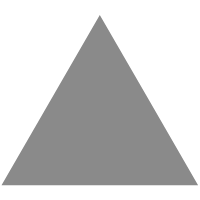
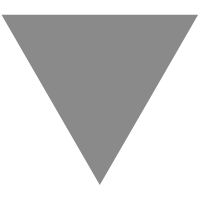
[Golang] Embed File in String Literal
source link: http://siongui.github.io/2017/04/26/go-embed-file-in-string-literal/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
[Golang] Embed File in String Literal
April 26, 2017
Embed any type of file, no matter it is data/assets/resources/binary/etc., in string literal in Go code.
When I tried googling to find the way to embed files directly in Go code, I found that if we combine the string literal with encoding/hex package in Go standard library, we can embed any type of file in Go code.
The first step is to convert the byte sequences of a file to hex value:
import ( "encoding/hex" "io/ioutil" ) func FileToStringLiteral(filepath string) (sl string, err error) { bs, err := ioutil.ReadFile(filepath) if err != nil { return } sl = hex.EncodeToString(bs) return }
I create a file, the content of which is hello world\n, the hex value returned by above method is 68656c6c6f20776f726c640a. Now we embed the file content in string literal as follows:
import ( "encoding/hex" ) const myFile = "68656c6c6f20776f726c640a" func ReadMyFile() ([]byte, error) { return hex.DecodeString(myFile) }
We can access the content of the file as follows:
b, err := ReadMyFile() if err != nil { panic(err) } print(string(b))
The printed output will be hello world\n.
Tested on: Ubuntu Linux 17.04, Go 1.8.1.
References:
[3]String literals - The Go Programming Language Specification - The Go Programming Language
[4]What is a string? - Strings, bytes, runes and characters in Go - The Go Blog
[6]hex - The Go Programming Language
[7]GitHub - siongui/goef: Embed file in your Go code
[8]static file embedding, modules, and non-developers : golang
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK