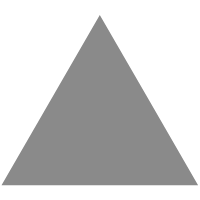
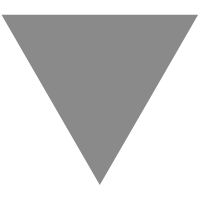
Override vs New Polymorphism In C# .NET
source link: https://dotnetcoretutorials.com/2022/05/13/override-vs-new-polymorphism-in-c-net/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Override vs New Polymorphism In C# .NET
I was recently asked by another developer on the difference between making a method virtual/override, and simply hiding the method using the new
keyword in C#.
I gave him what I thought to be the best answer (For example, you can change the return type when using the “new” keyword), and yet while showing him examples I managed to bamboozle myself into learning something new after all these years.
Take the following code for instance, what will it print?
Parent childOverride = new ChildOverride(); childOverride.WhoAmI(); Parent childNew = new ChildNew(); childNew.WhoAmI(); class Parent { public virtual void WhoAmI() { Console.WriteLine("Parent"); } } class ChildOverride : Parent { public override void WhoAmI() { Console.WriteLine("ChildOverride"); } } class ChildNew : Parent { public new void WhoAmI() { Console.WriteLine("ChildNew"); } }
At first glance, I assumed it would print the same thing either way. After all, I’m basically newing up the two different types, and in *both* cases I am casting it to the parent.
When casting like this, I like to tell junior developers that an object “Always remembers who it is”. That is, my ChildOverride can be cast to a Parent, or even an object, and it still remembers that it’s a ChildOverride.
So what does the above code actually print out?
ChildOverride Parent
So our Override method remembered who it was, and therefore it’s “WhoAmI” method. But our ChildNew did not… Kinda.
Why you might ask? Well it actually is quite simple if you think about it.
When you use the override keyword, it’s overriding the base class and there is a sort of “linkage” between the two methods. That is, it’s known that the child class is an override of the base.
When you use the new keyword, you are saying that the two methods are in no way related. And that your new method *only* exists on the child class, not on the parent. There is no “linkage” between the two.
This is why when you cast to the parent class, the overridden method is known, and the “new” method is not.
With all that being said, in many many years of programming in C# I have seldomly used the new keyword to override methods like this. Not only is there very little reason to do so, but it breaks a core SOLID principle in the Liskov Principle : https://dotnetcoretutorials.com/2019/10/20/solid-in-c-liskov-principle/
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Comment
Name *
Email *
Website
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK