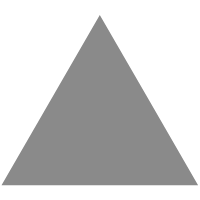
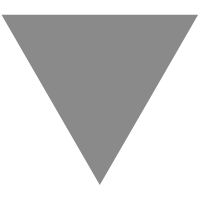
[Golang] Variadic Function Example - addEventListener
source link: http://siongui.github.io/2017/03/12/go-variadic-function-example-addEventListener/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
[Golang] Variadic Function Example - addEventListener
March 12, 2017
GopherJS [2] is a compiler from Go to JavaScript, which helps you write front-end Go code running in browsers.
To register event listeners, we can use the following code to call addEventListener method:
foo := js.Global.Get("document").Call("getElementById", "foo") // register onclick event foo.Call("addEventListener", "click", func(event *js.Object) { // do something }, false) // or foo.Call("addEventListener", "click", func(event *js.Object) { // do something })
You can pass two or three arguments to addEventListener method. But the above code is really ugly. We want to wrtie the code in idiomatic Go way, so I implement the addEventListener as a Go variadic function, which accepts two or more arguments.
The implementation of addEventListener in Go via GopherJS is as follows:
func (o *Object) AddEventListener(t string, listener func(Event), args ...interface{}) { if len(args) == 1 { o.Call("addEventListener", t, listener, args[0]) } else { o.Call("addEventListener", t, listener) } }
where the type Object and Event is as follows:
type Object struct { *js.Object } type Event struct { *js.Object }
JavaScript object is *js.Object in GopherJS, so the type Object and Event are in fact the wrapper for *js.Object.
Now we can register the event listeners in the following idiomatic Go way:
foo.AddEventListener("click", func(e Event) { // do something }) // or foo.AddEventListener("click", func(e Event) { // do something }, false)
You can pass more than three arguments, but the additional arguments after third argument will not be used in my implementation.
Tested on: Ubuntu Linux 16.10, Go 1.8
References:
[3][Golang] GopherJS Synonyms with JavaScript
[5]variadic function looping through channels : golang
[6]The Go variadic function, comparing errors in Golang, solving triangles & more : golang
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK