timeLayout))
now += 86400 //增加一天
fmt.Println(time.Unix(now, 0).Format(timeLayout))
// 通过日期时间减1小时
now1 := time.Now()
fmt.Println(now1) // 2022-05-27 17:08:02.215482 +0800 CST m=+0.000265867
tp, _ := time.ParseDuration("-3600s")
// -1h0m0s -1h0m0s -3600 -3600000000000
fmt.Println(tp, tp.Truncate(1000), tp.Seconds(), tp.Nanoseconds())
m1 := now1.Add(tp)
fmt.Println(m1) // 2022-05-27 16:08:02.215482 +0800 CST m=-3599.999734133
tp, _ = time.ParseDuration("-1h")
m2 := now1.Add(tp)
fmt.Println(m2) // // 2022-05-27 16:08:02.215482 +0800 CST m=-3599.999734133
fmt.Println("===")
三、计算日期间隔
timeLayout := "2006-01-02 15:04:05" //转化所需模板
// Sub 计算两个时间差
now2 := time.Now()
tp, _ := time.ParseDuration("-1h")
m2 := now2.Add(tp)
fmt.Println(m2)
subM := now2.Sub(m2)
fmt.Println(subM.Minutes(), "分钟") //60 分钟
sumH := now2.Sub(m2)
fmt.Println(sumH.Hours(), "小时") //1 小时
sumD := now2.Sub(m2)
fmt.Printf("%v 天\n", fmt.Sprintf("%.2f", sumD.Hours()/24)) //0.04 天
// 等价于 Now().Sub(t), 可用来计算一段业务的消耗时间
fmt.Println(time.Since(m2)) // 1h0m0.000397422s
time3 := "2022-05-27 16:22:26"
time4 := "2022-05-27 16:22:26"
//先把时间字符串格式化成相同的时间类型
t3, _ := time.Parse(timeLayout, time3)
t4, _ := time.Parse(timeLayout, time4)
fmt.Println(t3.Equal(t4)) // true
fmt.Println("===")
有疑问加站长微信联系(非本文作者))

入群交流(和以上内容无关):加入Go大咖交流群,或添加微信:liuxiaoyan-s 备注:入群;或加QQ群:701969077
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK