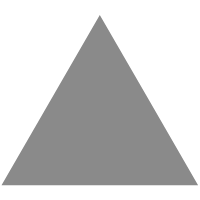
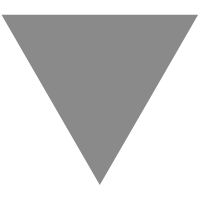
四种Javascript类型检测的方式
source link: https://www.fly63.com/article/detial/11296
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
一、typeof
主要用于判断基本数据类型 。使用方式:typeof(表达式)和typeof 变量名,第一种是对表达式做运算,第二种是对变量做运算。 typeof运算符的返回类型为字符串,值包括如下几种:
- 'undefined':未定义的变量或值
- 'boolean':布尔类型的变量或值
- 'string' :字符串类型的变量或值
- 'number':数字类型的变量或值
- 'object' :对象类型的变量或值,或者null(这个是js历史遗留问题,将null作为object类型处理)
- 'function' :函数类型的变量或值
示例如下:
console.log(typeof a); //'undefined'
console.log(typeof(true)); //'boolean'
console.log(typeof '123'); //'string'
console.log(typeof 123); //'number'
console.log(typeof NaN); //'number'
console.log(typeof null); //'object'
var obj = new String(); console.log(typeof(obj)); //'object'
var fn = function(){}; console.log(typeof(fn)); //'function'
console.log(typeof(class c{})); //'function'
typeof的不足之处:
- 不能区分对象、数组、正则,对它们操作都返回"object";(正则特殊一点后面说)
- Safar5,Chrome7之前的版本对正则对象返回 'function'
- 在IE6,7和8中,大多数的宿主对象是对象,而不是函数;如:typeof alert; //object
- 而在非ID浏览器或则IE9以上(包含IE9),typeof alert; //function
- 对于null,返回的是object.
typeof运算符用于判断对象的类型,但是对于一些创建的对象,它们都会返回'object',有时我们需要判断该实例是否为某个对象的实例,那么这个时候需要用到instanceof运算符。
二、instanceof
用于引用数据类型的判断。所有引用数据类型的值都是Object的实例。目的是判断一个对象在其原型链上是否存在构造函数的prototype属性。 用法:
variable instanceof constructor
示例如下:
// example
var arr = [];
由于:
1. arr.constructor === Array
2. arr.__proto__ === Array.prototype
3. arr.__poto__.proto__ === Object.prototype
所以, 以下都返回true
1. arr instanceof arr.constructor(Array)
2. arr instanceof arr.__proto__.constructor(Array)
3. arr instanceof arr.__proto__.__poto__.constructor(Object)
如果你了解原型链的话,你很快就会得出一些结论:
1. 所有对象 instanceof Object 都会返回 true
2. 所有函数 instanceof Function 都会返回 true
instanceof不仅能检测构造对象的构造器,还检测原型链。instanceof要求前面是个对象,后面是一个构造函数。而且返回的是布尔型的,不是true就是false。
三、Array.isArray()
Array.isArray()可以用于判断数组类型,支持的浏览器有IE9+、FireFox 4+、Safari 5+、Chrome; 兼容实现:
if (!Array.isArray) {
Array.isArray = function(arg) {
return Object.prototype.toString.call(arg) === '[object Array]';
};
}
示例如下:
// 1.
Array.isArray([1, 2, 3, 4]); // --> true
// 2.
var obj = {
a: 1,
b: 2
};
Array.isArray(obj); // --> false
// 3.
Array.isArray(new Array); // --> true
//4.
Array.isArray("Array"); // --> false
isArray是一个静态方法,使用Array对象(类)调用,而不是数组对象实例。其中Array.prototype 也是一个数组,Array.isArray 优于 instanceof。
四、Object.prototype.toString.call()
判断某个对象值属于哪种内置类型, 最靠谱的做法就是通过Object.prototype.toString方法。object.prototype.toString()输出的格式就是[object 对象数据类型]。
示例如下:
console.log(Object.prototype.toString.call("jerry"));//[object String]
console.log(Object.prototype.toString.call(12));//[object Number]
console.log(Object.prototype.toString.call(true));//[object Boolean]
console.log(Object.prototype.toString.call(undefined));//[object Undefined]
console.log(Object.prototype.toString.call(null));//[object Null]
console.log(Object.prototype.toString.call({name: "jerry"}));//[object Object]
console.log(Object.prototype.toString.call(function(){}));//[object Function]
console.log(Object.prototype.toString.call([]));//[object Array]
console.log(Object.prototype.toString.call(new Date));//[object Date]
console.log(Object.prototype.toString.call(/\d/));//[object RegExp]
function Person(){};
console.log(Object.prototype.toString.call(new Person));//[object Object]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK